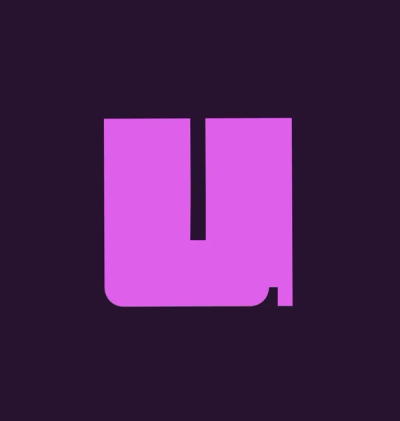
Product
Socket Now Supports uv.lock Files
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
broccoli-babel-transpiler
Advanced tools
A Broccoli plugin which transpile ES6 to readable ES5 by using babel.
The 'broccoli-babel-transpiler' package is a Broccoli plugin that uses Babel to transpile JavaScript files. It allows you to use the latest JavaScript features by converting ES6/ES7+ code into a backward-compatible version of JavaScript that can run in older environments.
Basic Transpilation
This feature allows you to transpile ES6+ JavaScript code to ES5 using Babel. The code sample demonstrates how to set up a basic transpilation process with the '@babel/preset-env' preset.
const Babel = require('broccoli-babel-transpiler');
const inputNode = 'src';
const outputNode = new Babel(inputNode, {
presets: ['@babel/preset-env']
});
module.exports = outputNode;
Custom Plugins
This feature allows you to use custom Babel plugins to transform your code. The code sample shows how to use the '@babel/plugin-transform-arrow-functions' plugin to transform arrow functions into regular functions.
const Babel = require('broccoli-babel-transpiler');
const inputNode = 'src';
const outputNode = new Babel(inputNode, {
plugins: ['@babel/plugin-transform-arrow-functions']
});
module.exports = outputNode;
Source Maps
This feature enables the generation of source maps, which help in debugging by mapping the transpiled code back to the original source code. The code sample demonstrates how to enable inline source maps.
const Babel = require('broccoli-babel-transpiler');
const inputNode = 'src';
const outputNode = new Babel(inputNode, {
sourceMaps: 'inline'
});
module.exports = outputNode;
The 'babel-cli' package provides a command-line interface for Babel. It allows you to transpile files from the command line, making it suitable for simple build scripts and quick transformations. Unlike 'broccoli-babel-transpiler', it is not a Broccoli plugin and does not integrate directly into the Broccoli build pipeline.
The 'gulp-babel' package is a Gulp plugin for Babel. It allows you to integrate Babel transpilation into a Gulp build process. This package is similar to 'broccoli-babel-transpiler' in that it integrates Babel into a build tool, but it is designed specifically for Gulp rather than Broccoli.
Webpack is a module bundler that can also transpile JavaScript using Babel through the 'babel-loader' plugin. It offers a more comprehensive solution for managing and bundling assets, including JavaScript, CSS, and images. While 'broccoli-babel-transpiler' focuses solely on JavaScript transpilation within the Broccoli ecosystem, Webpack provides a more extensive set of features for asset management and optimization.
A Broccoli plugin which transpiles ES6 to readable ES5 by using babel.
$ npm install broccoli-babel-transpiler --save-dev
In your Brocfile.js
:
var esTranspiler = require('broccoli-babel-transpiler');
var scriptTree = esTranspiler(inputTree, options);
You can find options at babel's github repo.
Currently this plugin only supports inline source map. If you need separate source map feature, you're welcome to submit a pull request.
filterExtensions
is an option to limit (or expand) the set of file extensions that will be transformed.
The default filterExtension
is js
var esTranspiler = require('broccoli-babel-transpiler');
var scriptTree = esTranspiler(inputTree, {
filterExtensions:['js', 'es6'] // babelize both .js and .es6 files
});
exportMetadata
is an option that can be used to write a JSON file to the output tree that gives you metadata about the tree's imports and exports.
FAQs
A Broccoli plugin which transpile ES6 to readable ES5 by using babel.
The npm package broccoli-babel-transpiler receives a total of 372,849 weekly downloads. As such, broccoli-babel-transpiler popularity was classified as popular.
We found that broccoli-babel-transpiler demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 8 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports uv.lock files to ensure consistent, secure dependency resolution for Python projects and enhance supply chain security.
Research
Security News
Socket researchers have discovered multiple malicious npm packages targeting Solana private keys, abusing Gmail to exfiltrate the data and drain Solana wallets.
Security News
PEP 770 proposes adding SBOM support to Python packages to improve transparency and catch hidden non-Python dependencies that security tools often miss.