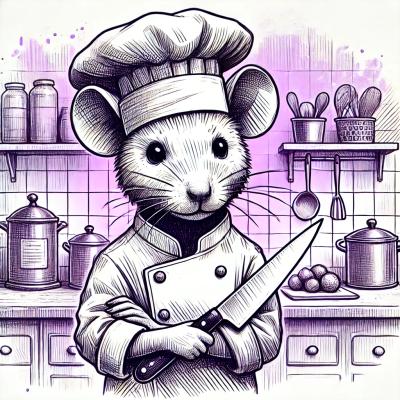
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
angular-oauth2-oidc
Advanced tools
The angular-oauth2-oidc package is an Angular library that provides support for OAuth 2 and OpenID Connect (OIDC) authentication. It allows developers to easily integrate secure authentication and authorization into their Angular applications.
OAuth2 and OIDC Authentication
This feature allows you to configure and initiate OAuth2 and OIDC authentication in your Angular application. The code sample demonstrates how to set up the OAuthService with the necessary configuration and initiate the login process.
import { OAuthService, AuthConfig } from 'angular-oauth2-oidc';
const authConfig: AuthConfig = {
issuer: 'https://your-identity-server',
redirectUri: window.location.origin + '/index.html',
clientId: 'your-client-id',
scope: 'openid profile email',
responseType: 'code'
};
oauthService.configure(authConfig);
oauthService.loadDiscoveryDocumentAndTryLogin();
Token Management
This feature provides methods to manage tokens, including retrieving access and ID tokens, and refreshing tokens. The code sample shows how to get the access token, ID token, and refresh the token using the OAuthService.
import { OAuthService } from 'angular-oauth2-oidc';
// Get access token
const accessToken = oauthService.getAccessToken();
// Get ID token
const idToken = oauthService.getIdToken();
// Refresh token
oauthService.refreshToken();
User Profile Information
This feature allows you to retrieve user profile information from the ID token. The code sample demonstrates how to get the identity claims and log them to the console.
import { OAuthService } from 'angular-oauth2-oidc';
// Get user profile information
const claims = oauthService.getIdentityClaims();
if (claims) {
console.log(claims);
}
The oidc-client package is a JavaScript library for managing OpenID Connect (OIDC) and OAuth2 authentication. It is framework-agnostic and can be used with any JavaScript framework, including Angular. Compared to angular-oauth2-oidc, oidc-client provides more flexibility but requires more manual setup and integration.
The angular-auth-oidc-client package is another Angular library for implementing OAuth2 and OIDC authentication. It offers similar functionalities to angular-oauth2-oidc, such as token management and user profile retrieval. However, it has a different API and configuration approach, which some developers might find more intuitive or easier to use.
The auth0-angular package is an Angular SDK for integrating Auth0 authentication and authorization services. It provides a seamless way to implement OAuth2 and OIDC authentication with Auth0 as the identity provider. Compared to angular-oauth2-oidc, auth0-angular is specifically designed for use with Auth0 and offers additional features like user management and analytics.
Support for OAuth 2 and OpenId Connect (OIDC) in Angular.
Sources and Sample: https://github.com/manfredsteyer/angular-oauth2-oidc
Source Code Documentation https://manfredsteyer.github.io/angular-oauth2-oidc/angular-oauth2-oidc/docs/
Successfully tested with the Angular 2 and 4 and its Router, PathLocationStrategy as well as HashLocationStrategy and CommonJS-Bundling via webpack. At server side we've used IdentityServer (.NET/ .NET Core) and Redhat's Keycloak (Java).
setupAutomaticSilentRefresh()
loadDiscoveryDocumentAndTryLogin()
events
.token_expires
can be used togehter with a silent refresh to automatically refresh a token when/ before it expires (see also property timeoutFactor
).oidc
defaults to true
.oidc
to false
. Otherwise, the validation of the user profile will fail!sessionStorage
is used. To use localStorage
call method setStoragerequireHttps
, e. g. for local testing.strictDiscoveryDocumentValidation
.You can use the OIDC-Sample-Server mentioned in the samples for Testing. It assumes, that your Web-App runns on http://localhost:8080.
