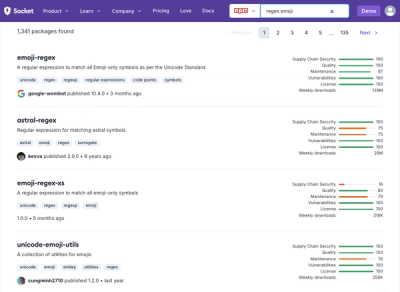
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Fast and powerful array sorting. Sort an array of objects by one or more properties. Any number of nested properties or custom comparison functions may be used.
The array-sort npm package is a small, fast, and dependency-free library for sorting arrays of objects based on one or more properties. It allows for complex sorting mechanisms including sorting by nested properties, custom comparator functions, and multiple criteria.
Simple sorting
Sorts an array of objects by a single property. In this example, it sorts the array of users by their names in ascending order.
const sort = require('array-sort');
const users = [{ name: 'John' }, { name: 'Amy' }];
sort(users, 'name');
Descending order sorting
Sorts an array of objects by a property in descending order. Here, users are sorted by age in descending order using the '-' prefix.
const sort = require('array-sort');
const users = [{ age: 30 }, { age: 24 }];
sort(users, '-age');
Multiple criteria sorting
Sorts an array of objects by multiple properties. This example sorts users first by name and then by age in ascending order.
const sort = require('array-sort');
const users = [{ name: 'John', age: 25 }, { name: 'Amy', age: 22 }];
sort(users, ['name', 'age']);
Custom comparator function
Allows for custom sorting logic using a comparator function. This example sorts users by name using a locale-aware comparison.
const sort = require('array-sort');
const users = [{ name: 'John' }, { name: 'Amy' }];
sort(users, (a, b) => a.name.localeCompare(b.name));
Lodash's orderBy function offers similar functionality to array-sort, allowing sorting by multiple criteria and in different orders. It is part of the larger lodash library, which might not be ideal for projects looking to minimize dependencies.
Sort-array is another npm package that provides sorting of arrays based on multiple fields. It is similar to array-sort but also includes additional options for case sensitivity and explicit ascending/descending orders.
Fast and powerful array sorting. Sort an array of objects by one or more properties. Any number of nested properties or custom comparison functions may be used.
Install with npm
$ npm i array-sort --save
Sort an array by the given object property:
var arraySort = require('array-sort');
arraySort([{foo: 'y'}, {foo: 'z'}, {foo: 'x'}], 'foo');
//=> [{foo: 'x'}, {foo: 'y'}, {foo: 'z'}]
Reverse order
arraySort([{foo: 'y'}, {foo: 'z'}, {foo: 'x'}], 'foo', {reverse: true});
//=> [{foo: 'z'}, {foo: 'y'}, {foo: 'x'}]
(Table of contents generated by verb)
arraySort(array, comparisonArgs);
array
: {Array} The array to sortcomparisonArgs
: {Function|String|Array}: One or more functions or object paths to use for sorting.var arraySort = require('array-sort');
var posts = [
{ path: 'c.md', locals: { date: '2014-01-09' } },
{ path: 'a.md', locals: { date: '2014-01-02' } },
{ path: 'b.md', locals: { date: '2013-05-06' } },
];
// sort by `locals.date`
console.log(arraySort(posts, 'locals.date'));
// sort by `path`
console.log(arraySort(posts, 'path'));
var arraySort = require('array-sort');
var posts = [
{ locals: { foo: 'bbb', date: '2013-05-06' }},
{ locals: { foo: 'aaa', date: '2012-01-02' }},
{ locals: { foo: 'ccc', date: '2014-01-02' }},
{ locals: { foo: 'ccc', date: '2015-01-02' }},
{ locals: { foo: 'bbb', date: '2014-06-01' }},
{ locals: { foo: 'aaa', date: '2014-02-02' }},
];
// sort by `locals.foo`, then `locals.date`
var result = arraySort(posts, ['locals.foo', 'locals.date']);
console.log(result);
// [ { locals: { foo: 'aaa', date: '2012-01-02' } },
// { locals: { foo: 'aaa', date: '2014-02-02' } },
// { locals: { foo: 'bbb', date: '2013-05-06' } },
// { locals: { foo: 'bbb', date: '2014-06-01' } },
// { locals: { foo: 'ccc', date: '2014-01-02' } },
// { locals: { foo: 'ccc', date: '2015-01-02' } } ]
If custom functions are supplied, array elements are sorted according to the return value of the compare function. See the docs for Array.sort()
for more details.
var arr = [
{one: 'w', two: 'b'},
{one: 'z', two: 'a'},
{one: 'x', two: 'c'},
{one: 'y', two: 'd'},
];
function compare(prop) {
return function (a, b) {
return a[prop].localeCompare(b[prop]);
};
}
var result = arraySort(arr, function (a, b) {
return a.two.localeCompare(b.two);
});
console.log(result);
// [ { one: 'z', two: 'a' },
// { one: 'w', two: 'b' },
// { one: 'x', two: 'c' },
// { one: 'y', two: 'd' } ]
var arr = [
{foo: 'w', bar: 'y', baz: 'w'},
{foo: 'x', bar: 'y', baz: 'w'},
{foo: 'x', bar: 'y', baz: 'z'},
{foo: 'x', bar: 'x', baz: 'w'},
];
// reusable compare function
function compare(prop) {
return function (a, b) {
return a[prop].localeCompare(b[prop]);
};
}
// the `compare` functions can be a list or array
var result = arraySort(arr, compare('foo'), compare('bar'), compare('baz'));
console.log(result);
// [ { foo: 'w', bar: 'y', baz: 'w' },
// { foo: 'x', bar: 'x', baz: 'w' },
// { foo: 'x', bar: 'y', baz: 'w' },
// { foo: 'x', bar: 'y', baz: 'z' } ]
a.b.c
) to get a nested value from an object.'a.b.c'
) paths.Install dev dependencies:
$ npm i -d && npm test
Pull requests and stars are always welcome. For bugs and feature requests, please create an issue
Jon Schlinkert
Copyright © 2015 Jon Schlinkert Released under the MIT license.
This file was generated by verb-cli on July 18, 2015.
FAQs
Fast and powerful array sorting. Sort an array of objects by one or more properties. Any number of nested properties or custom comparison functions may be used.
The npm package array-sort receives a total of 1,121,347 weekly downloads. As such, array-sort popularity was classified as popular.
We found that array-sort demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.