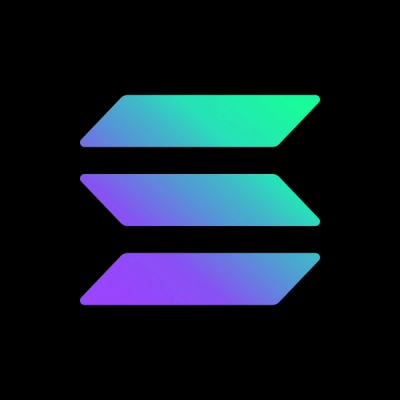
Security News
Supply Chain Attack Detected in Solana's web3.js Library
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
aws-xray-sdk
Advanced tools
The aws-xray-sdk is an npm package that provides tools for integrating AWS X-Ray tracing into your Node.js applications. AWS X-Ray helps developers analyze and debug distributed applications, such as those built using a microservices architecture. The SDK allows you to trace requests as they travel through your application, providing insights into performance bottlenecks and errors.
Automatic Instrumentation
This feature allows you to automatically capture HTTP and HTTPS requests made by your application. The SDK wraps the native HTTP and HTTPS modules to capture and record data about outgoing requests.
const AWSXRay = require('aws-xray-sdk');
const http = AWSXRay.captureHTTPs(require('http'));
const https = AWSXRay.captureHTTPs(require('https'));
http.get('http://www.example.com', (res) => {
res.on('data', (chunk) => {
console.log(`BODY: ${chunk}`);
});
res.on('end', () => {
console.log('No more data in response.');
});
});
Manual Instrumentation
This feature allows you to manually create and manage subsegments within your code. You can add annotations and metadata to these subsegments to provide additional context for your traces.
const AWSXRay = require('aws-xray-sdk');
AWSXRay.captureFunc('annotations', function(subsegment) {
subsegment.addAnnotation('userId', '12345');
subsegment.addMetadata('key', 'value');
});
Middleware Integration
This feature allows you to integrate AWS X-Ray with popular web frameworks like Express. The SDK provides middleware that automatically creates and closes segments for incoming HTTP requests.
const AWSXRay = require('aws-xray-sdk');
const express = require('express');
const app = express();
app.use(AWSXRay.express.openSegment('MyApp'));
app.get('/', function (req, res) {
res.send('Hello World!');
});
app.use(AWSXRay.express.closeSegment());
app.listen(3000);
Jaeger is an open-source end-to-end distributed tracing tool originally developed by Uber Technologies. The jaeger-client npm package allows you to instrument your Node.js applications to send trace data to a Jaeger backend. Unlike aws-xray-sdk, Jaeger is designed to work with the Jaeger tracing system and provides more flexibility in terms of deployment and configuration.
npm install
npm test
grunt docs
AWS SDK v2.7.15 or greater.
AWS X-Ray automatically records information for incoming and outgoing requests and responses, as well as local data such as function calls, time, variables (via metadata and annotations), even EC2 instance data. Currently only supports Express applications for auto capturing.
The AWS X-Ray SDK has two modes - Manual and CLS. CLS mode uses the Continuation Local Storage package and automatically keeps track of the current segment and subsegment. This is the default mode. Manual mode requires you pass around the segment reference.
In CLS mode, you can get the current segment/subsegment at any time: var segment = AWSXRay.getSegment();
In manual mode, you can get the base segment off the request object: var segment = req.segment;
The SDK exposes the Segment and Subsegment objects to create your own capturing mechanisms, but a few are supplied. These keep the current subsegment up to date automatically using CLS.
AWSXRay.capture - Takes a function that takes a single subsegment argument. This will create a new nested subsegment and expose it. The segment will close automatically when the function completes executing. This will not correctly time functions with asynchronous calls, instead use captureAsync.
AWSXRay.captureAsync - Takes a function that takes a single subsegment argument. This will create a new nested subsegment and expose it. The segment must be closed manually using subsegment.close() or subsegment.close(error) when the asynchronous function completes.
