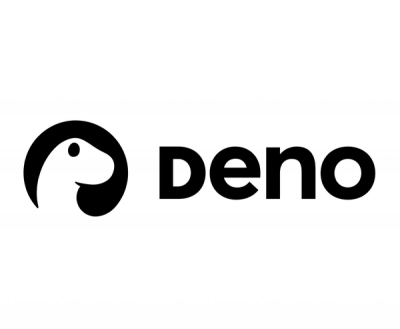
Security News
Deno 2.2 Improves Dependency Management and Expands Node.js Compatibility
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
capacitor-plugin-camera
Advanced tools
A capacitor camera plugin.
For Capacitor 5, use versions 1.x.
For Capacitor 6, use versions 2.x.
npm install capacitor-plugin-camera
npx cap sync
If you are developing a plugin, you can use reflection to get the camera frames as Bitmap or UIImage on the native side.
Java:
Class cls = Class.forName("com.tonyxlh.capacitor.camera.CameraPreviewPlugin");
Method m = cls.getMethod("getBitmap",null);
Bitmap bitmap = (Bitmap) m.invoke(null, null);
Objective-C:
- (UIImage*)getUIImage{
UIImage *image = ((UIImage* (*)(id, SEL))objc_msgSend)(objc_getClass("CameraPreviewPlugin"), sel_registerName("getBitmap"));
return image;
}
You have to call saveFrame
beforehand.
To use camera and microphone, we need to declare permissions.
Add the following to Android's AndroidManifest.xml
:
<uses-permission android:name="android.permission.CAMERA" />
<uses-permission android:name="android.permission.RECORD_AUDIO" />
Add the following to iOS's Info.plist
:
<key>NSCameraUsageDescription</key>
<string>For camera usage</string>
<key>NSMicrophoneUsageDescription</key>
<string>For video recording</string>
Why I cannot see the camera?
For native platforms, the plugin puts the native camera view behind the webview and sets the webview as transparent so that we can display HTML elements above the camera.
You may need to add the style below on your app's HTML or body element to avoid blocking the camera view:
ion-content {
--background: transparent;
}
In dark mode, it is neccessary to set the --ion-blackground-color
property. You can do this with the following code:
document.documentElement.style.setProperty('--ion-background-color', 'transparent');
initialize()
getResolution()
setResolution(...)
getAllCameras()
getSelectedCamera()
selectCamera(...)
setScanRegion(...)
setZoom(...)
setFocus(...)
setDefaultUIElementURL(...)
setElement(...)
startCamera()
stopCamera()
takeSnapshot(...)
saveFrame()
takeSnapshot2(...)
takePhoto(...)
toggleTorch(...)
getOrientation()
startRecording()
stopRecording(...)
setLayout(...)
requestCameraPermission()
requestMicroPhonePermission()
isOpen()
addListener('onPlayed', ...)
addListener('onOrientationChanged', ...)
removeAllListeners()
initialize() => Promise<void>
getResolution() => Promise<{ resolution: string; }>
Returns: Promise<{ resolution: string; }>
setResolution(options: { resolution: number; }) => Promise<void>
Param | Type |
---|---|
options | { resolution: number; } |
getAllCameras() => Promise<{ cameras: string[]; }>
Returns: Promise<{ cameras: string[]; }>
getSelectedCamera() => Promise<{ selectedCamera: string; }>
Returns: Promise<{ selectedCamera: string; }>
selectCamera(options: { cameraID: string; }) => Promise<void>
Param | Type |
---|---|
options | { cameraID: string; } |
setScanRegion(options: { region: ScanRegion; }) => Promise<void>
Param | Type |
---|---|
options | { region: ScanRegion; } |
setZoom(options: { factor: number; }) => Promise<void>
Param | Type |
---|---|
options | { factor: number; } |
setFocus(options: { x: number; y: number; }) => Promise<void>
Param | Type |
---|---|
options | { x: number; y: number; } |
setDefaultUIElementURL(url: string) => Promise<void>
Web Only
Param | Type |
---|---|
url | string |
setElement(ele: any) => Promise<void>
Web Only
Param | Type |
---|---|
ele | any |
startCamera() => Promise<void>
stopCamera() => Promise<void>
takeSnapshot(options: { quality?: number; }) => Promise<{ base64: string; }>
take a snapshot as base64.
