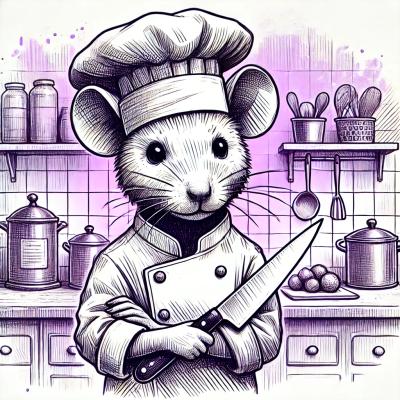
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
The asynckit npm package provides a minimalistic asynchronous control flow kit that offers a set of utilities for working with asynchronous operations in Node.js. It allows for sequential and parallel execution of asynchronous functions, as well as other utilities for handling streams and iterable collections.
Serial execution of async tasks
Executes an array of async tasks one after another. Each task is started only after the preceding task has completed. The results are collected in an array that is passed to the final callback.
const asyncKit = require('asynckit');
const fs = require('fs');
asyncKit.serial([
callback => fs.readFile('file1.txt', 'utf8', callback),
callback => fs.readFile('file2.txt', 'utf8', callback)
], (err, results) => {
if (err) throw err;
console.log(results); // results is an array of the contents of the two files
});
Parallel execution of async tasks
Executes an array of async tasks in parallel. All tasks are started at the same time, and the final callback is called when all tasks have completed. The results are collected in an array.
const asyncKit = require('asynckit');
const fs = require('fs');
asyncKit.parallel([
callback => fs.readFile('file1.txt', 'utf8', callback),
callback => fs.readFile('file2.txt', 'utf8', callback)
], (err, results) => {
if (err) throw err;
console.log(results); // results is an array of the contents of the two files
});
Stream processing
Provides a way to pipe data from a readable stream to a writable stream with the ability to handle errors that may occur during the streaming process.
const asyncKit = require('asynckit');
const stream = require('stream');
const readable = new stream.Readable();
const writable = new stream.Writable();
// Implement _read and _write methods for the streams
// ...
asyncKit.stream(readable, writable, (err) => {
if (err) throw err;
console.log('Stream processing complete');
});
The 'async' package is a comprehensive collection of asynchronous control flow utilities. It offers a wide range of functions for parallel, series, and other complex flow controls. Compared to asynckit, 'async' is more feature-rich and widely used, but it is also larger in size.
Bluebird is a fully-featured promise library that allows for converting callback-based APIs to promises. It includes utilities for sequential and parallel execution of tasks. While asynckit uses callbacks, Bluebird focuses on promises, which can lead to cleaner code with less nesting.
Q is another promise library that provides tools for creating and managing promises. It is similar to Bluebird in that it helps with asynchronous flow control but uses a different API. Asynckit is callback-based, whereas Q is promise-based.
Minimal async jobs utility library.
compression | size |
---|---|
asynckit.js | 2.27 kB |
asynckit.min.js | 958 B |
asynckit.min.js.gz | 491 B |
$ npm install asynckit --save
Runs iterator over provided array in parallel. Stores output in the result
array,
on the matching positions. In unlikely event of an error from one of the jobs,
will terminate rest of the active jobs (if abort function is provided)
and return error along with salvaged data to the main callback function.
var parallel = require('asynckit/parallel')
, assert = require('assert')
;
var source = [ 1, 1, 4, 16, 64, 32, 8, 2 ]
, expectedResult = [ 2, 2, 8, 32, 128, 64, 16, 4 ]
, expectedTarget = [ 1, 1, 2, 4, 8, 16, 32, 64 ]
, target = []
;
parallel(source, asyncJob, function(err, result)
{
assert.deepEqual(result, expectedResult);
assert.deepEqual(target, expectedTarget);
});
// async job accepts one element from the array
// and a callback function
function asyncJob(item, cb)
{
// different delays (in ms) per item
var delay = item * 25;
// pretend different jobs take different time to finish
// and not in consequential order
var timeoutId = setTimeout(function() {
target.push(item);
cb(null, item * 2);
}, delay);
// allow to cancel "leftover" jobs upon error
// return function, invoking of which will abort this job
return clearTimeout.bind(null, timeoutId);
}
More examples can be found in test folder.
Or open an issue with questions and/or suggestions.
AsyncKit is licensed under the MIT license.
FAQs
Minimal async jobs utility library, with streams support
The npm package asynckit receives a total of 35,778,762 weekly downloads. As such, asynckit popularity was classified as popular.
We found that asynckit demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.