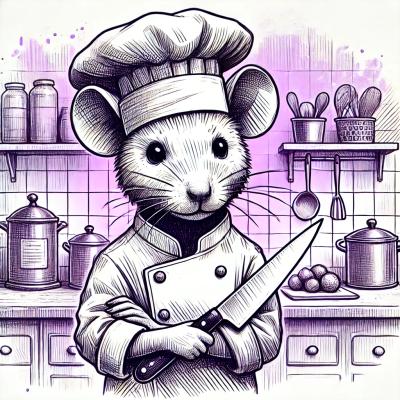
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@wdio/cucumber-framework
Advanced tools
A WebdriverIO plugin. Adapter for Cucumber.js testing framework.
@wdio/cucumber-framework is a plugin for WebdriverIO that allows you to use the Cucumber testing framework to write and execute feature files written in Gherkin syntax. This integration enables Behavior Driven Development (BDD) by allowing you to define application behavior in plain text and then automate the testing of that behavior.
Define Feature Files
You can define feature files using Gherkin syntax to describe the behavior of your application. These feature files are written in plain text and can be understood by all stakeholders.
Feature: User login
Scenario: Successful login with valid credentials
Given I am on the login page
When I enter valid credentials
Then I should be redirected to the dashboard
Step Definitions
Step definitions are used to map the steps in your feature files to actual code that performs the actions. This allows you to automate the testing of the behavior described in your feature files.
const { Given, When, Then } = require('@cucumber/cucumber');
Given('I am on the login page', async () => {
await browser.url('/login');
});
When('I enter valid credentials', async () => {
await $('#username').setValue('user');
await $('#password').setValue('password');
await $('button[type="submit"]').click();
});
Then('I should be redirected to the dashboard', async () => {
await expect(browser).toHaveUrl('/dashboard');
});
Hooks
Hooks allow you to run code before and after each scenario or step. This is useful for setting up preconditions or cleaning up after tests.
const { Before, After } = require('@cucumber/cucumber');
Before(async () => {
// Code to run before each scenario
await browser.reloadSession();
});
After(async () => {
// Code to run after each scenario
await browser.deleteCookies();
});
cypress-cucumber-preprocessor is a plugin for Cypress that allows you to use Cucumber with Cypress for BDD testing. It provides similar functionality to @wdio/cucumber-framework but is designed to work with the Cypress testing framework instead of WebdriverIO.
jest-cucumber is a library that allows you to use Cucumber with Jest for BDD testing. It provides similar functionality to @wdio/cucumber-framework but is designed to work with the Jest testing framework instead of WebdriverIO.
protractor-cucumber-framework is a plugin for Protractor that allows you to use Cucumber with Protractor for BDD testing. It provides similar functionality to @wdio/cucumber-framework but is designed to work with the Protractor testing framework instead of WebdriverIO.
A WebdriverIO plugin. Adapter for CucumberJS v5 testing framework.
The easiest way is to keep @wdio/cucumber-framework
as a devDependency in your package.json
, via:
npm install @wdio/cucumber-framework --save-dev
Instructions on how to install WebdriverIO
can be found here.
Following code shows the default wdio test runner configuration...
// wdio.conf.js
module.exports = {
// ...
framework: 'cucumber',
cucumberOpts: {
timeout: 10000
}
// ...
};
cucumberOpts
OptionsShow full backtrace for errors.
Type: Boolean
Default: false
Require modules prior to requiring any support files.
Type: String[]
Default: []
Example: ['@babel/register']
or [['@babel/register', { rootMode: 'upward', ignore: ['node_modules'] }]]
Abort the run on first failure.
Type: Boolean
Default: false
Only execute the scenarios with name matching the expression (repeatable).
Type: REGEXP[]
Default: []
Require files containing your step definitions before executing features. You can also specify a glob to your step definitions.
Type: String[]
Default: []
Example: [path.join(__dirname, 'step-definitions', 'my-steps.js')]
Paths to where your support code is, for ESM.
