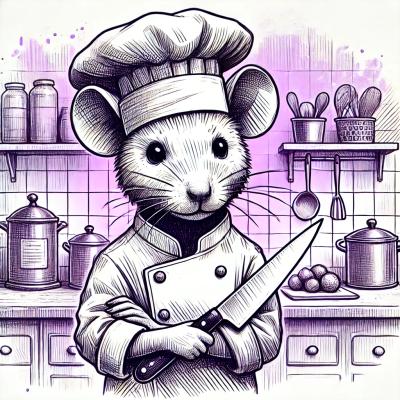
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
app-etc-config
Advanced tools
Creates a configuration API.
$ npm install app-etc-config
var etc = require( 'app-etc-config' );
Creates a configuration API.
var config = etc();
The constructor accepts the following options
:
'.'
.boolean
indicating whether to create a keypath if it does not exist. See utils-deep-set. Default: true
.To specify options
,
var config = etc({
'sep': '|',
'create': false
});
Sets a configuration value at a specified keypath
.
var bool = config.set( 'foo.bar', 'beep' );
// returns a <boolean> indicating whether a value was set
/*
{
'foo': {
'bar': 'beep'
}
}
*/
The method accepts the following options
:
boolean
indicating whether to create a keypath if it does not exist. See utils-deep-set.Specifying method options
will override the default options
provided during config
creation.
Merges a configuration object
or another config
instance.
var bool = config.merge({
'beep': 'boop'
});
// returns a <boolean> indicating if merge was successful
/*
{
'foo': {
'bar': 'beep'
},
'beep': 'boop'
}
*/
If provided a keypath
, the method merges a configuration object with a sub-configuration.
var config2 = etc();
config2.set( 'hello', 'world' );
var bool = config.merge( 'foo', config2 );
/*
{
'foo': {
'bar': 'beep',
'hello': 'world'
},
'beep': 'boop'
}
*/
If a keypath
does not correspond to an object
, the method returns false
and set()
should be used instead.
var bool = config.merge( 'abcdefg', config2 );
// returns false
/*
{
'foo': {
'bar': 'beep',
'hello': 'world'
},
'beep': 'boop'
}
*/
bool = config.set( 'abcdefg', config2 );
// returns true
The method accepts the following options
:
Specifying method options
will override the default options
provided during config
creation.
Returns a copy of the raw configuration store.
var obj = config.get();
/*
{
'foo': {
'bar': 'beep',
'hello': 'world'
},
'beep': 'boop'
}
*/
If provided a keypath
, the method returns a copy of the corresponding configuration value.
var val = config.get( 'foo.hello' );
// returns 'world'
If a keypath
does not exist, the method returns undefined
.
var val = config.get( 'non.existent.path' );
// returns undefined
The method accepts the following options
:
Specifying method options
will override the default options
provided during config
creation.
Clones a config
instance.
var config2 = config.clone();
console.log( config2.get() );
/*
{
'foo': {
'bar': 'beep',
'hello': 'world'
},
'beep': 'boop'
}
*/
console.log( config === config2 );
// returns false
If provided a keypath
, clones a sub-configuration value as a new config
instance.
var config2;
// Configuration value is an object:
config2 = config.clone( 'foo' );
/*
{
'bar': 'beep',
'hello': 'world'
}
*/
// Configuration value is not an object:
config2 = config.clone( 'beep' );
/*
{
'beep': 'boop'
}
*/
The method accepts the following options
:
Specifying method options
will override the default options
provided during config
creation.
Convenience method which loads and merges a configuration file.
config.load( '/path/to/config/file.<ext>' );
Note: this method does not directly support loading a configuration file into a sub-configuration. To achieve this, use app-etc-load and then merge
at a specified keypath
.
var load = require( 'app-etc-load' );
var obj = load( '/path/to/config/file.<ext>' );
config.merge( 'foo', obj );
Returns a list of supported filename extensions.
var exts = etc.exts();
// returns ['.json','.toml',...]
For more details, see app-etc-load.
Returns a parser for the specified extension.
var parser = etc.parser( '.json' );
Including the .
when specifying an extension is optional.
var parser = etc.parser( 'json' );
To support additional file formats or to override a parser, provide a parser
function for an associated extension.
var parser = require( 'my-special-fmt-parser' );
etc.parser( '<my-ext>', parser );
Once a parser is set, all config
instances will parse provided files accordingly.
config.load( './file.<my-ext>' );
For more details, see app-etc-load.
var etc = require( 'app-etc-config' );
// Create a new configuration API:
var config = etc();
// Load local files:
config.load( './.travis.yml' );
console.dir( config.get() );
config.load( './package.json' );
console.dir( config.get() );
// Merge in a custom object:
config.merge( 'author', {
'beep': 'boop'
});
console.dir( config.get( 'author' ) );
// Access a shallow value:
console.log( config.get( 'license' ) );
// Access nested values:
console.log( config.get( 'author.name' ) );
// Create and set shallow values:
config.set( 'hello', false );
console.log( config.get( 'hello' ) );
// Create and set deeply nested values:
config.set( 'foo.bar.bip', 'bap', {
'create': true
});
console.log( config.get( 'foo.bar.bip' ) );
// Clone the current configuration:
var clone = config.clone();
console.log( config === clone );
// returns false
To run the example code from the top-level application directory,
$ node ./examples/index.js
Unit tests use the Mocha test framework with Chai assertions. To run the tests, execute the following command in the top-level application directory:
$ make test
All new feature development should have corresponding unit tests to validate correct functionality.
This repository uses Istanbul as its code coverage tool. To generate a test coverage report, execute the following command in the top-level application directory:
$ make test-cov
Istanbul creates a ./reports/coverage
directory. To access an HTML version of the report,
$ make view-cov
Copyright © 2015. Athan Reines.
FAQs
Creates a configuration API.
The npm package app-etc-config receives a total of 0 weekly downloads. As such, app-etc-config popularity was classified as not popular.
We found that app-etc-config demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.