What is adaptivecards?
The adaptivecards npm package allows developers to create and render adaptive cards, which are a way to present and collect information in a consistent and visually appealing manner across different platforms. Adaptive cards are used in various applications like chatbots, messaging apps, and other interactive user interfaces.
What are adaptivecards's main functionalities?
Creating Adaptive Cards
This feature allows you to create an adaptive card with a simple text block and a submit button. The JSON structure defines the card's content and actions.
{
"type": "AdaptiveCard",
"version": "1.0",
"body": [
{
"type": "TextBlock",
"text": "Hello World!"
}
],
"actions": [
{
"type": "Action.Submit",
"title": "Submit"
}
]
}
Rendering Adaptive Cards
This feature allows you to render an adaptive card in a web application. The code parses the card JSON and appends the rendered card to the document body.
const AdaptiveCards = require('adaptivecards');
const adaptiveCard = new AdaptiveCards.AdaptiveCard();
adaptiveCard.parse({
"type": "AdaptiveCard",
"version": "1.0",
"body": [
{
"type": "TextBlock",
"text": "Hello World!"
}
],
"actions": [
{
"type": "Action.Submit",
"title": "Submit"
}
]
});
const renderedCard = adaptiveCard.render();
document.body.appendChild(renderedCard);
Handling User Input
This feature allows you to handle user input and actions within the adaptive card. The code sets up an event listener for when an action is executed, logging the action to the console.
adaptiveCard.onExecuteAction = function(action) {
console.log('Action executed:', action);
};
Other packages similar to adaptivecards
botframework-webchat
The botframework-webchat package provides a web-based chat interface for interacting with bots built using the Microsoft Bot Framework. It supports adaptive cards but is more focused on providing a complete chat experience, including message rendering, user input handling, and bot communication.
react-native-gifted-chat
The react-native-gifted-chat package is a popular React Native library for building chat interfaces. While it does not natively support adaptive cards, it offers a highly customizable chat UI and can be extended to support various message types and interactions.
botpress
Botpress is an open-source platform for building, deploying, and managing chatbots. It includes support for rich message types, including adaptive cards, and provides a comprehensive set of tools for bot development, including a visual flow editor and analytics.
Adaptive Cards
Adaptive Cards are a new way for developers to exchange card content in a common and consistent way.
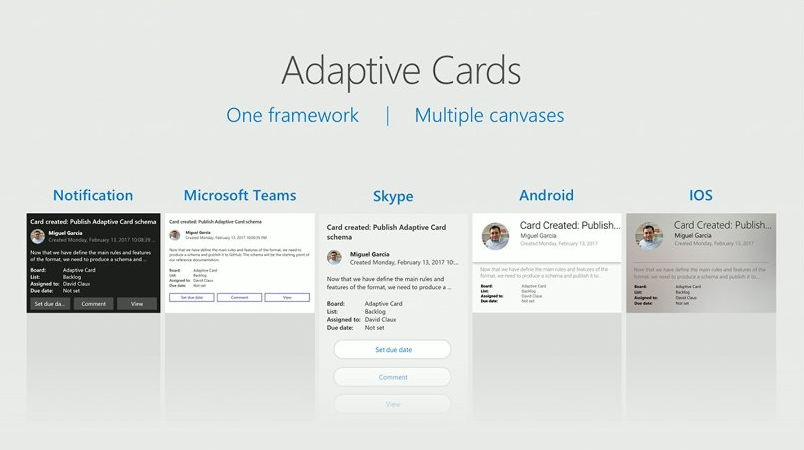
Expressive cards, open framework, multiple platforms
Break outside the box of templated cards. Adaptive Cards let you describe your content as you see fit and deliver it beautifully wherever your customers are.
The simple open card format enables an ecosystem of shared tooling, seamless integration between producers and consumers, and native cross-platform performance on any device.
Get Started
Check out the full documentation for more
Breaking changes
Please be aware of the following breaking changes in particular versions.
