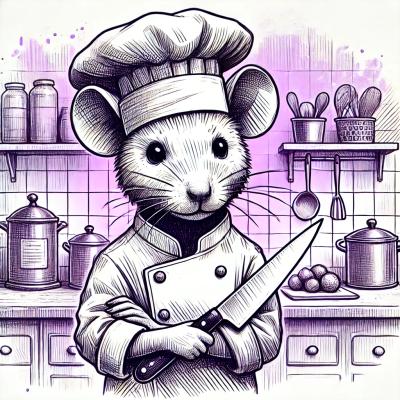
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
abstract-state-router
Advanced tools
The basics of a client-side state router ala the AngularJS ui-router, but without any DOM interactions
ui-router is fantastic, and I would use it in all of my projects if it wasn't tied to AngularJS.
But I don't want to use AngularJS - I want to use [my favorite templating/dom manipulation libraries here].
Thus, this library! Written to work with browserify, it lets you create nested "states" that correspond to different parts of the url path.
For example: if your "user profile" state contains "contact settings" and "profile image" sub-states, browsing from /profile/contact to /profile/image will change the content to display the "profile image" state without touching any of the DOM elements in the user profile page template.
To see an example app implemented with a couple of different browser rendering libraries, click here to visit the state-router-example on Github Pages.
var createStateRouter = require('abstract-state-router')
var stateRouter = createStateRouter(makeRenderer, rootElement, router)
The makeRenderer
should be a function that returns an object with four properties: render, destroy, getChildElement, and reset. Still needs to be documented, see test/support/renderer-mock.js for an implementation.
The rootElement
is the element where the first-generation states will be created.
router
defaults to an instance of a hash brown router. It's an optional argument for the purpose of passing in a mock for unit tests.
The addState function takes a single object of options. All of them are optional, unless stated otherwise.
name
is parsed in the same way as ui-router's dot notation, so 'contacts.list' is a child state of 'contacts'. Required.
route
is an express-style url string that is parsed with a fork of path-to-regexp. If the state is a child state, this route string will be concatenated to the route string of its parent (e.g. if 'contacts' state has route ':user/contacts' and 'contacts.list' has a route of '/list', you could visit the child state by browsing to '/tehshrike/contacts/list').
defaultChild
is a string (or a function that returns a string) of the default child's name. If you attempt to go directly to a state that has a default child, you will be directed to the default child. (E.g. if 'contacts' has the defaultChild 'list', then doing state.go('contacts')
will actually do state.go('contacts.list')
.)
data
is an object that can hold whatever you want - it will be passed in to the resolve and activate functions.
template
is a template string/object/whatever to be interpreted by the render function. Required.
resolve
is a function called when the selected state begins to be transitioned to, allowing you to accomplish the same objective as you would with ui-router's resolve.
activate
is a function called when the state is made active - the equivalent of the AngularJS controller to the ui-router.
querystringParameters
is an array of query string parameters that will be watched by this state.
The first argument is the data object you passed to the addState call. The second argument is an object containing the parameters that were parsed out of the route params and the query string.
If you call callback(err, content)
with a truthy err value, the state change will be cancelled and the previous state will remain active.
If you call callback.redirect(stateName, params)
, the state router will begin transitioning to that state instead. The current destination will never become active, and will not show up in the browser history.
The activate function is called when the state becomes active. It is passed an event emitter named context
with four properties:
domApi
: the DOM API returned by the rendererdata
: the data object given to the addState callparameters
: the route/querystring parameterscontent
: the object passed into the resolveFunction's callbackstateRouter.addState({
name: 'app',
data: {},
route: '/app',
template: '',
defaultChild: 'tab1',
resolve: function(data, parameters, cb) {
// Sync or asnyc stuff; just call the callback when you're done
isLoggedIn(function(err, isLoggedIn) {
cb(err, isLoggedIn)
})
}, activate: function(context) {
// Normally, you would set data in your favorite view library
var isLoggedIn = context.content
var ele = document.getElementById('status')
ele.innerText = isLoggedIn ? 'Logged In!' : 'Logged Out!'
}
})
stateRouter.addState({
name: 'app.tab1',
data: {},
route: '/tab_1',
template: '',
resolve: function(data, parameters, cb) {
getTab1Data(cb)
}, activate: function(context) {
document.getElementById('tab').innerText = context.content
}
})
stateRouter.addState({
name: 'app.tab2',
data: {},
route: '/tab_2',
template: '',
resolve: function(data, parameters, cb) {
getTab2Data(cb)
}, activate: function(context) {
document.getElementById('tab').innerText = context.content
}
})
Browses to the given state, with the current parameters. Changes the url to match.
The options object currently supports just one option "replace" - if it is truthy, the current state is replaced in the url history.
If a state change is triggered during a state transition, and the DOM hasn't been manipulated yet, then the current state change is discarded, and the new one replaces it. Otherwise, it is queued and applied once the current state change is done.
stateRouter.go('app')
// This actually redirects to app.tab1, because the app state has the default child: 'tab1'
You'll want to call this once you've added all your initial states. It causes the current path to be evaluated, and will activate the current state. If the current path doesn't match the route of any available states, the browser gets sent to the fallback route provided.
stateRouter.evaluateCurrentRoute('app.tab2')
FAQs
Like ui-router, but without all the Angular. The best way to structure a single-page webapp.
The npm package abstract-state-router receives a total of 219 weekly downloads. As such, abstract-state-router popularity was classified as not popular.
We found that abstract-state-router demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.