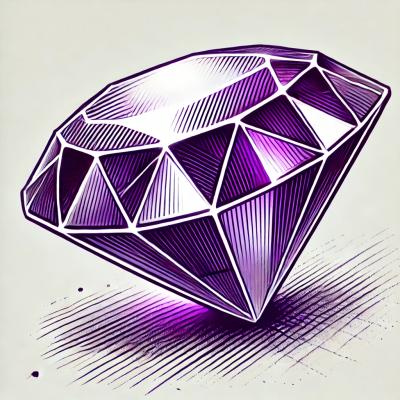
Security News
RubyGems.org Adds New Maintainer Role
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Backbone.js is a lightweight JavaScript library that provides the structure for web applications by offering models with key-value binding and custom events, collections with a rich API of enumerable functions, views with declarative event handling, and connects it all to your existing API over a RESTful JSON interface.
Models
Models in Backbone.js represent the data and the logic of the application. They provide methods for data manipulation and validation.
const Backbone = require('backbone');
const Book = Backbone.Model.extend({
defaults: {
title: 'Unknown',
author: 'Unknown'
}
});
const myBook = new Book({ title: '1984', author: 'George Orwell' });
console.log(myBook.get('title')); // Outputs: 1984
Collections
Collections in Backbone.js are ordered sets of models. They provide a rich set of methods for handling and manipulating groups of models.
const Backbone = require('backbone');
const Book = Backbone.Model.extend({});
const Library = Backbone.Collection.extend({
model: Book
});
const myLibrary = new Library([
{ title: '1984', author: 'George Orwell' },
{ title: 'Brave New World', author: 'Aldous Huxley' }
]);
console.log(myLibrary.length); // Outputs: 2
Views
Views in Backbone.js are responsible for rendering the user interface and handling user interactions. They are associated with a DOM element and a model.
const Backbone = require('backbone');
const BookView = Backbone.View.extend({
tagName: 'li',
render: function() {
this.$el.html(this.model.get('title'));
return this;
}
});
const book = new Backbone.Model({ title: '1984' });
const bookView = new BookView({ model: book });
console.log(bookView.render().el.outerHTML); // Outputs: <li>1984</li>
Routers
Routers in Backbone.js provide methods for routing client-side pages and connecting them to actions and events.
const Backbone = require('backbone');
const AppRouter = Backbone.Router.extend({
routes: {
'books/:id': 'showBook'
},
showBook: function(id) {
console.log('Book ID:', id);
}
});
const router = new AppRouter();
Backbone.history.start();
router.navigate('books/1', { trigger: true }); // Outputs: Book ID: 1
React is a JavaScript library for building user interfaces. It allows developers to create large web applications that can update and render efficiently in response to data changes. Unlike Backbone, React focuses on the view layer and uses a component-based architecture.
Angular is a platform and framework for building single-page client applications using HTML and TypeScript. It provides a comprehensive solution for building dynamic web applications, including data binding, dependency injection, and a powerful templating system. Angular is more opinionated and feature-rich compared to Backbone.
Vue.js is a progressive JavaScript framework for building user interfaces. It is designed to be incrementally adoptable and focuses on the view layer. Vue provides a reactive data binding system and a component-based architecture, similar to React but with a more flexible and less opinionated approach compared to Angular.
____ __ __
/\ _`\ /\ \ /\ \ __
\ \ \ \ \ __ ___\ \ \/'\\ \ \____ ___ ___ __ /\_\ ____
\ \ _ <' /'__`\ /'___\ \ , < \ \ '__`\ / __`\ /' _ `\ /'__`\ \/\ \ /',__\
\ \ \ \ \/\ \ \.\_/\ \__/\ \ \\`\\ \ \ \ \/\ \ \ \/\ \/\ \/\ __/ __ \ \ \/\__, `\
\ \____/\ \__/.\_\ \____\\ \_\ \_\ \_,__/\ \____/\ \_\ \_\ \____\/\_\_\ \ \/\____/
\/___/ \/__/\/_/\/____/ \/_/\/_/\/___/ \/___/ \/_/\/_/\/____/\/_/\ \_\ \/___/
\ \____/
\/___/
(_'_______________________________________________________________________________'_)
(_.———————————————————————————————————————————————————————————————————————————————._)
Backbone supplies structure to JavaScript-heavy applications by providing models key-value binding and custom events, collections with a rich API of enumerable functions, views with declarative event handling, and connects it all to your existing application over a RESTful JSON interface.
For Docs, License, Tests, pre-packed downloads, and everything else, really, see: http://backbonejs.org
To suggest a feature, report a bug, or general discussion: http://github.com/jashkenas/backbone/issues
Backbone is an open-sourced component of DocumentCloud: https://github.com/documentcloud
Many thanks to our contributors: http://github.com/jashkenas/backbone/contributors
Special thanks to Robert Kieffer for the original philosophy behind Backbone. http://github.com/broofa
FAQs
Give your JS App some Backbone with Models, Views, Collections, and Events.
The npm package backbone receives a total of 198,603 weekly downloads. As such, backbone popularity was classified as popular.
We found that backbone demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
RubyGems.org has added a new "maintainer" role that allows for publishing new versions of gems. This new permission type is aimed at improving security for gem owners and the service overall.
Security News
Node.js will be enforcing stricter semver-major PR policies a month before major releases to enhance stability and ensure reliable release candidates.
Security News
Research
Socket's threat research team has detected five malicious npm packages targeting Roblox developers, deploying malware to steal credentials and personal data.