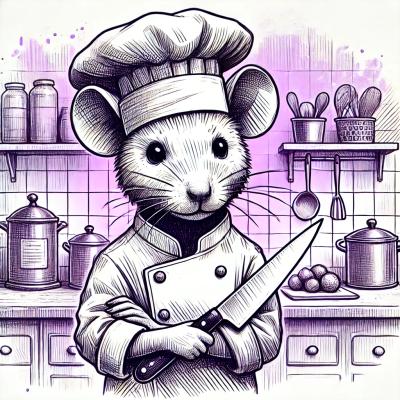
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@webdiscus/pug-loader
Advanced tools
The pug loader resolves paths and webpack aliases in a pug template and compiles it to HTML or into a template function.
Webpack loader for the Pug templates.
The pug loader resolves paths and webpack aliases for extends
/include
/require()
in a pug template and compiles it to HTML or to a template function.
NEW: The
pug-loader
is now the part of the pug-plugin. This plugin extracts HTML from thepug
files defined in the webpack entry and save them in the output directory. Now is possible definepug
files directly inwebpack entry
. See usage examples.
pugjs/pug-loader
pugjs/pug-loader
resolve.alias
and resolve.plugins
, works with and without the prefixes ~
@
tsconfig.json
using tsconfig-paths-webpack-plugin
Angular Component
CommonJS
and ES modules
in generated templates for loading them via require
or import
const tmpl = require('template.pug');
const html = tmpl({ key: "value" })
'render'
or query parameter ?pug-render
), e.g. using in javascript:
const html = require('template.pug?pug-render');
srcset
in img
tag:
img(srcset=`${require('./image1.jpeg')} 320w, ${require('./image2.jpeg')} 640w` src=require('./image.jpeg'))
output
<img srcset="/assets/image1.f78b30f4.jpeg 320w, /assets/image2.f78b30f4.jpeg 640w" src="/assets/image.f78b30f4.jpeg">
require()
for CommonJS and JSON files in pug templates, e.g.: data.json
[
{ "id": 1, "name": "abc" },
{ "id": 2, "name": "xyz" }
]
say-hello.js
module.exports = function (name) {
return `Hello ${name}!`;
}
pug template
- var sayHello = require('./say-hello')
h1 #{ sayHello('pug') }
- var myData = require('./data.json')
each item in myData
div #{item.id} #{item.name}
'html'
to handle HTML in additional loaders, e.g. in html-loader
data
, e.g.:
{
test: /\.pug$/,
loader: 'pug-loader',
options: {
method: 'render',
data: { "key": "value" }
}
},
or via query parameters:
const html = require('template.pug?pug-render&{"key":"value"}');
Why use this particular pug loader instead of the original one?
pugjs/pug-loader
is outdated and not maintained morepugjs/pug-loader
has error by npm install
see issue:
npm ERR! Found: pug@3.0.2 ... pug-loader@2.4.0" has incorrect peer dependency "pug@^2.0.0"
resolve.alias
also without the prefix ~
pugjs/pug-loader
Install
Webpack config
Options
Usage in Pug templates
Usage in JavaScript
Usage method compile
in JavaScript
Usage method render
in JavaScript
Usage method html
in JavaScript
Usage with Angular Component
Passing data into template
Usage embedded resources
More examples of usages
npm install @webdiscus/pug-loader --save-dev
For usage pug templates only in javascript is enough add to a webpack config:
{
module: {
rules: [
{
test: /\.pug$/,
loader: '@webdiscus/pug-loader',
}
]
}
}
Or you can define the resolveLoader.alias
to use the pug-loader
as default pug loader name:
{
resolveLoader: {
alias: {
'pug-loader': '@webdiscus/pug-loader'
}
},
// ...
module: {
rules: [
{
test: /\.pug$/,
loader: 'pug-loader',
}
]
}
}
For processing an embedded resource see usage embedded resources.
For rendering pug templates into static HTML is needed the pug-plugin.
The complete example of the webpack config file:
const path = require('path');
const PugPlugin = require('pug-plugin');
// The absolute path to the base directory of application.
const basePath = path.resolve(__dirname);
// Default pug-loader options.