Type: String[]
Default: []
Example: [path.join(__dirname, 'step-definitions', 'my-steps.js')]
Fail if there are any undefined or pending steps
Type: Boolean
Default: false
Only execute the features or scenarios with tags matching the expression. Note that untagged
features will still spawn a Selenium session (see issue webdriverio/webdriverio#1247).
Please see the Cucumber documentation for more details.
If passing as a command-line argument, compound expressions may need to be enclosed in three sets of double quotes if WebdriverIO is invoked using npx
on Windows.
E.g.: npx wdio wdio.config.js --cucumberOpts.tags """@Smoke and not @Pending"""
Type: String
Default: ``
Timeout in milliseconds for step definitions.
Type: Number
Default: 30000
Specify the number of times to retry failing test cases.
Type: Number
Default: 0
Only retries the features or scenarios with tags matching the expression (repeatable). This option requires '--retry' to be specified.
Type: RegExp
Default language for your feature files
Type: String
Default: en
Run tests in defined / random order
Type: String
Default: defined
Name and output file path of formatter to use. WebdriverIO primarily supports only the Formatters that writes output to a file.
Type: string[]
Options to be provided to formatters
Type: object
Add cucumber tags to feature or scenario name
Type: Boolean
Default: false
Please note that this is a @wdio/cucumber-framework specific option and not recognized by cucumber-js itself
Treat undefined definitions as warnings.
Type: Boolean
Default: false
Please note that this is a @wdio/cucumber-framework specific option and not recognized by cucumber-js itself
Treat ambiguous definitions as errors.
Type: Boolean
Default: false
Please note that this is a @wdio/cucumber-framework specific option and not recognized by cucumber-js itself
Only execute the features or scenarios with tags matching the expression. Note that untagged
features will still spawn a Selenium session (see issue webdriverio/webdriverio#1247).
Please see the Cucumber documentation for more details.
If passing as a command-line argument, compound expressions may need to be enclosed in three sets of double quotes if WebdriverIO is invoked using npx
on Windows.
E.g.: npx wdio wdio.config.js --cucumberOpts.tagExpression """@Smoke and not @Pending"""
Type: String
Default: ``
Please note that this option would be deprecated in future. Use tags
config property instead
Specify the profile to use.
Type: string[]
Default: []
Kindly take note that only specific values (worldParameters, name, retryTagFilter) are supported within profiles, as cucumberOpts
takes precedence. Additionally, when using a profile, make sure that the mentioned values are not declared within cucumberOpts
.
Cucumber provides a feature to publish your test run reports to https://reports.cucumber.io/
, which can be controlled either by setting the publish
flag in cucumberOpts
or by configuring the CUCUMBER_PUBLISH_TOKEN
environment variable. However, when you use WebdriverIO
for test execution, there's a limitation with this approach. It updates the reports separately for each feature file, making it difficult to view a consolidated report.
To overcome this limitation, we've introduced a promise-based method called publishCucumberReport
within @wdio/cucumber-framework
. This method should be called in the onComplete
hook, which is the optimal place to invoke it. publishCucumberReport
requires the input of the report directory where cucumber message reports are stored.
You can generate cucumber message
reports by configuring the format
option in your cucumberOpts
. It's highly recommended to provide a dynamic file name within the cucumber message
format option to prevent overwriting reports and ensure that each test run is accurately recorded.
Before using this function, make sure to set the following environment variables:
Here's an example of the necessary configurations and code samples for implementation:
import { v4 as uuidv4 } from 'uuid'
import { publishCucumberReport } from '@wdio/cucumber-framework';
export const config = {
// ... Other Configuration Options
cucumberOpts: {
// ... Cucumber Options Configuration
format: [
['message', `./reports/${uuidv4()}.ndjson`],
['json', './reports/test-report.json']
]
},
async onComplete() {
await publishCucumberReport('./reports');
}
}
Please note that ./reports/
is the directory where cucumber message
reports will be stored.
For more information on WebdriverIO see the homepage.
FAQs
A WebdriverIO plugin. Adapter for Cucumber.js testing framework.
The npm package @wdio/cucumber-framework receives a total of 138,433 weekly downloads. As such, @wdio/cucumber-framework popularity was classified as popular.
We found that @wdio/cucumber-framework demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.