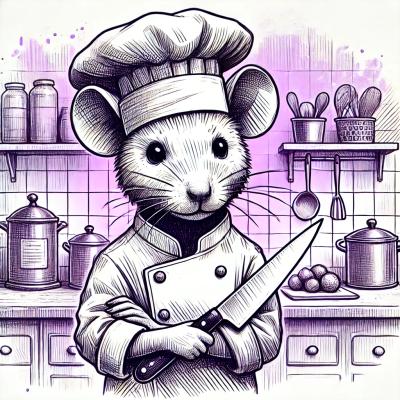
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
@websanova/vue-auth
Advanced tools
Vue.js token based authentication plugin. Supports simple token based and Json Web Tokens (JWT) authentication.
Vue.js token based authentication plugin. Supports simple token based and JSON Web Tokens (JWT) authentication.
Note this is the new name for the formerly named vue-jwt-auth
. Since it's likely this package will expand with potentially more authentication options.
Early support for Vue 2.0 is now available also but may still be a bit unstable due to many changes in the api. Please let me know of any issues you may find.
router
and http
options must ALWAYS set the driver to use. Please read the Change Log for more info.> sudo npm install @websanova/vue-auth
The router
and http
drivers MUST be set. The drivers are quite small so can be replaced or overridden as necessary.
Note that the version in the driver file should denote the version it is compatible with. So router.2x.
means it's for router 2.x.
Vue.http.options.root = 'https://api-demo.websanova.com/api/v1';
Vue.router = new VueRouter({
...
});
Vue.use(require('@websanova/vue-auth'), {
auth: require('@websanova/vue-auth/drivers/auth/bearer.js'),
http: require('@websanova/vue-auth/drivers/http/resource.1.x.js'),
router: require('@websanova/vue-auth/drivers/router/router.2.x.js'),
...
rolesVar: 'type'
...
});
> sudo npm install @websanova/vue-auth
Vue.http.options.root = 'https://api-demo.websanova.com/api/v1';
Vue.router = new VueRouter({
...
});
Vue.use(require('@websanova/vue-auth'), {
rolesVar: 'type'
});
NOTE: If you do not set your router as Vue.router = new VueRouter()
then you will need to feed the router
in directly through the options. Also true for http
.
var router = new VueRouter();
Vue.use(Auth, {
router: router,
http: http
});
To run the front end part of the demo just install and run. The demo runs on a publicly available server so just the front end needs run.
app.js
demo file to a different server for personal testing.> sudo npm install
> sudo npm run 1.x.demo
> sudo npm run 2.x.demo
Note: For Vue 2 demo there is a separate package.json. Unfortunately there is no really great way to run both at the same time.
If a different path is required it must be set in the demo/app.js
file.
To run the build:
> sudo webpack
A common gotcha is getting the $auth.user()
data properly. The plugin is designed to expect the user object to be in the data
parameter of the response.
Depending on what version of the vue-resource
plugin you are using the response data itself will come from either res.json()
or res.data
. Note that the data here is not the response data.
Sample response.
{
"status": "success",
"data": {
"id": 1,
"name": "Websanova",
...
}
}
The plugin has a function for parsing this user data when it receives it called _parseUserData
. The data
variable there is the res.json()
or res.data
object.
function _parseUserData(data) {
return data.data;
}
If a different format is needed simply override this function in the options.
Vue.use(require('vue-auth'), {
_parseUserData: function (data) {
return data.whatever;
}
});
Because there are so many different authentication schemes with tokens Vue Auth uses a simple model so that it can easily be extended for custom scenarios.
Each request and response that is made to the server is intercepted internally by the plugin.
request
driver method where it can be processed for delivery to the server.The current bearer
driver is quite simple and looks like this:
bearerAuth: {
request: function (req, token) {
this.options._setHeaders.call(this, req, {Authorization: 'Bearer ' + token});
},
response: function (res) {
var token = this.options._getHeaders.call(this, res).Authorization;
if (token) {
token = token.split('Bearer ');
return token[token.length > 1 ? 1 : 0];
}
}
},
The driver request
method receives the req
and token
arguments. From there we use an internal _setHeaders
method. However with the req
object pretty much anything can be assembled.
The driver response
method receives the res
argument and should parse out the token from the response and return a token which will get stored internally by the plugin.
To setup a custom driver we simply need to set the authType
option and the naming convention will follow.