const pugLoaderOptions = {
method: 'compile',
esModule: false,
};‚
module.exports = {
resolve: {
// aliases used in the code examples below
alias: {
Components: path.join(basePath, 'src/lib/components/ui/'),
Images: path.join(basePath, 'src/images/'),
Templates: path.join(basePath, 'src/templates/'),
}
},
resolveLoader: {
// alias for pug-loader
alias: {
'pug-loader': '@webdiscus/pug-loader'
}
},
output: {
path: path.join(basePath, 'public/'), // output path
publicPath: '/',
filename: '[name].js',
},
entry: {
// the script used a pug template
'script': './src/js/script.js',
// save HTML into output.path as index.html
'index': 'src/templates/index.pug',
},
plugins: [
// this plugin extract HTML from pug template defined in webpack entry
new PugPlugin(),
],
module: {
rules: [
{
test: /\.pug$/,
loader: 'pug-loader',
options: pugLoaderOptions
},
// processing an embedded resource, e.g. img(src=require('./image.jpeg'))
{
test: /\.(png|jpg|jpeg)/,
type: 'asset/resource'
generator: {
filename: 'assets/images/[name]-[hash][ext][query]',
},
}
]
}
};
See original description of options
basedir
Type: string
Default: /
The root directory of all absolute inclusion.
doctype
Type: string
Default: html
Specifies the type of document. See available doctypes.
self
Type: boolean
Default: false
Use the self
as namespace for the local variables in template. It will speed up the compilation, but for access to variable, e.g. myVariable
, you must write self.myVariable
.
globals
Type: Array<string>
Default: []
Add a list of global names to make accessible in templates.
filters
Type: object
Default: undefined
Hash table of custom filters.
Filters let to use other languages in Pug templates.
plugins
Type: Array<Object>
Default: []
Plugins allow to manipulate pug tags, template content in compile process.
How it works see in source of pug.
compileDebug
Type: boolean
Default: false
Includes the function source in the compiled template to improve error reporting.
pretty
Type: boolean
Default: false
This option is deprecated by pugjs and always is false
. Don't use it.
method
Type: string
Default: compile
Values:
compile
the pug template compiles into a template function and in JavaScript can be called with variables to render into HTML at runtime. ?pug-compile
. Can be used if the method is render
. render
the pug template renders into HTML at compile time and exported as a string.
All required resource will be processed by the webpack and separately included as added strings wrapped to a function. ?pug-render
. Can be used if the method is compile
or is not defined in options. html
the template renders into a pure HTML string at compile time. The method need an addition loader to handles the HTML. html-loader
see usageEmbedded resources such as
img(src=require('./image.jpeg'))
handles at compile time by the webpack using asset/resource.
esModule
Type: Boolean
Default: false
Enable/disable ES modules syntax in generated JS modules.
Values:
true
The pug-loader
generates JS modules with the ES modules syntax. import html from 'template.pug';
. false
defaults. The pug-loader
generates JS modules with the CommonJS modules syntax. const html = require('template.pug')
. false
for compatibility with the JS modules that is generated by the original pug-loader.Note: The option
esModule
is irrelevant for thehtml
method, because it returns a pure HTML string.
For generates smaller and faster JS code, it is recommended to use this options:
{ method: 'render', esModule: true }
data
Type: Object
Default: {}
The custom data will be passed in all pug templates, it can be useful by pass global data.
The example of simple file structure of an application under the path /srv/vhost/sample.com/
:
.
├--public/
├--lib/
| └--components/
| ├--ui/
| | ├--layout.pug
| | ├--mixins.pug
| | └--colors.json
| ...
├--src/
| ├--images/
| | ├--image.jpeg
| | ├--image1.jpeg
| | ├--image2.jpeg
| | └--image3.jpeg
| ├--js/
| | ├--script.js
| | └--data.json
| └--templates/
| ├--index.pug
| └--mixins.pug
| ├--widget.pug
└--webpack.config.js
The source Pug templates from src/templates/
after compilation are saved as HTML files in public/
.
File ./src/templates/index.pug
// 'Components' is the webpack alias for 'src/lib/components/ui/'
extends Components/layout.pug
include Components/mixins.pug
block content
- const colors = require('Components/colors.json')`
.color-container
+show-colors(colors)
File ./lib/components/ui/layout.pug
html
head
block head
body
block content
File ./lib/components/ui/mixins.pug
mixin show-colors(colors)
each color in colors
div(style=`background-color:${color.hex};`)= color.name
File ./lib/components/ui/colors.json
[
{
"name": "red",
"hex": "#f00"
},
{
"name": "green",
"hex": "#0f0"
},
{
"name": "blue",
"hex": "#00f"
}
]
In the sample above uses Webpack alias Components
instand of relative path ../../lib/components/ui/
.
This pug loader resolve all paths and aliases in Pug templates required from JavaScript.
For example, see the file structure of the application above, the pug template can be loaded in JavaScript via require().
The result of require() is a template function, where the argument is an object of variableName:value
, which are available in the pug template.