AWSXRay.captureCallback - Takes a function to be used as a callback. Useful for capturing callback information and directly associate it to the call that generated it. This will create a new nested subsegment and expose it by appending it onto the arguments used to call the callback. For this reason, always call your captured callbacks with the full parameter list. The subsegment will close automatically when the function completes executing.
Environment variables always override values set in code.
AWS_XRAY_DEBUG_MODE Enables logging to console output. Logging to a file is no longer built in, see 'configure logging' below.
AWS_XRAY_TRACING_NAME For overriding the default segment name to be used with the middleware. See 'dynamic and fixed naming modes'.
XRAY_TRACING_NAME For overriding the default segment name to be used with the middleware. See 'dynamic and fixed naming modes'.
**This will be deprecated in favor of AWS_XRAY_TRACING_NAME for the GA release.
AWS_XRAY_DAEMON_ADDRESS For setting the daemon address and port. Expects 'x.x.x.x', ':yyyy' or 'x.x.x.x:yyyy' IPv4 formats.
POSTGRES_DATABASE_VERSION Sets additional data for the sql subsegment.
POSTGRES_DRIVER_VERSION Sets additional data for the sql subsegment.
MYSQL_DATABASE_VERSION Sets additional data for the sql subsegment.
MYSQL_DRIVER_VERSION Sets additional data for the sql subsegment.
By default, the SDK expects the daemon to be at 127.0.0.1 (localhost) on port 2000. You can override the address, port, or both. This can be changed via the environment variables listed above, or through code. The same format is applicable for both.
AWSXRay.setDaemonAddress('186.34.0.23:8082');
AWSXRay.setDaemonAddress(':8082');
AWSXRay.setDaemonAddress('186.34.0.23');
Default logging to a file has been removed. To set up file logging, please configure a logger which responds to debug, info, warn and error functions. To log information about configuration, make sure you set the logger before other configuration options.
AWSXRay.setLogger(logger);
A sampling rule defines the rate at which requests are sampled for a particular endpoint, HTTP method and URL of the incoming request. In this way, you can change the behavior of sampling using http_method, service_name, url_path attributes to specify the route, then use fixed_target and rate to determine sampling rates.
Fixed target refers to the maximum number of requests to sample per second. When this threshold has been reached, the sampling decision will use the specified percentage (rate) to sample on.
The SDK comes with a default sampling file at /lib/resources/sampling_rules.js. You can choose to override this by providing one.
AWSXRay.middleware.setSamplingRules(<path to file>);
A sampling file must have a "default" defined. The default matches all routes as a fall-back, if none of the rules match.
{
"rules": [],
"default": {
"fixed_target": 10,
"rate": 0.05
},
"version": 1
}
Order of priority is determined by the spot in the rules array, top being highest priority. The default is always checked last. Service name, URL path and HTTP method patterns are case insensitive, and use a string with wild cards as the pattern format. A '*' represents any number of characters, while '?' represents a single character. Description is optional.
{
"rules": [
{
"description": "Sign-in request",
"http_method": "GET",
"service_name": "*.foo.com",
"url_path": "/signin/*",
"fixed_target": 10,
"rate": 0.05
}
],
"default": {
"fixed_target": 10,
"rate": 0.05
},
"version": 1
}
The SDK requires a default segment name to be set when using the middleware. If it is not set, an error will be thrown. This value can be overridden via the AWS_XRAY_TRACING_NAME (or XRAY_TRACING_NAME) environment variable.
app.use(AWSXRay.express.openSegment('defaultName'));
The SDK defaults to a fixed naming mode. This means that each time a new segment is created for an incoming request, the name of that segment is set to the default name.
In dynamic mode, the segment name can vary between the host header of the request or the default name.
AWSXRay.middleware.enableDynamicNaming(<pattern>);
If no pattern is provided, the host header is used as the segment name. If no host header is present, the default is used. This is equivalent to using the pattern '*'.
