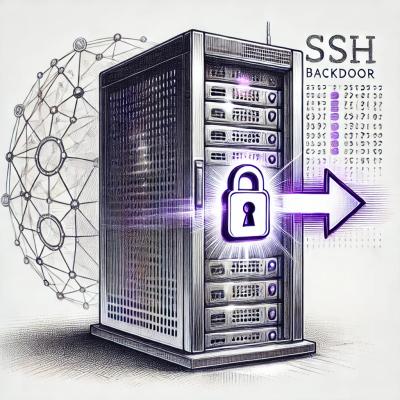
Research
Security News
Malicious npm Packages Inject SSH Backdoors via Typosquatted Libraries
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
apollo-link-context
Advanced tools
The apollo-link-context package is used to set context for Apollo Client operations. It allows you to dynamically set headers, authentication tokens, and other context values for each GraphQL request.
Setting Headers
This feature allows you to set headers for each request dynamically. In this example, an authorization token is retrieved from local storage and added to the headers of each request.
const { setContext } = require('apollo-link-context');
const { ApolloClient, InMemoryCache, HttpLink } = require('@apollo/client');
const httpLink = new HttpLink({ uri: 'https://example.com/graphql' });
const authLink = setContext((_, { headers }) => {
const token = localStorage.getItem('token');
return {
headers: {
...headers,
authorization: token ? `Bearer ${token}` : '',
}
};
});
const client = new ApolloClient({
link: authLink.concat(httpLink),
cache: new InMemoryCache()
});
Setting Context Values
This feature allows you to set custom context values for each request. In this example, a custom header and a custom context value are added to each request.
const { setContext } = require('apollo-link-context');
const { ApolloClient, InMemoryCache, HttpLink } = require('@apollo/client');
const httpLink = new HttpLink({ uri: 'https://example.com/graphql' });
const contextLink = setContext((_, { headers }) => {
return {
headers: {
...headers,
'x-custom-header': 'custom-value'
},
customValue: 'myCustomValue'
};
});
const client = new ApolloClient({
link: contextLink.concat(httpLink),
cache: new InMemoryCache()
});
The apollo-link package provides a standard interface for creating custom links to handle various aspects of GraphQL requests. It is more general-purpose compared to apollo-link-context, which is specifically for setting context.
The apollo-link-http package is used to send GraphQL operations over HTTP. It can be combined with other links like apollo-link-context to handle HTTP-specific configurations and context settings.
The apollo-link-error package allows you to handle errors in your GraphQL requests. It can be used alongside apollo-link-context to manage error handling and context setting in a unified way.
The setContext
function takes a function that returns either an object or a promise that returns an object to set the new context of a request.
It receives two arguments: the GraphQL request being executed, and the previous context. This link makes it easy to perform async look up of things like authentication tokens and more!
import { setContext } from "apollo-link-context";
const setAuthorizationLink = setContext((request, previousContext) => ({
authorization: "1234"
}));
const asyncAuthLink = setContext(
request =>
new Promise((success, fail) => {
// do some async lookup here
setTimeout(() => {
success({ token: "async found token" });
}, 10);
})
);
Typically async actions can be expensive and may not need to be called for every request, especially when a lot of request are happening at once. You can setup your own caching and invalidation outside of the link to make it faster but still flexible!
Take for example a user auth token being found, cached, then removed on a 401 response:
import { setContext } from "apollo-link-context";
import { onError } from "apollo-link-error";
// cached storage for the user token
let token;
const withToken = setContext(() => {
// if you have a cached value, return it immediately
if (token) return { token };
return AsyncTokenLookup().then(userToken => {
token = userToken;
return { token };
});
});
const resetToken = onError(({ networkError }) => {
if (networkError && networkError.statusCode === 401) {
// remove cached token on 401 from the server
token = null;
}
});
const authFlowLink = withToken.concat(resetToken);
FAQs
An easy way to set and cache context changes for Apollo Link
The npm package apollo-link-context receives a total of 243,516 weekly downloads. As such, apollo-link-context popularity was classified as popular.
We found that apollo-link-context demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.
Security News
MITRE's 2024 CWE Top 25 highlights critical software vulnerabilities like XSS, SQL Injection, and CSRF, reflecting shifts due to a refined ranking methodology.
Security News
In this segment of the Risky Business podcast, Feross Aboukhadijeh and Patrick Gray discuss the challenges of tracking malware discovered in open source softare.