Example 1: Token in sub object of body
Maybe the token is returned in a non standard way such as {data: {token: 'abcd1234'}}
.
authType: 'custom1',
custom1Auth: {
request: function (req, token) {
req.headers.set('SomeHeader', token);
},
response: function (res) {
return (res.data.data || {}).token;
}
}
Example 2: Token with multiple parts
Another common scenario may be a token with multiple data points that all need to be returned to the server.
authType: 'custom2',
custom2Auth: {
request: function (req, token) {
token = token.split(';');
this.options._setHeaders.call(this, req {
header1: token[0],
header2: token[1],
header3: token[2],
header4: token[3]
});
},
response: function (res) {
var headers = this.options._getHeaders.call(this, res);
if (headers.header1 && headers.header2 && headers.header3 && headers.header4) {
return headers.header1 + ';' + headers.header2 + ';' + headers.header3 + ';' + headers.header4;
}
}
}
The vue-auth
plugin works with the vue-router
plugin. Setting an auth
field in the router mapping section will set access to that route.
Vue 1.x
Vue.router.map({
'/admin': {
auth: 'admin',
component: require('./Admin')
},
'/manage': {
auth: ['admin', 'manager'],
component: require('./Manage')
},
'/account': {
auth: true,
component: require('./Account')
},
'/private': {
auth: [{"people": "administrator", "people": "superadmin"}],
component: require('./Account')
},
'/login': {
auth: false,
component: require('./Login')
},
'/contact': {
component: require('./Contact')
}
});
Vue 2.x
Vue.router = new VueRouter({
routes: [{
path: '/admin',
meta: {auth: 'admin'},
component: require('./Admin')
}, {
path: '/manage',
meta: {auth: ['admin', 'manager']},
component: require('./Manage')
}, {
path: '/account',
meta: {auth: true},
component: require('./Account')
}, {
path: '/private',
meta: {auth: [{"people": "administrator", "people": "superadmin"}]},
component: require('./Account')
}, {
path: '/login',
meta: {auth: false},
component: require('./Login')
}, {
path: '/contact',
component: require('./Contact')
}]
});
true
false
undefined
Array
String
roles
variable can be set in the options.Object
$auth.check({some: 'name'})
where the value can be a String
or Array
.These are all methods available in the vue app via $auth
.
success
and error
functions will receive proper context from currently called component.<div v-if="$auth.ready()">
<vue-router></vue-router>
</div>
<div v-if="!$auth.ready()">
Site loading...
</div>
<div>
{{ $auth.user().email }}
</div>
<a v-if="!$auth.check()" v-link="'/login'">login</a>
<a v-if="$auth.check('admin')">admin</a>
<a v-if="$auth.check(['admin', 'manager')]">manage</a>
<a v-if="$auth.check()" v-on:click="$auth.logout()">logout</a>
<a v-if="$auth.other()" v-on:click="logoutOther()">logout other</a>
this.$auth.disableOther();
this.$http.get('users'); // Will run as admin.
this.$auth.enableOther();
other
and default
will actually return anything.var token = this.$auth.token();
var token = this.$auth.token('other');
var token = this.$auth.token('default');
this.$auth.fetch({
params: {},
success: function () {},
error: function () {},
// etc...
});
expiredToken
option method.expiredToken
and token
to write custom refreshes.expiredToken
method and return boolean
.this.$auth.refresh({
params: {},
success: function () {},
error: function () {},
// etc...
});
autoLogin
parameter to attempt login directly after register.rememberMe
parameter when used in conjunction with autoLogin
equal to true
.redirect
parameter which is passed directly to router.this.$auth.register({
params: {},
success: function () {},
error: function () {},
autoLogin: true,
rememberMe: true,
redirect: {name: 'account'},
// etc...
});
rememberMe
parameter.redirect
parameter which is passed directly to router.fetchUser
param which allows disabling fetching user after login.this.$auth.login({
params: {},
success: function () {},
error: function () {},
rememberMe: true,
redirect: '/account',
fetchUser: true
// etc...
});
redirect
parameter which is passed directly to router.makeRequest
parameter which must be set to true
to send request to api. Otherwise the logout just happens locally by deleting tokens.this.$auth.logout({
makeRequest: true,
params: {},
success: function () {},
error: function () {},
redirect: '/login',
// etc...
});
redirect
parameter which is passed directly to router.this.$auth.loginOther({
params: {},
success: function () {},
error: function () {},
redirect: {name: 'account'},
// etc...
});
redirect
parameter which is passed directly to router.makeRequest
parameter same as logout
method.this.$auth.logoutOther({
makeRequest: true,
params: {},
success: function () {},
error: function () {},
redirect: {path: '/admin'},
// etc...
