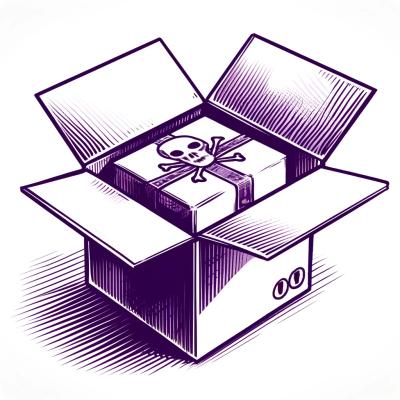
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
@vercel/sqs-consumer
Advanced tools
@vercel/sqs-consumer is a Node.js library that simplifies the process of consuming messages from Amazon SQS (Simple Queue Service). It provides a high-level API to handle message processing, error handling, and visibility timeout management, making it easier to build robust and scalable applications that interact with SQS.
Creating a Consumer
This feature allows you to create a consumer that listens to an SQS queue and processes messages. The `handleMessage` function is where you define the logic for handling each message.
const { Consumer } = require('@vercel/sqs-consumer');
const app = Consumer.create({
queueUrl: 'https://sqs.us-east-1.amazonaws.com/account-id/queue-name',
handleMessage: async (message) => {
console.log(message);
}
});
app.on('error', (err) => {
console.error(err.message);
});
app.start();
Error Handling
This feature demonstrates how to handle errors that occur during message processing. The `error` event is emitted for general errors, while the `processing_error` event is specific to errors that occur within the `handleMessage` function.
const { Consumer } = require('@vercel/sqs-consumer');
const app = Consumer.create({
queueUrl: 'https://sqs.us-east-1.amazonaws.com/account-id/queue-name',
handleMessage: async (message) => {
throw new Error('Something went wrong');
}
});
app.on('error', (err) => {
console.error('Error:', err.message);
});
app.on('processing_error', (err) => {
console.error('Processing error:', err.message);
});
app.start();
Batch Processing
This feature allows you to process messages in batches, which can be more efficient for high-throughput applications. The `handleMessageBatch` function is used to define the logic for handling a batch of messages.
const { Consumer } = require('@vercel/sqs-consumer');
const app = Consumer.create({
queueUrl: 'https://sqs.us-east-1.amazonaws.com/account-id/queue-name',
batchSize: 10,
handleMessageBatch: async (messages) => {
messages.forEach(message => console.log(message));
}
});
app.on('error', (err) => {
console.error(err.message);
});
app.start();
The `sqs-consumer` package is another popular library for consuming messages from Amazon SQS. It offers similar functionality to @vercel/sqs-consumer, including message processing, error handling, and batch processing. The main difference is that `sqs-consumer` is maintained by a different team and may have different release cycles and support policies.
The `aws-sdk` package is the official AWS SDK for JavaScript. While it provides low-level access to all AWS services, including SQS, it requires more boilerplate code to achieve the same functionality as @vercel/sqs-consumer. However, it offers greater flexibility and control over AWS service interactions.
Build SQS-based applications without the boilerplate. Just define an async function that handles the SQS message processing.
npm install sqs-consumer --save
const { Consumer } = require('sqs-consumer');
const app = Consumer.create({
queueUrl: 'https://sqs.eu-west-1.amazonaws.com/account-id/queue-name',
handleMessage: async (message) => {
// do some work with `message`
}
});
app.on('error', (err) => {
console.error(err.message);
});
app.on('processing_error', (err) => {
console.error(err.message);
});
app.start();
batchSize
option detailed below.keepAlive: true
.const { Consumer } = require('sqs-consumer');
const AWS = require('aws-sdk');
const app = Consumer.create({
queueUrl: 'https://sqs.eu-west-1.amazonaws.com/account-id/queue-name',
handleMessage: async (message) => {
// do some work with `message`
},
sqs: new AWS.SQS({
httpOptions: {
agent: new https.Agent({
keepAlive: true
})
}
})
});
app.on('error', (err) => {
console.error(err.message);
});
app.on('processing_error', (err) => {
console.error(err.message);
});
app.start();
By default the consumer will look for AWS credentials in the places specified by the AWS SDK. The simplest option is to export your credentials as environment variables:
export AWS_SECRET_ACCESS_KEY=...
export AWS_ACCESS_KEY_ID=...