If a pattern is provided, in the form of a string with wild cards (ex: '..us-east-?.elasticbeanstalk.com') then the host header of the request will be checked against it. A '*' represents any number of characters, while '?' represents a single character. If the host header is present and matches this pattern, it is used as the segment name. Otherwise, the default name is used.
By default, the SDK is configured to have a threshold of 100 subsegments per segment. This is because the UDP packet maximum size is ~65 kb, and larger segments may trigger the 'Segment too large to send' error.
To remedy this, the SDK will automatically send the completed subsegments to the daemon when the threshold has been breached. Additionally, subsegments that complete when over threshold automatically send themselves. If a subsegment is sent out-of-band, it will be pruned from the segment object. The full segment will be reconstructed on the service-side. You can change the threshold as needed.
AWSXRay.setStreamingThreshold(10);
Subsegments may be marked as 'in_progress' when sent to the daemon. The SDK is telling the service to anticipate the asynchronous subsegment to be received out-of-band when it has completed. When received, the in_progress subsegment will be discarded in favor of the completed subsegment.
Use the 'npm start' script to enable.
var app = express();
//...
var AWSXRay = require('aws-xray-sdk');
app.use(AWSXRay.express.openSegment('defaultName')); //required at the start of your routes
app.get('/', function (req, res) {
res.render('index');
});
app.use(AWSXRay.express.closeSegment()); //required at the end of your routes / first in error handling routes
var AWS = captureAWS(require('aws-sdk'));
//create new clients as per usual
//make sure any outgoing calls that are dependent on another async
//function are wrapped with captureAsync, otherwise duplicate segments may leak
//see usages for clients in manual and CLS modes
var AWSXRay = require('aws-xray-sdk');
AWSXRay.config([AWSXRay.plugins.EC2]);
var key = 'hello';
var value = 'there'; // must be string, boolean or finite number
subsegment.addAnnotation(key, value);
var key = 'hello';
var value = 'there';
subsegment.addMetadata(key, value);
var newSubseg = subsegment.addNewSubsegment(name);
// or
var subsegment = new Subsegment(name);
var app = express();
//...
var AWSXRay = require('aws-xray-sdk');
app.use(AWSXRay.express.openSegment('defaultName'));
app.get('/', function (req, res) {
res.render('index');
});
app.use(AWSXRay.express.closeSegment());
var AWSXRay = require('aws-xray-sdk');
app.use(AWSXRay.express.openSegment('defaultName'));
app.get('/', function (req, res) {
var host = 'samplego-env.us-east-1.elasticbeanstalk.com';
AWSXRay.captureAsync('send', function(seg) {
sendRequest(host, function() {
console.log("rendering!");
res.render('index');
seg.close();
});
});
});
app.use(AWSXRay.express.closeSegment());
function sendRequest(host, cb) {
var options = {
host: host,
path: '/',
};
var callback = function(response) {
var str = '';
response.on('data', function (chunk) {
str += chunk;
});
response.on('end', function () {
cb();
});
}
http.request(options, callback).end();
};
var s3 = AWSXRay.captureAWSClient(new AWS.S3());
//use client as usual
//make sure any outgoing calls that are dependent on another async
//function are wrapped with captureAsync, otherwise duplicate segments may leak
var aws = AWSXRay.captureAWS(require('aws-sdk'));
//create new clients as per usual
//make sure any outgoing calls that are dependent on another async
//function are wrapped with captureAsync, otherwise duplicate segments may leak
AWSXRay.captureHTTPs(http); //patching the http module will patch for https as well
var options = {
...
