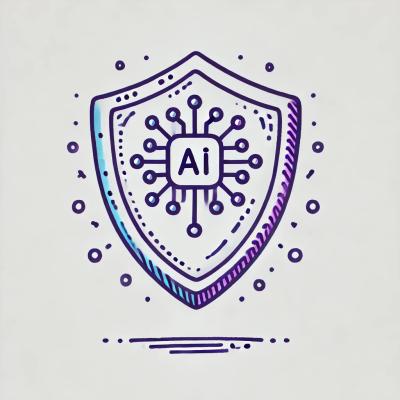
Security News
38% of CISOs Fear They’re Not Moving Fast Enough on AI
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
The 'brcast' npm package is a small library for creating a broadcast system. It allows you to create a broadcaster that can notify listeners of changes. This is particularly useful for managing state changes in applications, especially in the context of React or other component-based frameworks.
Creating a Broadcaster
This feature allows you to create a broadcaster with an initial state. The broadcaster can then be used to manage and notify listeners of state changes.
const createBroadcast = require('brcast');
const broadcast = createBroadcast(initialState);
Subscribing to Changes
This feature allows you to subscribe to state changes. The provided callback function will be called whenever the state changes, allowing you to react to those changes.
const unsubscribe = broadcast.subscribe((state) => {
console.log('State changed:', state);
});
Updating the State
This feature allows you to update the state of the broadcaster. All subscribed listeners will be notified of the new state.
broadcast.setState(newState);
Unsubscribing from Changes
This feature allows you to unsubscribe from state changes, stopping the callback function from being called when the state changes.
unsubscribe();
Redux is a popular state management library for JavaScript applications. It provides a more complex and feature-rich approach to managing state compared to brcast, including middleware support, time-travel debugging, and a strict unidirectional data flow.
MobX is another state management library that focuses on making state management simple and scalable by using observable state and reactions. It provides a more reactive approach compared to brcast, with automatic tracking of state changes.
RxJS is a library for reactive programming using Observables, to make it easier to compose asynchronous or callback-based code. It offers a more powerful and flexible way to handle state changes and asynchronous events compared to brcast.
Tiny data broadcaster with 0 dependencies
The current size of brcast/dist/brcast.umd.min.js
is:
It's like a data store you can subscribe to, with a setter to pump data in. For browsers and node.
This project uses node and npm. Go check them out if you don't have them locally installed.
$ npm install --save brcast
Then with a module bundler like rollup or webpack, use as you would anything else:
// using ES6 modules
import brcast from 'brcast'
// using CommonJS modules
var brcast = require('brcast')
The UMD build is also available on unpkg:
<script src="https://unpkg.com/brcast/dist/brcast.umd.js"></script>
You can find the library on window.brcast
.
import brcast from 'brcast'
let broadcast = brcast()
// subscribe
const subscriptionId = broadcast.subscribe(state => console.log(state))
// setState sets the state and invoke all subscription callbacks passing in the state
broadcast.setState(1)
// setState returns the current state
broadcast.getState()
// unsubscribe to unbind the handler
broadcast.unsubscribe(subscriptionId)
brcast([initialState])
Creates a broadcast
object.
1 - [initialState
] (any): The initial state.
(broadcast
): An object that holds the state.
broadcast.setState(state)
Store the new state.
1 - state
(any): The new state.
Nothing.
broadcast.getState()
Get the stored state.
(Any
): The stored state.
broadcast.subscribe(handler)
Subscribe to state changes.
1 - handler
(Function): The callback to be invoked any time the state changes.
(Number
): The subscription id to be used to unsubscribe.
broadcast.unsubscribe(subscriptionId)
Unsubscribe the change listener.
1 - subscriptionId
(Number): The subscription id returned by subscribing.
Nothing.
$ npm run test
FAQs
Tiny data broadcaster with 0 dependencies
The npm package brcast receives a total of 322,818 weekly downloads. As such, brcast popularity was classified as popular.
We found that brcast demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISOs are racing to adopt AI for cybersecurity, but hurdles in budgets and governance may leave some falling behind in the fight against cyber threats.
Research
Security News
Socket researchers uncovered a backdoored typosquat of BoltDB in the Go ecosystem, exploiting Go Module Proxy caching to persist undetected for years.
Security News
Company News
Socket is joining TC54 to help develop standards for software supply chain security, contributing to the evolution of SBOMs, CycloneDX, and Package URL specifications.