react-codemirror

CodeMirror component for React. demo @uiwjs.github.io/react-codemirror/
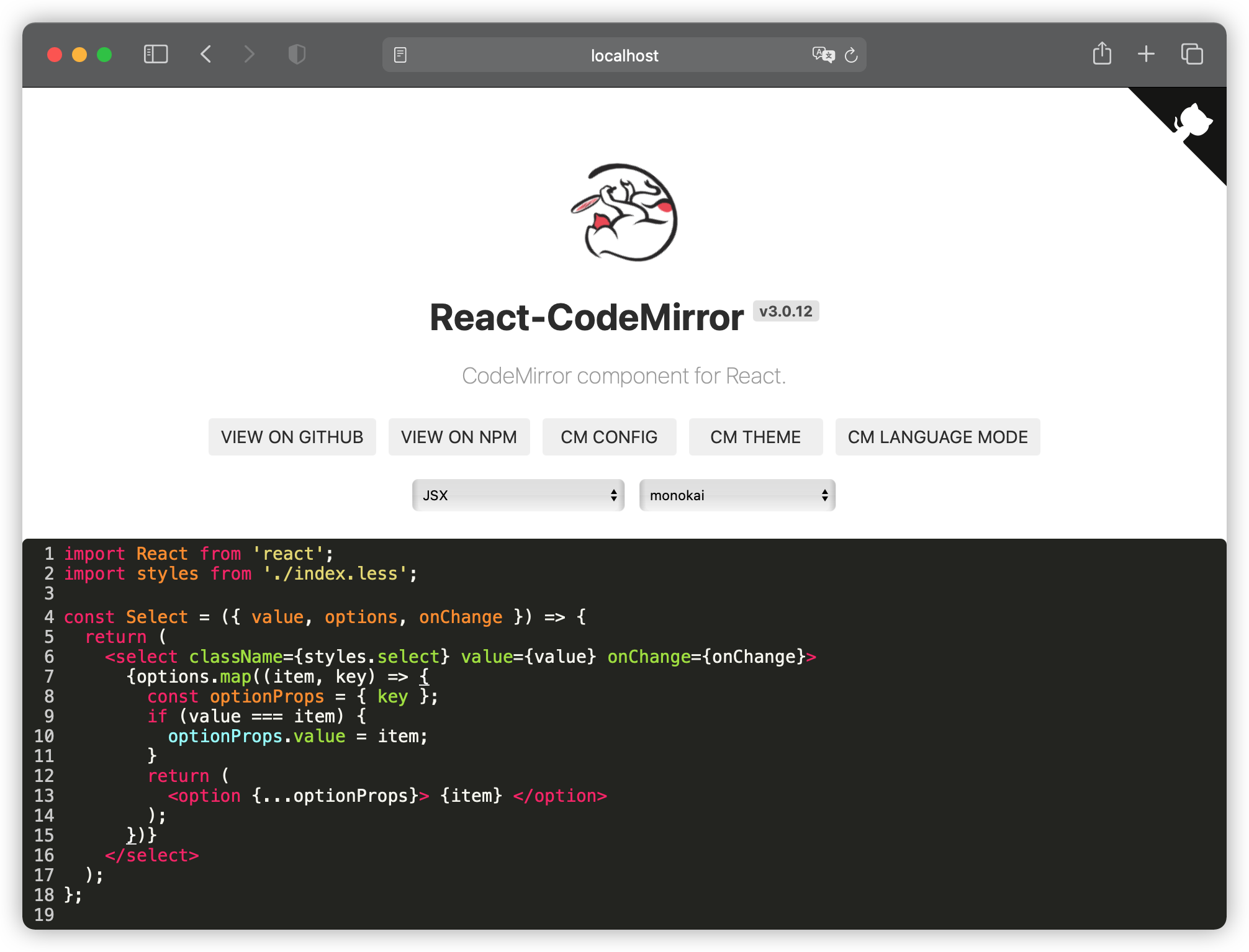
Features:
🌱 Automatically load mode
files based on configuration.
🚀 Quickly and easily configure the API.
🌎 There are better sample previews.
📚 Use Typescript to write and integrate @type declaration file.
