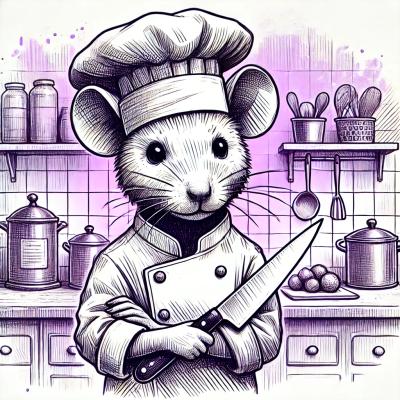
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
asynchronize
Advanced tools
Make synchronous functions look asynchronous.
Current Version: 1.2.0
Build Status:
Node Support: 0.6, 0.8, 0.10
Browser Support: Android Browser 2.2–4.2, Firefox 3.6, Firefox 4–19, Google Chrome 14–26, Internet Explorer 6–10, Mobile Safari iOS 3–6, Opera 12.10, Safari 5–6
I needed a simple way to make synchronous JavaScript functions look asynchronous, for use in control flow libraries like async. Thus, Asynchronize was born.
As an oversimplified example, if you have a function which takes an input and returns an output which works like this:
var result = lowercase('FOO');
console.log(result); // 'foo'
Asynchronize will turn it into a function which works like this:
lowercase('FOO', function (error, result) {
console.log(result); // 'foo'
});
You can use Asynchronize on the server side with Node.js and npm:
$ npm install asynchronize
On the client side, you can either install Asynchronize through Bower/Component:
$ bower install asynchronize
$ component install rowanmanning/asynchronize
$ component install rowanmanning/asynchronize
or by simply including asynchronize.js
in your page:
<script src="path/to/lib/asynchronize.js"></script>
In Node.js or using Component, you can include Asynchronize in your script by using require:
var asynchronize = require('asynchronize');
Asynchronize also works with AMD-style module loaders, just specify it as a dependency.
If you're just including with a <script>
, asynchronize
is available as a function in the global scope.
Create an async-style version of fn
.
fn: (function) The function to convert.
return: (function) Returns the new async-style function.
function lowercase (val) {
return val.toLowerCase();
}
var lowercaseAsync = asynchronize(lowercase);
The created function accepts any number of arguments, the last one being used as the callback if it's a function. The callback is called with two arguments; an error object (the result of a throw
within the original function), and a result (the return value of the original function).
Let's extend the example to throw errors:
function lowercase (val) {
if (typeof val !== 'string') {
throw new Error('Expected string');
}
return val.toLowerCase();
}
var lowercaseAsync = asynchronize(lowercase);
// Call with string
lowercaseAsync('FOO', function (error, result) {
console.log(error); // undefined
console.log(result); // 'foo'
});
// Call with non-string
lowercaseAsync(123, function (error, result) {
console.log(error); // new Error('Expected string')
console.log(result); // undefined
});
To develop Asynchronize, you'll need to clone the repo and install dependencies with make deps
. If you're on Windows, you'll also need to install Make for Windows.
Once you're set up, you can run the following commands:
$ make deps # Install dependencies
$ make lint # Run JSHint with the correct config
$ make test # Run unit tests in Node
$ make test-server # Run a server for browser unit testing (visit localhost:3000)
When no build target is specified, make will run deps lint test
. This means you can use the following command for brevity:
$ make
Code with lint errors or no/failing tests will not be accepted, please use the build tools outlined above.
Asynchronize is licensed under the MIT license.
FAQs
Make synchronous functions look asynchronous
The npm package asynchronize receives a total of 4 weekly downloads. As such, asynchronize popularity was classified as not popular.
We found that asynchronize demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.