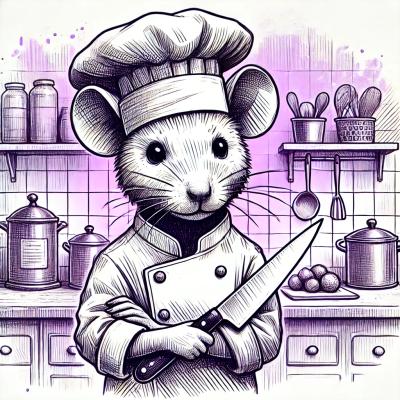
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
This project is a tentative to create a simple JavaScript AST modification library.
If you've ever worked with AST trying to edit source code, you'll know it is a bad time. AST syntax is terse and forces you to loop a tree and use conditional structure a lot. AST Query hide these complexities behind a declarative façade.
Making the simplicity choice means AST Query won't try to cover the full AST API. Rather we strive to answer commons needs.
Install: npm install --save ast-query
First, you need to pass a program code into AST query:
var program = require("ast-query");
var tree = program("var a = 'foo'");
This function returns a wrapped AST tree you can query and modify.
Once you've modified the AST, get the source code back by calling the toString
method on the tree.
// ...
tree.var("a").value("'bar'");
console.log( tree.toString() );
// LOG: var a = 'bar';
Remember that you are editing source code. This mean you provide raw source code strings. This mean you need to double wrap strings (e.g.: "'foo'"
). If that's not done, AST-query assume you're referencing a variable called foo
.
var tree = program( sourceCode, escodegenOptions, esprimaOptions )
Returns an AST tree you can then query as explained below:
tree.var( name )
Find and returns a Variable
node.
Given this code
var bar = 23;
You'd call tree.var('bar')
to get the Variable node.
tree.callExpression( name )
Find a function or method call and return a CallExpression
node
Given this code
grunt.initConfig({});
You'd call tree.callExpression('grunt.initConfig')
to get the CallExpression node.
tree.assignment( assignedTo )
Find and return an AssignmentExpression
node.
You'd call tree.assignment('module.exports')
to query the code below:
module.exports = function () {
// code
};
tree.body
Property representing the program body in a Body
node.
tree.verbatim( body )
Adds body and return a token assigment.
tree.body.append('var a = 1;' + tree.verbatim('ANYTHING'));
.value( value )
It returns the current or new value wrapped in AST query interface.
.rename( name )
.filter( iterator )
Return a new CallExpression nodes collection with nodes passing the iterator test.
.arguments
A property pointing to an ArrayExpression
node referencing the called function arguments.
.value( value )
Replace the assignment value with a new value or return the current value wrapped in an AST query interface.
A Literal node represent a raw JavaScript value as a String, a Number or a Boolean.
.value( value )
Get or update the value.
Node representing a function declaration (e.g. function () {}
).
.body
Property pointing to a Body
node representing the function expression body.
.key( name )
value( value )
Replace current node with a new value. Returns the new value wrapped.
.push( value )
.unshift( value )
.at( index )
Returns a value wrapped in an AST query interface.
value( value )
Replace current node with a new value. Returns the new value wrapped.
.prepend( code )
Preprend the given code lines in the body. If a "use strict";
statement is present, it always stay first.
.append( code )
Append the given code lines in the body.
Style Guide: Please base yourself on Idiomatic.js
style guide with two space indent
Unit test: Unit test are wrote in Mocha. Please add a unit test for every new feature
or bug fix. npm test
to run the test suite.
Documentation: Add documentation for every API change. Feel free to send corrections
or better docs!
Pull Requests: Send fixes PR on the master
branch. Any new features should be send
on the wip
branch.
Copyright (c) 2013 Simon Boudrias (twitter: @vaxilart) Licensed under the MIT license.
FAQs
Declarative JavaScript AST modification façade
We found that ast-query demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.