File ./src/js/script.js
:
// 'Templates' is the webpack alias for 'src/templates/'
const widgetTemplate = require('Templates/widget.pug');
// variables passed to the pug template
const locals = {
text: 'Hello World!',
colors: [
{
"name": "red",
"hex": "#f00"
},
{
"name": "green",
"hex": "#0f0"
},
{
"name": "blue",
"hex": "#00f"
}
]
}
// render template function with variables to HTML
const html = widgetTemplate(locals);
console.log(html);
File ./src/templates/widget.pug
:
include ~Templates/mixins
h2 Pug demo widget
//- the variables 'text' and `colors` are passed from 'script.js'
+widget(text, colors)
File ./src/templates/mixins.pug
:
mixin widget(text, colors)
.widget
p= text
each color in colors
div(style=`color:${color.hex};`)= color.name
The result of console.log(html)
:
<h2>Pug demo widget</h2>
<div class='widget'>
<p>Hello World!</p>
<div style="color:#f00">red</div>
<div style="color:#0f0">green</div>
<div style="color:#00f">blue</div>
</div>
See the simple web app example.
compile
, render
or html
in JavaScriptcompile
(default)In JavaScript the required template will be compiled into template function.
In webpack config add to module.rules
:
{
test: /\.pug$/,
loader: 'pug-loader',
options: {
method: 'compile' // default method `compile` can be omitted
}
}
In JavaScript, the result of require () is a template function. Call the template function with some variables to render it то HTML:
const tmpl = require('template.pug');
const html = tmpl({ key: 'value' }); // the HTML string
You can apply the method render
to single template using the query parameter ?pug-render
:
const html = require('template.pug?pug-render&{"key":"value"}'); // the HTML string
Note: if the query parameter
pug-render
is set, then will be used rendering for this template, independent of the loader optionmethod
. Variables passed in template with methodrender
will be used at compile time.
render
This method will render the pug into HTML at compile time.
In webpack config add to module.rules
:
{
test: /\.pug$/,
loader: 'pug-loader',
options: {
method: 'render'
}
}
In JavaScript the result of require() is an HTML string:
const html = require('template.pug'); // the HTML string
html
This method will render the pug to pure HTML and should be used with an additional loader to handle HTML.
In webpack config add to module.rules
:
{
test: /\.pug$/,
use: [
{
loader: 'html-loader',
options: {
esModule: false, // allow to use the require() for load a templqte in JavaScript
},
},
{
loader: 'pug-loader',
options: {
method: 'html',
},
},
],
}
In JavaScript the result of require() is an HTML string:
const html = require('template.pug'); // the HTML string
Webpack config:
{
test: /\.pug$/,
loader: 'pug-loader'
}
JavaScript:
// compile into template function, because loader option 'method' defaults is 'compile'
const tmpl = require('template.pug');
const html = tmpl({...});
// render the pug file into HTML, using the parameter 'pug-render'
const html2 = require('template2.pug?pug-render');
Webpack config:
{
test: /\.pug$/,
loader: 'pug-loader',
options: {
method: 'render'
}
}
JavaScript:
// render into HTML, because loader option 'method' is 'render'
const html = require('template.pug');
// compile into template function, using the parameter 'pug-compile'
const tmpl2 = require('template2.pug?pug-compile');
const html2 = tmpl2({...});
For usage pug-loader with Angular is needed to customize the webpack config.
Install packages:
npm i --saveDev @webdiscus/pug-loader pug-plugin-ng
in pug-loader can be used optional a plugin, e.g. pug-plugin-ng, to allow unquoted syntax of Angular: [(bananabox)]="val"
Create the file webpack.config.js
in root directory of angular project:
module.exports = {
resolveLoader: {
alias: {
'pug-loader': '@webdiscus/pug-loader',
},
},
module: {
rules: [
{
test: /\.pug$/,
loader: 'pug-loader',
options: {
method: 'render',
doctype: 'html',
plugins: [require('pug-plugin-ng')],
},
},
// processing an embedded resource by webpack
{
test: /\.(png|jpg|jpeg)/,
type: '`asset/resource`',
},
],
},
};
Bind the file webpack.config.js
in the Angular config angular.json
:
{
// ...
"projects": {
// ...
"architect": {
"build": {
// replace architect.build.builder with this value:
"builder": "@angular-builders/custom-webpack:browser",
// add the options:
"options": {
"aot": true,
"customWebpackConfig": {
"path": "./webpack.config.js" // the path to webpack.config.js
},
// ...
},
// ...
},
"serve": {
// replace architect.serve.builder with this value:
"builder": "@angular-builders/custom-webpack:dev-server",
"options": {
"browserTarget": "<app-name>:build"
},
// ...
},
// ...
