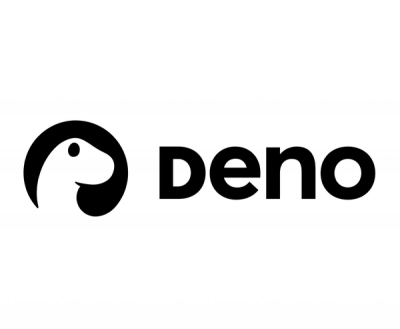
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
@zenginehq/frontend-bulk
Advanced tools
Module for working with large frontend Zengine API requests and avoiding API rate limits.
Module for working with frontend bulk Zengine API requests and avoiding API rate limits.
The methods included in this module are wrappers around the znData service, which provides a convenient means of communication with the Zengine API.
For clarification on the znData service please refer to https://zenginehq.github.io/developers/plugins/api/services/
npm install @zenginehq/frontend-bulk --save
plugin.controller('Controller', ['$scope', 'wgnBulk', function ($scope, wgnBulk) {
$scope.deleteRecords = function deleteRecords(records) {
var delay = 1500,
params = {};
params.formId = 12345;
wgnBulk.deleteAll('FormRecords', params, records, delay)
.then(function(response) {
//do something with the response
})
.catch(function(err) {
//handle the error
})
};
$scope.getAllRecords = function getAllRecords() {
var delay = 5000,
params = {
// Note that filters should be stringified
filter: JSON.stringify({ and: [{ prefix: '', attribute: 'isComplete', value: 1 }] })
};
params.formId = 12345;
wgnBulk.getAll('FormRecords', params, delay)
.then(function(response) {
//do something with the response
})
.catch(function(err) {
//handle the error
})
};
$scope.updateRecords = function updateRecords(updates) {
var delay = 10000,
params = {};
params.formId = 12345;
wgnBulk.saveAll('FormRecords', params, updates, delay)
.then(function(response) {
//do something with the response
})
.catch(function(err) {
//handle the error
});
};
}]);
FAQs
Module for working with large frontend Zengine API requests and avoiding API rate limits.
The npm package @zenginehq/frontend-bulk receives a total of 5 weekly downloads. As such, @zenginehq/frontend-bulk popularity was classified as not popular.
We found that @zenginehq/frontend-bulk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 6 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.