}
http.request(options, callback).end();
//create new requests as per usual
//make sure any outgoing calls that are dependent on another async
//function are wrapped with captureAsync, otherwise duplicate segments may leak
var AWSXRay = require('aws-xray-sdk');
var pg = AWSXRay.capturePostgres(require('pg'));
...
var client = new pg.Client();
client.connect(function (err) {
...
client.query({name: 'moop', text: 'SELECT $1::text as name'}, ['brianc'], function (err, result) {
//automatically captures query information and error (if any)
});
});
...
var pool = new pg.Pool(config);
pool.connect(function(err, client, done) {
if(err) {
return console.error('error fetching client from pool', err);
}
var query = client.query('SELECT * FROM mytable', function(err, result) {
//automatically captures query information and error (if any)
});
});
var AWSXRay = require('aws-xray-sdk');
var mysql = AWSXRay.captureMySQL(require('mysql'));
var config = { ... };
...
var connection = mysql.createConnection(config);
connection.query('SELECT * FROM cats', function(err, rows) {
//automatically captures query information and error (if any)
});
...
var pool = mysql.createPool(config);
var segment = req.segment;
pool.query('SELECT * FROM cats', function(err, rows, fields) {
//automatically captures query information and error (if any)
}
Enable manual mode:
AWSXRay.enableManualMode();
var AWSXRay = require('aws-xray-sdk');
AWSXRay.enableManualMode();
app.use(AWSXRay.express.openSegment('defaultName'));
app.get('/', function (req, res) {
var segment = req.segment;
var host = 'samplego-env.us-east-1.elasticbeanstalk.com';
AWSXRay.captureAsync('send', function(seg) {
sendRequest(host, function() {
console.log("rendering!");
res.render('index');
seg.close();
});
}, segment);
});
app.use(AWSXRay.express.closeSegment());
function sendRequest(host, cb, segment) {
var options = {
host: host,
path: '/',
Segment: segment
};
var callback = function(response) {
var str = '';
//the whole response has been received, so we just print it out here
//another chunk of data has been received, so append it to `str`
response.on('data', function (chunk) {
str += chunk;
});
response.on('end', function () {
cb();
});
}
http.request(options, callback).end();
};
var s3 = AWSXRay.captureAWSClient(new AWS.S3());
var params = {
Bucket: bucketName,
Key: keyName,
Body: 'Hello!',
Segment: subsegment //required "Segment" param
};
s3.putObject(params, function(err, data) {
...
});
var AWS = captureAWS(require('aws-sdk'));
//create new clients as per usual
//make sure any outgoing calls that are dependent on another async
//functions are wrapped, otherwise duplicate segments may leak.
AWSXRay.captureHTTPs(http); //patching the http module will patch for https as well
...
//include segment/subsegment reference in options as 'Segment'
var options = {
...
Segment: subsegment
}
http.request(options, callback).end();
var AWSXRay = require('aws-xray-sdk');
var pg = AWSXRay.capturePostgres(require('pg'));
...
var client = new pg.Client();
client.connect(function (err) {
...
client.query({name: 'moop', text: 'SELECT $1::text as name'}, ['mcmuls'], function (err, result) {
//automatically captures query information and error (if any)
});
});
...
var pool = new pg.Pool(config);
pool.connect(function(err, client, done) {
if(err) {
return console.error('error fetching client from pool', err);
}
var query = client.query('SELECT * FROM mytable', function(err, result) {
//automatically captures query information and error (if any)
}, segment));
};
var AWSXRay = require('aws-xray-sdk');
var mysql = AWSXRay.captureMySQL(require('mysql'));
var config = { ... };
...
var connection = mysql.createConnection(config);
connection.query('SELECT * FROM cats', function(err, rows) {
//automatically captures query information and error (if any)
});
...
var pool = mysql.createPool(config);
pool.query('SELECT * FROM cats', function(err, rows, fields) {
//automatically captures query information and error (if any)
}, segment);
FAQs
AWS X-Ray SDK for Javascript
The npm package aws-xray-sdk receives a total of 255,117 weekly downloads. As such, aws-xray-sdk popularity was classified as popular.
We found that aws-xray-sdk demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 24 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
A supply chain attack has been detected in versions 1.95.6 and 1.95.7 of the popular @solana/web3.js library.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.