If you need to specify your credentials manually, you can use a pre-configured instance of the AWS SQS client:
const { Consumer } = require('sqs-consumer');
const AWS = require('aws-sdk');
AWS.config.update({
region: 'eu-west-1',
accessKeyId: '...',
secretAccessKey: '...'
});
const app = Consumer.create({
queueUrl: 'https://sqs.eu-west-1.amazonaws.com/account-id/queue-name',
handleMessage: async (message) => {
// ...
},
sqs: new AWS.SQS()
});
app.on('error', (err) => {
console.error(err.message);
});
app.on('processing_error', (err) => {
console.error(err.message);
});
app.on('timeout_error', (err) => {
console.error(err.message);
});
app.start();
Consumer.create(options)
Creates a new SQS consumer.
queueUrl
- String - The SQS queue URLregion
- String - The AWS region (default eu-west-1
)handleMessage
- Function - An async
function (or function that returns a Promise
) to be called whenever a message is received. Receives an SQS message object as it's first argument.handleMessageBatch
- Function - An async
function (or function that returns a Promise
) to be called whenever a batch of messages is received. Similar to handleMessage
but will receive the list of messages, not each message individually. If both are set, handleMessageBatch
overrides handleMessage
.handleMessageTimeout
- Number - Time in ms to wait for handleMessage
to process a message before timing out. Emits timeout_error
on timeout. By default, if handleMessage
times out, the unprocessed message returns to the end of the queue.attributeNames
- Array - List of queue attributes to retrieve (i.e. ['All', 'ApproximateFirstReceiveTimestamp', 'ApproximateReceiveCount']
).messageAttributeNames
- Array - List of message attributes to retrieve (i.e. ['name', 'address']
).batchSize
- Number - The number of messages to request from SQS when polling (default 1
). This cannot be higher than the AWS limit of 10.visibilityTimeout
- Number - The duration (in seconds) that the received messages are hidden from subsequent retrieve requests after being retrieved by a ReceiveMessage request.heartbeatInterval
- Number - The interval (in seconds) between requests to extend the message visibility timeout. On each heartbeat the visibility is extended by adding visibilityTimeout
to the number of seconds since the start of the handler function. This value must less than visibilityTimeout
.terminateVisibilityTimeout
- Boolean - If true, sets the message visibility timeout to 0 after a processing_error
(defaults to false
).waitTimeSeconds
- Number - The duration (in seconds) for which the call will wait for a message to arrive in the queue before returning.authenticationErrorTimeout
- Number - The duration (in milliseconds) to wait before retrying after an authentication error (defaults to 10000
).pollingWaitTimeMs
- Number - The duration (in milliseconds) to wait before repolling the queue (defaults to 0
).sqs
- Object - An optional AWS SQS object to use if you need to configure the client manuallyconsumer.start()
Start polling the queue for messages.
consumer.stop()
Stop polling the queue for messages.
consumer.isRunning
Returns the current polling state of the consumer: true
if it is actively polling, false
if it is not.
Each consumer is an EventEmitter
and emits the following events:
Event | Params | Description |
---|---|---|
error | err , [message] | Fired when an error occurs interacting with the queue. If the error correlates to a message, that error is included in Params |
processing_error | err , message | Fired when an error occurs processing the message. |
timeout_error | err , message | Fired when handleMessageTimeout is supplied as an option and if handleMessage times out. |
message_received | message | Fired when a message is received. |
message_processed | message | Fired when a message is successfully processed and removed from the queue. |
response_processed | None | Fired after one batch of items (up to batchSize ) has been successfully processed. |
stopped | None | Fired when the consumer finally stops its work. |
empty | None | Fired when the queue is empty (All messages have been consumed). |
Consumer will receive and delete messages from the SQS queue. Ensure sqs:ReceiveMessage
and sqs:DeleteMessage
access is granted on the queue being consumed.
See contributing guidelines
FAQs
Build SQS-based Node applications without the boilerplate
The npm package @vercel/sqs-consumer receives a total of 307,174 weekly downloads. As such, @vercel/sqs-consumer popularity was classified as popular.
We found that @vercel/sqs-consumer demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 10 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.