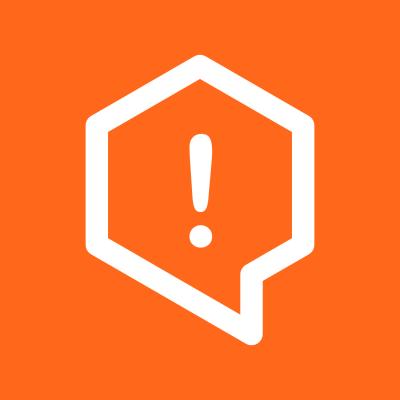
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
@wework/react-floormap
Advanced tools
React FloorMap Component (Powered by FloorMap-SDK) - By Location Based Experience
React FloorMap Component (Powered by FloorMap-SDK) - By Location Based Experience
yarn add @wework/react-floormap
Display Floormap by providing buildingId
and floorId
import React from 'react'
import { FloorMap } from `@wework/react-floormap`
class MyMap extends React.Component {
render() {
return (
<FloorMap
configuration={{
appId: configuration.appId,
appSecret: configuration.appSecret,
baseUrl: configuration.baseUrl,
spacemanToken: configuration.spacemanToken, // optional
mwAccessToken: configuration.mwAccessToken, // optional
}}
buildingId="b308b94c-bca6-4318-b906-1c148f7ca183"
floorId="54c2fe02-180f-11e7-9312-063c4950d72"
onClickSpace={(e) => {}}
onMouseOver={(e) => {}}
onMouseLeave={(e) => {}}
/>
)
}
}
<FloorMap
// ...
onClickSpace={(event) => {}}
onMouseOver={(event) => {}}
onMouseLeave={(event) => {}}
/>
onClickSpace
When user click on a spaceonMouseOver
When mouse over on a spaceonMouseLeave
When mouse leave on a spaceevent
object will be contains:
id
- ID of the spacepayload
- Event informationdata?
- If user interacts with space, the data field will presented
<FloorMap
// ...
onDataChange={(event) => {}}
onLoad={({ loading }) => {}}
onRender={({ building, floor, spaces, objects, options }) => {}}
onError={(e) => {
console.log(e.message)
console.log(e.data)
console.log(e.options)
}}
/>
onDataChange(event)
Gets triggered when data inside the map change via floorMap.updateData(id, data)
(An floorMap SDK instance, can via access via ref.current.getInstance()
)
onDataChange: function(space: Space)
onLoad(event)
Get call when the map start loading data
onLoad: function({ buildingId, floorId })
onRender(event)
Get call when the map finished rendering
onError(error)
Get call when an error occured during the map loading/rendering process
onError: function({ message, data, options })
message
- An error messagedata
- Data associated with the erroroptions
- Options parameters that was passed to render functiononSessionExpired(error)
Get call when session in the map is expired
<FloorMap
// ...
polarAngle={0} // Vetical rotation
azimuthAngle={90} // Horizontal rotation
fitContent={{ padding: 50 }}
/>
deskLayout
- Show/Hide desk layoutdeskInteractable
- Enable interaction on Desk and Chair<FloorMap
// ...
deskLayout={true}
deskInteractable={true}
/>
We provide simple way to make a selection and highlight styling to spaces by passing:
selectedSpaces
- Array of uuid of space (or space object) you want to style as selectionselectionColor
- Color to use style when space is in selection state (selectedSpaces
)highlightedSpaces
- Array of uuid of space (or space object) you want to style as highlight (we use this for hover effect)highlightColor
- Color to use style when space is in selection state (highlightedSpaces
)The different between selection
and highlight
is selection will have more priority then highlight, Thus space that got selection won't get highlight style even it is part of highlightedSpaces
<FloorMap
// ...
selectedSpaces={[uuid1, uuid2]}
highlightedSpaces={[uuid3]}
selectionColor="#acc3fe"
highlightColor="#ffffff"
/>
class MyMap extends React.Component {
state = { selected: []}
render() {
return (
<FloorMap
// ...
selectedSpaces={this.state.selected}
selectionColor="#acc3fe"
onClickSpace={({ data }) => {
if (data) {
this.setState({ selected: [data.uuid] })
} else {
// No data means click outside any space
this.setState({ selected: [] })
}
}}
/>
)
}
}
In case you want to take more control of styling or configure each space (eg. visible, interactable). You can use configureSpace
props to config each space in the Map.
<FloorMap
// ...
configureSpace={(space) => {
// Hide all DESK and CHIAR
if (space.type === 'object') {
return { visible: fales }
}
// All work type will be #f0f0ff
if (space.programType === 'WORK') {
return {
style: { color: '#f0f0ff' },
interactable: true,
}
}
// otherwise set color to #d0d0d0
// and set interactable to false
return {
style: { color: '#d0d0d0' }
interactable: false,
}
}}
/>
configureSpace
will get called everytime when to map need to style a space or you can force the map to re-configure space by call invalidate(space: Space[]?)
on FloorMap Instance
Use configureSpace to style space when user click
class MyMap extends React.Component {
floorMapRef = React.createRef()
render() {
return (
<FloorMap
// ...
ref={this.floorMapRef}
onClickSpace={({ data }) => {
this.setState({ currentSelection: data })
this.floorMapRef.invalidate()
}}
configureSpace={(space) => {
// Has currectSelection and uuid === space.uuid
if (
this.currentSelection
&& this.currentSelection.uuid === space.uuid
) {
return { style: { color: 'green' }}
} else {
// Otherwise
return { style: { color: '#f0f0f0' }}
}
}}
/>
)
}
}
To prevent configureSpace
get called for every spaces, you can pass array of spaces/uuid to configureSpace
to reload only specific spaces.
React Floormap is a wrapper on top of the FloorMap, simplify the API, and makes it easy to use on React application.
To use functions inside Floormap SDK such as adding an overlay, controlling the camera - You can access to the FloormapSDK instance by calling getInstance()
on the ref
class MyMap extends React.Component {
floorMapRef = React.createRef()
render() {
return (
<FloorMap
// ...
ref={this.floorMapRef}
onRender={(space) => {
const floorMap = this.floorMapRef.current.getInstance()
floormap.addOverlay(...)
}}
/>
)
}
}
Register extensions to the floormap, passing array of extension instances as a props to the component.
import { OccupancyExtension } from '@wework/floormap-extensions'
class MyMap extends React.Component {
occupancyExtension = new OccupancyExtension()
render() {
return (
<FloorMap
// ...
extensions={[this.occupancyExtension]}
/>
)
}
}
git clone git@github.com:WeConnect/react-floormap.git
cd react-floormap
yarn
yarn dev
yarn start:demo
FAQs
React FloorMap Component (Powered by FloorMap-SDK) - By Location Based Experience
We found that @wework/react-floormap demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 30 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.