Username/Password: max/geheim
clientIds:
redirectUris:
npm i angular-oauth2-oidc --save
import { OAuthModule } from 'angular-oauth2-oidc';
[...]
@NgModule({
imports: [
[...]
HttpModule,
OAuthModule.forRoot()
],
declarations: [
AppComponent,
HomeComponent,
[...]
],
bootstrap: [
AppComponent
]
})
export class AppModule {
}
This section shows how to implement login leveraging implicit flow. This is the OAuth2/OIDC flow best suitable for Single Page Application. It sends the user to the Identity Provider's login page. After logging in, the SPA gets tokens. This also allows for single sign on as well as single sign off.
To configure the library the following sample uses the new configuration API introduced with Version 2.1. Hence, The original API is still supported.
import { AuthConfig } from 'angular-oauth2-oidc';
export const authConfig: AuthConfig = {
// Url of the Identity Provider
issuer: 'https://steyer-identity-server.azurewebsites.net/identity',
// URL of the SPA to redirect the user to after login
redirectUri: window.location.origin + '/index.html',
// The SPA's id. The SPA is registerd with this id at the auth-server
clientId: 'spa-demo',
// set the scope for the permissions the client should request
// The first three are defined by OIDC. The 4th is a usecase-specific one
scope: 'openid profile email voucher',
}
Configure the OAuthService with this config object when the application starts up:
import { OAuthService } from 'angular-oauth2-oidc';
import { JwksValidationHandler } from 'angular-oauth2-oidc';
import { authConfig } from './auth.config';
import { Component } from '@angular/core';
@Component({
selector: 'flight-app',
templateUrl: './app.component.html'
})
export class AppComponent {
constructor(private oauthService: OAuthService) {
this.configureWithNewConfigApi();
}
private configureWithNewConfigApi() {
this.oauthService.configure(authConfig);
this.oauthService.tokenValidationHandler = new JwksValidationHandler();
this.oauthService.loadDiscoveryDocumentAndTryLogin();
}
}
After you've configured the library, you just have to call initImplicitFlow
to login using OAuth2/ OIDC.
import { Component } from '@angular/core';
import { OAuthService } from 'angular-oauth2-oidc';
@Component({
templateUrl: "app/home.html"
})
export class HomeComponent {
constructor(private oauthService: OAuthService) {
}
public login() {
this.oauthService.initImplicitFlow();
}
public logoff() {
this.oauthService.logOut();
}
public get name() {
let claims = this.oAuthService.getIdentityClaims();
if (!claims) return null;
return claims.given_name;
}
}
The following snippet contains the template for the login page:
<h1 *ngIf="!name">
Hallo
</h1>
<h1 *ngIf="name">
Hallo, {{name}}
</h1>
<button class="btn btn-default" (click)="login()">
Login
</button>
<button class="btn btn-default" (click)="logoff()">
Logout
</button>
<div>
Username/Passwort zum Testen: max/geheim
</div>
Pass this Header to the used method of the Http
-Service within an Instance of the class Headers
:
var headers = new Headers({
"Authorization": "Bearer " + this.oauthService.getAccessToken()
});
If you are using the new HttpClient, use the class HttpHeaders instead:
var headers = new HttpHeaders({
"Authorization": "Bearer " + this.oauthService.getAccessToken()
});
See the documentation for more information about this library.
FAQs
Support for OAuth 2(.1) and OpenId Connect (OIDC) in Angular
The npm package angular-oauth2-oidc receives a total of 145,438 weekly downloads. As such, angular-oauth2-oidc popularity was classified as popular.
We found that angular-oauth2-oidc demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.