Install
npm install @uiw/react-codemirror --save
Usage

import CodeMirror from '@uiw/react-codemirror';
import 'codemirror/keymap/sublime';
import 'codemirror/theme/monokai.css';
const code = 'const a = 0;';
<CodeMirror
value={code}
options={{
theme: 'monokai',
keyMap: 'sublime',
mode: 'jsx',
}}
/>
requiring codemirror resources. This is often done when specifying certain language modes and themes. Language resources do not need to be imported, just set the mode and they will be automatically lazy loaded.
import CodeMirror from '@uiw/react-codemirror';
import 'codemirror/addon/display/autorefresh';
import 'codemirror/addon/comment/comment';
import 'codemirror/addon/edit/matchbrackets';
import 'codemirror/keymap/sublime';
import 'codemirror/theme/monokai.css';
const code = 'const a = 0;';
<CodeMirror
value={code}
options={{
theme: 'monokai',
tabSize: 2,
keyMap: 'sublime',
mode: 'jsx',
}}
/>
If you do not want to lazy load the language resources, you can set the prop lazyLoadMode
to false
. You will need to load the language resources yourself in this case.
import CodeMirror from '@uiw/react-codemirror';
import 'codemirror/keymap/sublime';
import 'codemirror/theme/monokai.css';
import 'codemirror/mode/javascript/javascript';
const code = 'console.log("hello world!");';
<CodeMirror
value={code}
lazyLoadMode={false}
options={{
theme: 'monokai',
tabSize: 2,
keyMap: 'sublime',
mode: 'js',
}}
/>
Change Theme
import CodeMirror from '@uiw/react-codemirror';
import 'codemirror/addon/display/autorefresh';
import 'codemirror/addon/comment/comment';
import 'codemirror/addon/edit/matchbrackets';
import 'codemirror/keymap/sublime';
+ import 'codemirror/theme/monokai.css';
const code = 'const a = 0;';
<CodeMirror
value={code}
options={{
+ theme: 'monokai',
keyMap: 'sublime',
mode: 'jsx',
}}
/>
Setting Placeholder
import CodeMirror from '@uiw/react-codemirror';
import 'codemirror/theme/monokai.css';
import 'codemirror/mode/javascript/javascript';
import "codemirror/addon/display/placeholder";
const code = 'console.log("hello world!");';
<CodeMirror
value={code}
lazyLoadMode={false}
options={{
placeholder: 'Please enter the JavaScript code.',
theme: 'monokai',
tabSize: 2,
keyMap: 'sublime',
mode: 'js',
}}
/>
Properties
width
width of editor. Defaults to 100%
.height
height of editor. Defaults to 100%
.value
value of the auto created model in the editor.lazyLoadMode
should the mode by automatically lazy loaded. If this is set to false you will need to load the mode yourself. Defaults to true.options
refer to codemirror options.
Props Events
An editor instance fires the following codemirror events. The instance argument always refers to the editor itself.
<CodeMirror value="..." onChange={() => { }} />
import React from 'react';
import CodeMirror from 'codemirror';
export type DOMEvent = 'onMouseDown' | 'onDblClick' | 'onTouchStart' | 'onContextMenu' | 'onKeyDown' | 'onKeyPress'
| 'onKeyUp' | 'onCut' | 'onCopy' | 'onPaste' | 'onDragStart' | 'onDragEnter' | 'onDragOver' | 'onDragLeave' | 'onDrop';
export type IDOMEvent = {
[P in DOMEvent]?: (instance: CodeMirror.Editor, event: Event) => void;
}
export type Editor = CodeMirror.Editor;
export interface IReactCodemirror extends IDOMEvent {
onChange?: (instance: CodeMirror.Editor, change: CodeMirror.EditorChangeLinkedList[]) => void;
onChanges?: (instance: CodeMirror.Editor, change: CodeMirror.EditorChangeLinkedList[]) => void;
onBeforeChange?: (instance: CodeMirror.Editor, change: CodeMirror.EditorChangeCancellable) => void;
onCursorActivity?: (instance: CodeMirror.Editor) => void;
onBeforeSelectionChange?: (instance: CodeMirror.Editor, selection: { head: Position; anchor: Position; }) => void;
onViewportChange?: (instance: CodeMirror.Editor, from: number, to: number) => void;
onGutterClick?: (instance: CodeMirror.Editor, line: number, gutter: string, clickEvent: Event) => void;
onFocus?: (instance: CodeMirror.Editor) => void;