});
code
parameter which should be set to true
when the code is set.provider
parameter which hooks into data for third party.facebookData
, facebookOath2Data
. Check options section for more info.redirect
parameter which is passed directly to router.if (this.$route.query.code) {
this.$auth.oauth2({
code: true,
provider: 'facebook',
params: {
code: this.code
},
success: function(res) {},
error: function (res) {}
redirect: {path: '/account'},
// etc
});
}
else {
this.$auth.oauth2({
provider: 'facebook'
});
}
Pretty much all methods are overrideable now in case there any specific issues with a particular version of Vue.
[{request: 'Authorization', response: 'Authorization', authType: 'bearer', foundIn: 'header'}, {request: 'token', response: 'token', authType: 'bearer', foundIn: 'response'}]
'auth-token'
'roles'
{path: '/login'}
{path: '/403'}
{path: '/404'}
false
).{url: 'auth/register', method: 'POST', redirect: '/login'}
{url: 'auth/login', method: 'POST', redirect: '/', fetchUser: true}
fetchUser
to false
.{url: 'auth/logout', method: 'POST', redirect: '/', makeRequest: false}
makeRequest
is set to true.{url: 'auth/login', method: 'POST'}
{url: 'auth/user', method: 'GET'}
{url: 'auth/refresh', method: 'GET', atInit: true}
{url: 'auth/login-other', method: 'POST', redirect: '/'}
{url: 'auth/logout-other', method: 'POST', redirect: '/admin', makeRequest: false}
{url: 'auth/facebook', method: 'POST', redirect: '/'}
{url: 'auth/google', method: 'POST', redirect: '/'}
{url: 'https://www.facebook.com/v2.5/dialog/oauth', redirect: function () { return this.options.getUrl() + '/login/facebook'; }, clientId: '', scope: 'email'}
oauth2()
method or in the plugin options on init.{url: 'https://accounts.google.com/o/oauth2/auth', redirect: function () { return this.options.getUrl() + '/login/google'; }, clientId: '', scope: 'https://www.googleapis.com/auth/plus.me https://www.googleapis.com/auth/plus.login https://www.googleapis.com/auth/plus.profile.emails.read'}
_getUrl
_cookieDomain
rememberMe
option._parseUserData
_tokenExpired
false
when creating a custom solution._check
check
method.These are all function related directly to Vue that sort of acts like a driver. It makes it easier to deal with incompatibilities between Vue versions.
_init
_bind
_watch
_getHeaders
_setHeaders
_bindData
_interceptor
_beforeEach
_invalidToken
_routerReplace
_routerGo
_httpData
_http
Vue has been in such a volatile state, especially between versions and 1 and 2. There have been many breaking changes as well as removing vue-resource as the officially recommended package for Vue.
Because of all these changes and potential breaking changes the package has been changed to a much more driver centric model for "router", "resource" and "authentication" . Due to the nature of Vue and the current eco-system with Webpack, to avoid bloat this means the drivers will need to be passed in manually.
We will see some ugly require
code when including the plugin. But as a trade off it will reduce bloat and allow the plugin to much better support different versions of Vue as well as different "router", "resource" and "authentication" modules.
To ease confusion for this driver centric model the plugin has been bumped to a 2.x version. This will also keep it in line with the current Vue 2.x version.
router
, http
and auth
must be set now and will not auto bind (this is because webpack would pre load all drivers with dynamic variable).loginData.fetchUser
option to allow disabling of user fetch on login (which will also be disabled on refreshes).auth
matching via parent in Vue 2.request
and response
params instead of name
.auth
in meta
of route.vue-auth
from vue-jwt-auth
.@websanova/vue-auth
now.http
method. So it can be called with whatever parameters the method supports.success
and error
functions will contain proper context from Vue component./path
or {name: 'object'}
etc.register
call with the option to auto login on success.auth
parameter in routes and the check()
method now support objects for checking more complicated roles.other
now.disabledOther
and enableOther
.login
, oauth2
, etc all have their own default parameters now.google
and facebook
functions just use oauth2
method now.logout
method can now perform an api request if makdeRequest
is set to true.bearer
and basic
.other
or default
will return the appropriate token.FAQs
A simple light-weight authentication library for Vue.js
The npm package @websanova/vue-auth receives a total of 4,035 weekly downloads. As such, @websanova/vue-auth popularity was classified as popular.
We found that @websanova/vue-auth demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.