}
}
},
}
In a component file, e.g. ./src/app/app.component.ts
set the templateUrl
with pug file:
import { Component } from '@angular/core';
// the variable `description` will be passed into pug template via resource query
const templateVars = '{"description": "Use pug template with Angular."}';
@Component({
selector: 'app-root',
styleUrls: ['./app.component.css'],
templateUrl: './app.component.pug?' + templateVars,
})
export class AppComponent {
title = 'ng-app';
}
Create a pug template, e.g. ./src/app/app.component.pug
:
h1 Hello Pug!
p Description: #{description}
See the source files of this example.
html-loader
Install the html-loader
:
npm i --saveDev html-loader
If your templates use the html-loader
, then modify the webpack.config.js
file:
module.exports = {
resolveLoader: {
alias: {
'pug-loader': '@webdiscus/pug-loader',
},
},
module: {
rules: [
{
test: /\.pug$/,
use: [
{
loader: 'html-loader',
options: {
esModule: false,
},
},
{
loader: 'pug-loader',
options: {
method: 'html', // the method render into HTML string and require additional loader `html-loader`
doctype: 'html',
plugins: [require('pug-plugin-ng')], // optional plugin
},
},
],
},
// processing an embedded resource by webpack
{
test: /\.(png|jpg|jpeg)/,
type: 'asset/resource',
},
],
},
};
See the source files of this example.
By default, the pug file is compiled as template function, into which can be passed an object with template variables.
const tmpl = require('template.pug');
const html = tmpl2({
key: 'value',
foo: 'bar',
});
But how pass variables in template which is rendered into HTML?
const html = require('template.pug');
Variables can be passed with query parameters, e.g.:
const html = require('template.pug?key=value&foo=bar');
or as a JSON object, e.g.:
const html = require('template.pug?{"key":"value","foo":"bar"}');
Using the method render
and JSON object:
const html = require('template.pug?pug-render&{"key":"value","foo":"bar"}');
Usage of query parameters is legal and official documented feature of webpack loader.
To pass variables global, in all templates at compile time use loader option data
:
{
test: /\.pug$/,
loader: 'pug-loader',
options: {
data: {
key: 'value',
foo: 'bar'
}
}
}
The variables will be passed in all templates independent of the method.
For processing image resources in templates with webpack use the require()
function:
img(src=require('./path/to/image.jpeg'))
To handles embedded resources in pug is needed the webpack module asset/resource
:
module.exports = {
module: {
rules: [
{
test: /\.(png|jpg|jpeg)/,
type: 'asset/resource'
},
// ...
]
},
};
More information about asset-modules see here.
The example of dynamically generating embedded resources in template:
- files = ['image1.jpeg', 'image2.jpeg', 'image3.jpeg']
each file in files
img(src=require(file))
The example of webpack alias used in the table below:
resolve: {
alias: {
Images: path.join(__dirname, 'src/images/'),
},
}
Code | @webdiscus/pug-loader | pugjs/pug-loader |
---|---|---|
img(src=require('image.jpeg')) | OK | fail |
img(src=require('./image.jpeg')) | OK | OK |
img(src=require('./images/image.jpeg')) | OK | OK |
img(src=require('../images/image.jpeg')) | OK | OK |
img(src=require('Images/image.jpeg')) | OK | OK |
- var file = 'image.jpeg' img(src=require('Images/' + file)) | OK | OK |
- var file = 'image.jpeg' img(src=require(`Images/${file}`)) | OK | OK |
- var file = 'image.jpeg' img(src=require(file)) | OK | fail |
- var file = 'image.jpeg' img(src=require(`${file}`)) | OK | fail |
- var file = 'image.jpeg' img(src=require('./' + file)) | OK | OK |
- var file = './image.jpeg' img(src=require(file)) | OK | fail |
- var file = './image.jpeg' img(src=require('' + file)) | OK | OK |
- var file = 'images/image.jpeg' img(src=require(file)) | OK | fail |
- var file = 'image.jpeg' img(src=require('./images/' + file)) | OK | OK |
- var file = 'image.jpeg' img(src=require(`./images/${file}`)) | OK | OK |
- var file = '../images/image.jpeg' img(src=require(file)) | OK | fail |
- var file = 'image.jpeg' img(src=require('../images/' + file)) | OK | OK |
- var file = 'image.jpeg' img(src=require(`../images/${file}`)) | OK | OK |
the pugjs/pug-loader can't resolve when used a mixin and require on same file: include mixins img(src=require('./image.jpeg')) | OK | fail |
Important: in examples used name of loader as
pug-loader
, because it is defined as alias at resolveLoader:
{ resolveLoader: { alias: { 'pug-loader': '@webdiscus/pug-loader' } }, }
npm run test
will run the unit and integration tests.
npm run test:coverage
will run the tests with coverage.
pug GitHub
pug API Reference
pug-plugin
FAQs
Pug loader renders Pug files into HTML or compiles them into a template function.
We found that @webdiscus/pug-loader demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.