onBlur?: (instance: CodeMirror.Editor) => void;
onScroll?: (instance: CodeMirror.Editor) => void;
onUpdate?: (instance: CodeMirror.Editor) => void;
onRenderLine?: (instance: CodeMirror.Editor, line: CodeMirror.LineHandle, element: HTMLElement) => void;
onOverwriteToggle?: (instance: CodeMirror.Editor, overwrite: boolean) => void;
onCursorActivity?(instance: CodeMirror.Editor): void;
onDelete?(): void;
onBeforeCursorEnter?(): void;
onClear?(): void;
onHide?(): void;
onUnhide?(): void;
onRedraw?(): void;
}
Options
These are the supported codemirror options:
<CodeMirror value="..." options={{ ... }} />
interface EditorConfiguration {
value?: any;
mode?: any;
theme?: string;
indentUnit?: number;
smartIndent?: boolean;
tabSize?: number;
indentWithTabs?: boolean;
electricChars?: boolean;
rtlMoveVisually?: boolean;
keyMap?: string;
extraKeys?: any;
lineWrapping?: boolean;
lineNumbers?: boolean;
firstLineNumber?: number;
lineNumberFormatter?: (line: number) => string;
gutters?: string[];
foldGutter?: boolean;
fixedGutter?: boolean;
scrollbarStyle?: string;
readOnly?: any;
showCursorWhenSelecting?: boolean;
undoDepth?: number;
historyEventDelay?: number;
tabindex?: number;
autofocus?: boolean;
dragDrop?: boolean;
onDragEvent?: (instance: CodeMirror.Editor, event: Event) => boolean;
onKeyEvent?: (instance: CodeMirror.Editor, event: Event) => boolean;
cursorBlinkRate?: number;
cursorHeight?: number;
workTime?: number;
workDelay?: number;
pollInterval?: number
flattenSpans?: boolean;
maxHighlightLength?: number;
viewportMargin?: number;
lint?: boolean | LintOptions;
placeholder?: string;
}
Editor
hasFocus(): boolean;
findPosH(start: CodeMirror.Position, amount: number, unit: string, visually: boolean): { line: number; ch: number; hitSide?: boolean; };
findPosV(start: CodeMirror.Position, amount: number, unit: string): { line: number; ch: number; hitSide?: boolean; };
findWordAt(pos: CodeMirror.Position): CodeMirror.Range;
setOption(option: string, value: any): void;
getOption(option: string): any;
addKeyMap(map: any, bottom?: boolean): void;
removeKeyMap(map: any): void;
addOverlay(mode: any, options?: any): void;
removeOverlay(mode: any): void;
getDoc(): CodeMirror.Doc;
swapDoc(doc: CodeMirror.Doc): CodeMirror.Doc;
getValue(seperator?: string): string;
setValue(content: string): void;
setGutterMarker(line: any, gutterID: string, value: HTMLElement | null): CodeMirror.LineHandle;
clearGutter(gutterID: string): void;
addLineClass(line: any, where: string, _class_: string): CodeMirror.LineHandle;
removeLineClass(line: any, where: string, class_?: string): CodeMirror.LineHandle;
lineAtHeight(height: number, mode?: CoordsMode): number;
heightAtLine(line: any, mode?: CoordsMode, includeWidgets?: boolean): number;
lineInfo(line: any): {
line: any;
handle: any;
text: string;
gutterMarkers: any;
textClass: string;
bgClass: string;
wrapClass: string;
widgets: any;
};
addWidget(pos: CodeMirror.Position, node: HTMLElement, scrollIntoView: boolean): void;
addLineWidget(line: any, node: HTMLElement, options?: {
coverGutter: boolean;
noHScroll: boolean;
above: boolean;
showIfHidden: boolean;
}): CodeMirror.LineWidget;
setSize(width: any, height: any): void;
scrollTo(x?: number | null, y?: number | null): void;
getScrollInfo(): CodeMirror.ScrollInfo;
scrollIntoView(pos: CodeMirror.Position | null, margin?: number): void;
scrollIntoView(pos: { left: number; top: number; right: number; bottom: number; }, margin: number): void;
scrollIntoView(pos: { line: number, ch: number }, margin?: number): void;
scrollIntoView(pos: { from: CodeMirror.Position, to: CodeMirror.Position }, margin: number): void;
cursorCoords(where: boolean, mode?: CoordsMode): { left: number; top: number; bottom: number; };
cursorCoords(where: CodeMirror.Position, mode?: CoordsMode): { left: number; top: number; bottom: number; };