Param | Type |
---|---|
options | { quality?: number; } |
Returns: Promise<{ base64: string; }>
saveFrame() => Promise<{ success: boolean; }>
save a frame internally. Android and iOS only.
Returns: Promise<{ success: boolean; }>
takeSnapshot2(options: { canvas: HTMLCanvasElement; maxLength?: number; }) => Promise<{ scaleRatio?: number; }>
take a snapshot on to a canvas. Web Only
Param | Type |
---|---|
options | { canvas: any; maxLength?: number; } |
Returns: Promise<{ scaleRatio?: number; }>
takePhoto(options: { pathToSave?: string; includeBase64?: boolean; }) => Promise<{ path?: string; base64?: string; blob?: Blob; }>
Param | Type |
---|---|
options | { pathToSave?: string; includeBase64?: boolean; } |
Returns: Promise<{ path?: string; base64?: string; blob?: any; }>
toggleTorch(options: { on: boolean; }) => Promise<void>
Param | Type |
---|---|
options | { on: boolean; } |
getOrientation() => Promise<{ "orientation": "PORTRAIT" | "LANDSCAPE"; }>
get the orientation of the device.
Returns: Promise<{ orientation: 'PORTRAIT' | 'LANDSCAPE'; }>
startRecording() => Promise<void>
stopRecording(options: { includeBase64?: boolean; }) => Promise<{ path?: string; base64?: string; blob?: Blob; }>
Param | Type |
---|---|
options | { includeBase64?: boolean; } |
Returns: Promise<{ path?: string; base64?: string; blob?: any; }>
setLayout(options: { top: string; left: string; width: string; height: string; }) => Promise<void>
Param | Type |
---|---|
options | { top: string; left: string; width: string; height: string; } |
requestCameraPermission() => Promise<void>
requestMicroPhonePermission() => Promise<void>
isOpen() => Promise<{ isOpen: boolean; }>
Returns: Promise<{ isOpen: boolean; }>
addListener(eventName: 'onPlayed', listenerFunc: onPlayedListener) => Promise<PluginListenerHandle>
Param | Type |
---|---|
eventName | 'onPlayed' |
listenerFunc | onPlayedListener |
Returns: Promise<PluginListenerHandle>
addListener(eventName: 'onOrientationChanged', listenerFunc: onOrientationChangedListener) => Promise<PluginListenerHandle>
Param | Type |
---|---|
eventName | 'onOrientationChanged' |
listenerFunc | onOrientationChangedListener |
Returns: Promise<PluginListenerHandle>
removeAllListeners() => Promise<void>
measuredByPercentage: 0 in pixel, 1 in percent
Prop | Type |
---|---|
left | number |
top | number |
right | number |
bottom | number |
measuredByPercentage | number |
Prop | Type |
---|---|
remove | () => Promise<void> |
(result: { resolution: string; }): void
(): void
FAQs
A capacitor camera plugin
The npm package capacitor-plugin-camera receives a total of 82 weekly downloads. As such, capacitor-plugin-camera popularity was classified as not popular.
We found that capacitor-plugin-camera demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Deno 2.2 enhances Node.js compatibility, improves dependency management, adds OpenTelemetry support, and expands linting and task automation for developers.
Security News
React's CRA deprecation announcement sparked community criticism over framework recommendations, leading to quick updates acknowledging build tools like Vite as valid alternatives.
Security News
Ransomware payment rates hit an all-time low in 2024 as law enforcement crackdowns, stronger defenses, and shifting policies make attacks riskier and less profitable.