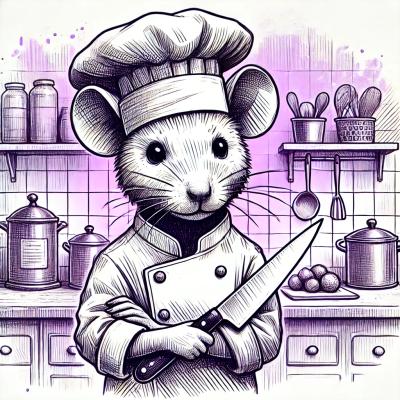
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
async-deco
Advanced tools
A collection of decorators for adding features to asynchronous functions (callback or promise based).
async-deco is a collection of decorators. It allows to add features such as timeout, retry, dedupe, limit and much more! They can be combined together using the "compose" function (included).
All decorators are designed to work with functions using a callback or returning a promise. In case of callbacks, it must follow the node convention: the callback should be the last argument and its arguments should be, an error instance and the output of the function.
Every decorator is available in two different flavours:
var logDecorator = require('async-deco/callback/log');
This should be applied to functions with the node callback convention:
var decoratedFunction = logDecorator(logger)(function (a, b, c, next) {
...
next(undefined, result); // or next(error);
});
var logDecorator = require('async-deco/promise/log');
This should be used for function returning promises:
var decoratedFunction = logDecorator(logger)(function (a, b, c) {
return new Promise(function (resolve, reject) {
...
resolve(result); // or reject(error);
});
});
Then you can run the decorated function.
You can either:
var memoizeDecorator = require('async-deco/callback/memoize');
or
var memoizeDecorator = require('async-deco').callback.memoize;
or
var callbackDecorators = require('async-deco');
var memoizeDecorator = callbackDecorators.callback.memoize;
I strongly advice to use the first method, especially when using browserify. It allows to import only the functions you are actually using.
You can pass a logger to any decorators. This function is called with the same arguments of the original function and should return a function with this signature:
function (type, obj)
So for example, assuming that the first argument of the decorated function is a name:
var logger = function (name) {
return function (type, obj) {
console.log(name + ': ' + type);
};
};
The examples are related to the callback version. Just import the promise version in case of decorating promise based functions.
It caches the result. At any subsequent calls it will return the cached result.
var memoizeDecorator = require('async-deco/callback/memoize');
var simpleMemoize = memoizeDecorator(getKey, logger);
var myfunc = simpleMemoize(function (..., cb) { .... });
It takes 2 arguments:
It is a more sophisticated version of the memoize decorator. It can be used for caching in a db/file etc (You may have to write your own cache object). memoize-cache is an in-memory reference implementation (https://github.com/sithmel/memoize-cache).
var cacheDecorator = require('async-deco/callback/cache');
var cached = cacheDecorator(cache, logger);
var myfunc = cached(function (..., cb) { .... });
It takes 2 arguments:
It executes a "guard" function before the original one. If it returns an error it will use this error as the return value of the original function. It is useful if you want to run a function only if it passes some condition (access control).
var proxyDecorator = require('async-deco/callback/proxy');
var proxy = cacheDecorator(function (..., cb) {
// calls cb(errorInstance) if the access is denied
// calls cb() if I can procede calling the function
}, logger);
var myfunc = proxy(function (..., cb) { .... });
It takes 2 arguments:
If a function fails, calls another one
var fallbackDecorator = require('async-deco/callback/fallback');
var fallback = fallbackDecorator(function (a, b, c, func) {
func(undefined, 'giving up');
}, Error, logger);
var myfunc = fallback(function (..., cb) { .... });
It takes 3 arguments:
If a function fails, returns a value
var fallbackValueDecorator = require('async-deco/callback/fallback-value');
var fallback = fallbackValueDecorator('giving up', Error, logger);
var myfunc = fallback(function (..., cb) { .... });
It takes 3 arguments:
If a function fails, it tries to use a previous cached result.
var fallbackCacheDecorator = require('async-deco/callback/fallback-cache');
var fallback = fallbackCacheDecorator(cache, Error, logger);
var myfunc = fallback(function (..., cb) { .... });
It takes 3 arguments:
Logs when a function start, end and fail
ar logDecorator = require('async-deco/callback/log');
var addLogs = logDecorator(logger);
var myfunc = addLogs(function (..., cb) { .... });
If a function takes to much, returns a timeout exception.
var timeoutDecorator = require('async-deco/callback/timeout');
var timeout20 = timeoutDecorator(20, logger);
var myfunc = timeout20(function (..., cb) { .... });
This will wait 20 ms before returning a TimeoutError. It takes 2 arguments:
If a function fails, it retry running it again
var retryDecorator = require('async-deco/callback/retry');
var retryTenTimes = retryDecorator(10, 0, Error, logger);
var myfunc = retryTenTimes(function (..., cb) { .... });
You can initialise the decorator with 3 arguments:
Limit the concurrency of a function.
var limitDecorator = require('async-deco/callback/limit');
var limitToTwo = limitDecorator(2, getKey,logger);
var myfunc = limitToTwo(function (..., cb) { .... });
You can initialise the decorator with 2 arguments:
It executes the original function, while is waiting for the output it doesn't call the function anymore but instead it collects the callbacks. After getting the result, it dispatches the same to all callbacks. It may use the "getKey" function to group the callbacks into queues.
var dedupeDecorator = require('async-deco/callback/dedupe');
var dedupe = dedupeDecorator(getKey, getLogger);
var myfunc = dedupe(function (..., cb) { .... });
The arguments:
Convert a synchronous/promise based function to a plain callback.
var callbackify = require('async-deco/utils/callbackify');
var func = callbackify(function (a, b){
return a + b;
});
func(4, 6, function (err, result){
... // result === 10 here
})
Convert a callback based function to a function returning a promise. (It uses https://www.npmjs.com/package/es6-promisify)
var promisify = require('async-deco/utils/promisify');
var func = promisify(function (a, b, next){
return next(undefined, a + b);
});
func(4, 6).then(function (result){
... // result === 10 here
})
It can combine more than one decorators. You can pass either an array or using multiple arguments. "undefined" items are ignored.
var compose = require('async-deco/utils/compose');
var decorator = compose(
retryDecorator(10, Error, logger),
timeoutDecorator(20, logger));
var newfunc = decorator(function (..., cb) { .... });
Timeout after 20 ms and then retry 10 times before giving up. You should consider the last function is the one happen first!
Using memoize(or cache) on an asynchronous function has a conceptual flaw. Let's say for example I have a function with 100ms latency. I call this function every 10 ms:
executed ⬇⬇⬇⬇⬇⬇⬇⬇⬇⬇
------------------------------
requested ⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆
What happen is that while I am still waiting for the first result (to cache) I regularly execute other 9 functions. What if I compose memoize with dedupe?
var decorator = compose(dedupeDecorator(), memoizeDecorator());
var newfunc = decorator(function (..., cb) { .... });
dedupe should fill the gap:
executed ⬇
------------------------------
requested ⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆⬆
Imagine a case in which you want to be sure you did everything to get a result, and in case is not possible you want to return a sane fallback:
var decorator = compose(
fallbackDecorator(getFallbackResult), // last resort fallback
fallbackCacheDecorator(cache), // try to use a previous cached output
retryDecorator(3), // it retry 3 times
timeoutDecorator(5000)); // it times out after 5 seconds
var newfunc = decorator(function (..., cb) { .... });
FAQs
A collection of decorators for adding features to asynchronous functions
The npm package async-deco receives a total of 3 weekly downloads. As such, async-deco popularity was classified as not popular.
We found that async-deco demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.