In version | Change description |
---|
1.2 | The default value of an Input.Time no longer accepts seconds. 08:25:32 will now be treated as an invalid value and ignored; it should be replaced with 08:25. This behavior is consistent with other Adaptive Card renderers. |
| The ICardObject interface has been REMOVED, replaced with the CardObject class that both CardElement and Action extend. This change should have little to no impact on any application. |
| The CardElement.validate() and Action.validate() methods have been REMOVED, replaced with CardObject.validateProperties() and CardObject.internalValidateProperties(context: ValidationContext) . Custom elements and actions now must override internalValidateProperties and add validation failures as appropriate to the context object passed as a parameter using its addFailure method. Be sure to always call super.internalValidateProperties(context) in your override. |
1.1 | Due to a security concern, the processMarkdown event handler has been REMOVED. Setting it will throw an exception that will halt your code. Please change your code to set the onProcessMarkdown(text, result) event handler instead (see example below.) |
1.0 | The standalone renderCard() helper function was removed as it was redundant with the class methods. Please use adaptiveCard.render() as described below. |
Install
Node
npm install adaptivecards --save
CDN
The unpkg.com CDN makes it easy to load the script in an browser.
The latest release will keep you up to date with features and fixes, but may have breaking changes over time. For maximum stability you should use a specific version.
adaptivecards.js
- non-minified, useful for devadaptivecards.min.js
- minified version, best for production
<script src="https://unpkg.com/adaptivecards/dist/adaptivecards.min.js"></script>
<script src="https://unpkg.com/adaptivecards@1.1.1/dist/adaptivecards.min.js"></script>
Usage
Import the module
import * as AdaptiveCards from "adaptivecards";
var AdaptiveCards = require("adaptivecards");
Render a card
var card = {
"type": "AdaptiveCard",
"version": "1.0",
"body": [
{
"type": "Image",
"url": "http://adaptivecards.io/content/adaptive-card-50.png"
},
{
"type": "TextBlock",
"text": "Hello **Adaptive Cards!**"
}
],
"actions": [
{
"type": "Action.OpenUrl",
"title": "Learn more",
"url": "http://adaptivecards.io"
},
{
"type": "Action.OpenUrl",
"title": "GitHub",
"url": "http://github.com/Microsoft/AdaptiveCards"
}
]
};
var adaptiveCard = new AdaptiveCards.AdaptiveCard();
adaptiveCard.hostConfig = new AdaptiveCards.HostConfig({
fontFamily: "Segoe UI, Helvetica Neue, sans-serif"
});
adaptiveCard.onExecuteAction = function(action) { alert("Ow!"); }
adaptiveCard.parse(card);
var renderedCard = adaptiveCard.render();
document.body.appendChild(renderedCard);
Supporting Markdown
Markdown is a standard feature of Adaptive Cards, but to give users flexibility we don't bundle a particular implementation with the library.
Option 1: Markdown-It
The easiest way to get markdown support is by adding markdown-it to your document.
<script type="text/javascript" src="https://unpkg.com/markdown-it/dist/markdown-it.min.js"></script>
Option 2: Any other 3rd party library
If you want to use another library or handle markdown yourself, use the AdaptiveCards.onProcessMarkdown
event.
IMPORTANT SECURITY NOTE: When you process Markdown (yourself or using a library) you are responsible for making sure the output HTML is safe.
For example, you must remove <script>
or other HTML elements that could be injected onto the page.
- Failure to do so will make your application susceptible to script injection attacks.
- Most Markdown libraries, such as
Markdown-It
, offer HTML sanitation.
AdaptiveCards.onProcessMarkdown = function(text, result) {
result.outputHtml = <your Markdown processing logic>;
result.didProcess = true;
}
Make sure to set result.didProcess
to true
, otherwise the library will consider the input text as not processed and will be treated as plain text.
Webpack
If you don't want adaptivecards in your bundle, make sure the script is loaded and add the following the your webpack.config.json
module.exports = {
...
externals: [
{ adaptivecards: { var: "AdaptiveCards" } }
]
};