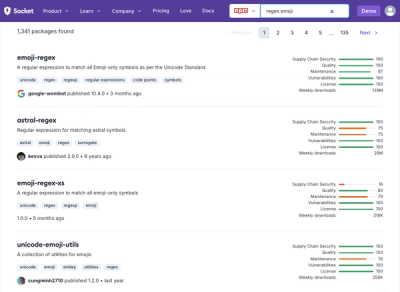
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
I always want to shoot myself in the head when looking at code like the following:
var url = "http://example.org/foo?bar=baz",
separator = url.indexOf('?') > -1 ? '&' : '?';
url += separator + encodeURIComponent("foo") + "=" + encodeURIComponent("bar");
I still can't believe javascript - the f**ing backbone-language of the web - doesn't offer an API for mutating URLs. Browsers (Firefox) don't expose the Location
object (the structure behind window.location). Yes, one could think of decomposed IDL attributes as a native URL management library. But it relies on the DOM element <a>, it's slow and doesn't offer any convenience at all.
How about a nice, clean and simple API for mutating URIs:
var url = new URI("http://example.org/foo?bar=baz");
url.addQuery("foo", "bar");
URI.js is here to help with that.
// mutating URLs
URI("http://example.org/foo.html?hello=world")
.username("rodneyrehm")
// -> http://rodneyrehm@example.org/foo.html?hello=world
.username("")
// -> http://example.org/foo.html?hello=world
.directory("bar")
// -> http://example.org/bar/foo.html?hello=world
.suffix("xml")
// -> http://example.org/bar/foo.xml?hello=world
.query("")
// -> http://example.org/bar/foo.xml
.tld("com")
// -> http://example.com/bar/foo.xml
.query({ foo: "bar", hello: ["world", "mars"] });
// -> http://example.com/bar/foo.xml?foo=bar&hello=world&hello=mars
// cleaning things up
URI("?&foo=bar&&foo=bar&foo=baz&")
.normalizeQuery();
// -> ?foo=bar&foo=baz
// working with relative paths
URI("/foo/bar/baz.html")
.relativeTo("/foo/bar/world.html");
// -> ./baz.html
URI("/foo/bar/baz.html")
.relativeTo("/foo/bar/sub/world.html")
// -> ../baz.html
.absoluteTo("/foo/bar/sub/world.html");
// -> /foo/bar/baz.html
// URI Templates
URI.expand("/foo/{dir}/{file}", {
dir: "bar",
file: "world.html"
});
// -> /foo/bar/world.html
See the About Page and API Docs for more stuff.
I guess you'll manage to use the build tool or follow the instructions below to combine and minify the various files into URI.min.js - and I'm fairly certain you know how to <script src=".../URI.min.js"></script>
that sucker, too.
Install with npm install URIjs
or add "URIjs"
to the dependencies in your package.json
.
// load URI.js
var URI = require('URIjs');
// load an optional module (e.g. URITemplate)
var URITemplate = require('URIjs/src/URITemplate');
URI("/foo/bar/baz.html")
.relativeTo("/foo/bar/sub/world.html")
// -> ../baz.html
Clone the URI.js repository or use a package manager to get URI.js into your project.
require.config({
paths: {
URIjs: 'where-you-put-uri.js/src'
}
});
require(['URIjs/URI'], function(URI) {
console.log("URI.js and dependencies: ", URI("//amazon.co.uk").is('sld') ? 'loaded' : 'failed');
});
require(['URIjs/URITemplate'], function(URITemplate) {
console.log("URITemplate.js and dependencies: ", URITemplate._cache ? 'loaded' : 'failed');
});
See the build tool or use Google Closure Compiler:
// ==ClosureCompiler==
// @compilation_level SIMPLE_OPTIMIZATIONS
// @output_file_name URI.min.js
// @code_url http://medialize.github.com/URI.js/src/IPv6.js
// @code_url http://medialize.github.com/URI.js/src/punycode.js
// @code_url http://medialize.github.com/URI.js/src/SecondLevelDomains.js
// @code_url http://medialize.github.com/URI.js/src/URI.js
// @code_url http://medialize.github.com/URI.js/src/URITemplate.js
// ==/ClosureCompiler==
Documents specifying how URLs work:
Informal stuff
How other environments do things
If you don't like URI.js, you may like one of these:
URI.js is published under the MIT license and GPL v3.
hasQuery()
- (Issue #71)jquery.URI.js
) - (Issue #69)URI(location)
.setQuery()
- (Issue #64).query()
.fragmentPrefix()
to configure prefix of fragmentURI and fragmentQuery extensions - (Issue #55).toString()
, .valueOf()
and .href()
- (Issue #56).relativeTo()
for descendants - (Issue #57).resource()
as compound of [path, query, fragment].duplicateQueryParameters()
to control if key=value
duplicates have to be preserved or reduced (Issue #51).addQuery("empty")
to properly add ?empty
- (Issue #46)http://username:pass:word@hostname
file://C:/WINDOWS/foo.txt
URI(location)
to properly parse the URL - (Issue #52)Note: QUnit seems to be having some difficulties on IE8. While the jQuery-plugin tests fail, the plugin itself works. We're still trying to figure out what's making QUnit "lose its config state".
/wiki/Help:IPA
- (Issue #49)strictEncodeURIComponent()
to properly encode *
to %2A
img.href
being available - (Issue #48).tld()
- foot.se
would detect t.se
- (Issue #42).absoluteTo()
to comply with RFC 3986 Section 5.2.2 - (Issue #41)location
not being available in non-browser environments like node.js (Issue #45 grimen).segment()
's append operation - (Issue #39)base
- (Issue #33 LarryBattle).segment()
accessor - (Issue #34)URI.encode()
to strict URI encoding according to RFC3986URI.encodeReserved()
to exclude reserved characters (according to RFC3986) from being encodedURITemplate()
.absoluteTo()
to join two relative paths properly - (Issue #29).clone()
to copy an URI instance.directory()
now returns empty string if there is no directory.absoluteTo()
to join two relative paths properly - (Issue #29)javascript:
, mailto:
, ...) support.scheme()
as alias of .protocol()
.userinfo()
to comply with terminology of RFC 3986src/jquery.URI.js
URI.iso8859()
and URI.unicode()
to switch base charsets - (Issue #10, mortenn).iso8859()
and .unicode()
to convert an URI's escape encoding.hostname()
.subdomain()
convenience accessorURI("http://example.org").query(true)
- (Issue #6).equals()
for URL comparison.pathname()
, .directory()
, .filename()
and .suffix()
according to RFC 3986 3.3+
according to application/x-www-form-urlencodedURI.buildQuery()
to build duplicate key=value combinationsURI(string, string)
constructor to conform with the specification.readable()
for humanly readable representation of encoded URIsURI.withinString()
.normalizeProtocol()
to lowercase protocols.normalizeHostname()
to lowercase hostnames{foo: null}
Algorithm for collecting URL parameters{foo: null, bar: ""}
to "?foo&bar=" Algorithm for serializing URL parameters1.10.0 (March 16th 2013) ###
hasQuery()
- (Issue #71)jquery.URI.js
) - (Issue #69)FAQs
package renamed to , please update dependencies!
The npm package URIjs receives a total of 46,283 weekly downloads. As such, URIjs popularity was classified as popular.
We found that URIjs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.