charCoords(pos: CodeMirror.Position, mode?: CoordsMode): { left: number; right: number; top: number; bottom: number; };
coordsChar(object: { left: number; top: number; }, mode?: CoordsMode): CodeMirror.Position;
defaultTextHeight(): number;
defaultCharWidth(): number;
getViewport(): { from: number; to: number };
refresh(): void;
getTokenAt(pos: CodeMirror.Position, precise?: boolean): Token;
getTokenTypeAt(pos: CodeMirror.Position): string;
getLineTokens(line: number, precise?: boolean): Token[];
getStateAfter(line?: number): any;
operation<T>(fn: ()=> T): T;
indentLine(line: number, dir?: string): void;
isReadOnly(): boolean;
execCommand(name: string): void;
focus(): void;
getInputField(): HTMLTextAreaElement;
getWrapperElement(): HTMLElement;
getScrollerElement(): HTMLElement;
getGutterElement(): HTMLElement;
on(eventName: string, handler: (instance: CodeMirror.Editor) => void ): void;
off(eventName: string, handler: (instance: CodeMirror.Editor) => void ): void;
on(eventName: 'change', handler: (instance: CodeMirror.Editor, change: CodeMirror.EditorChangeLinkedList) => void ): void;
off(eventName: 'change', handler: (instance: CodeMirror.Editor, change: CodeMirror.EditorChangeLinkedList) => void ): void;
on(eventName: 'changes', handler: (instance: CodeMirror.Editor, change: CodeMirror.EditorChangeLinkedList[]) => void ): void;
off(eventName: 'changes', handler: (instance: CodeMirror.Editor, change: CodeMirror.EditorChangeLinkedList[]) => void ): void;
on(eventName: 'beforeChange', handler: (instance: CodeMirror.Editor, change: CodeMirror.EditorChangeCancellable) => void ): void;
off(eventName: 'beforeChange', handler: (instance: CodeMirror.Editor, change: CodeMirror.EditorChangeCancellable) => void ): void;
on(eventName: 'cursorActivity', handler: (instance: CodeMirror.Editor) => void ): void;
off(eventName: 'cursorActivity', handler: (instance: CodeMirror.Editor) => void ): void;
on(eventName: 'beforeSelectionChange', handler: (instance: CodeMirror.Editor, selection: { head: CodeMirror.Position; anchor: CodeMirror.Position; }) => void ): void;
off(eventName: 'beforeSelectionChange', handler: (instance: CodeMirror.Editor, selection: { head: CodeMirror.Position; anchor: CodeMirror.Position; }) => void ): void;
on(eventName: 'viewportChange', handler: (instance: CodeMirror.Editor, from: number, to: number) => void ): void;
off(eventName: 'viewportChange', handler: (instance: CodeMirror.Editor, from: number, to: number) => void ): void;
on(eventName: 'gutterClick', handler: (instance: CodeMirror.Editor, line: number, gutter: string, clickEvent: Event) => void ): void;
off(eventName: 'gutterClick', handler: (instance: CodeMirror.Editor, line: number, gutter: string, clickEvent: Event) => void ): void;
on(eventName: 'focus', handler: (instance: CodeMirror.Editor) => void ): void;
off(eventName: 'focus', handler: (instance: CodeMirror.Editor) => void ): void;
on(eventName: 'blur', handler: (instance: CodeMirror.Editor) => void ): void;
off(eventName: 'blur', handler: (instance: CodeMirror.Editor) => void ): void;
on(eventName: 'scroll', handler: (instance: CodeMirror.Editor) => void ): void;
off(eventName: 'scroll', handler: (instance: CodeMirror.Editor) => void ): void;
on(eventName: 'update', handler: (instance: CodeMirror.Editor) => void ): void;
off(eventName: 'update', handler: (instance: CodeMirror.Editor) => void ): void;
on(eventName: 'renderLine', handler: (instance: CodeMirror.Editor, line: CodeMirror.LineHandle, element: HTMLElement) => void ): void;
off(eventName: 'renderLine', handler: (instance: CodeMirror.Editor, line: CodeMirror.LineHandle, element: HTMLElement) => void ): void;
on(eventName: DOMEvent, handler: (instance: CodeMirror.Editor, event: Event) => void ): void;
off(eventName: DOMEvent, handler: (instance: CodeMirror.Editor, event: Event) => void ): void;
state: any;
Related
Contributors
As always, thanks to our amazing contributors!
License
Licensed under the MIT License.