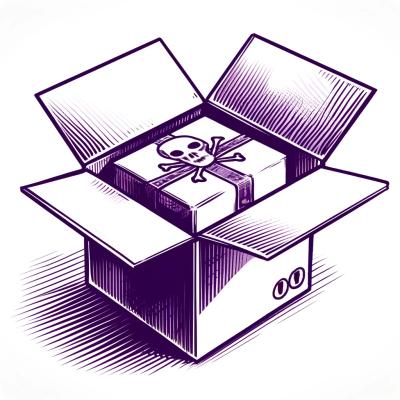
Security News
Research
Data Theft Repackaged: A Case Study in Malicious Wrapper Packages on npm
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
check-more-types
Advanced tools
Additional type checks for https://github.com/philbooth/check-types.js
The check-more-types npm package provides a variety of type checking and assertion functions that enhance JavaScript's type testing capabilities. It offers a wide range of predicates for more precise type validation, making it easier to enforce type safety in JavaScript applications.
Primitive type checks
This feature allows for checking JavaScript's primitive types such as strings, numbers, and booleans. It's useful for basic type validation.
const check = require('check-more-types');
// checks if a value is a string
check.string('hello'); // true
// checks if a value is a number
check.number(42); // true
Complex type checks
This feature extends the library's capabilities to more complex types, such as arrays of specific types or objects matching a schema. It's particularly useful for validating data structures.
const check = require('check-more-types');
// checks if a value is an array of strings
check.arrayOfStrings(['hello', 'world']); // true
// checks if a value is an object with specific properties
check.schema({
name: check.string,
age: check.number
})({ name: 'John', age: 30 }); // true
Custom predicates
check-more-types allows for the creation of custom predicates, enhancing its flexibility and allowing users to define their own specific type checks.
const check = require('check-more-types');
// defines a custom predicate for checking if a number is even
check.mixin({
even: function (n) { return check.number(n) && n % 2 === 0; }
});
// uses the custom predicate
check.even(42); // true
Joi is a powerful schema description language and data validator for JavaScript. Compared to check-more-types, Joi offers a more comprehensive API for defining complex validation schemas, making it better suited for validating nested objects and complex data structures.
Prop-types is a library for type checking React component props. While check-more-types is more general-purpose, prop-types is specifically designed for React applications, offering a straightforward way to ensure components receive props of the correct type.
Validator is a library that provides string validation and sanitization. Unlike check-more-types, which offers a broad range of type checks, Validator focuses on string validation, offering functions for format validation, sanitization, and more.
Additional type checks for check-types.js
See Readable conditions for advice and examples.
node: npm install check-more-types --save
global.check = require('check-types');
require('check-more-types');
// patches global check object
browser bower install check-more-types --save
<script src="check-types.js"></script>
<script src="check-more-types.js"></script>
check.defined(0); // true
check.defined(1); // true
check.defined(true); // true
check.defined(false); // true
check.defined(null); // true
check.defined(''); // true
check.defined(); // false
check.defined(root.doesNotExist); // false
check.defined({}.doesNotExist); // false
check.bit(0); // true
check.bit(1); // true
check.bit('1'); // false
check.bit(2); // false
check.bit(true); // false
var foo = {},
bar = {};
check.same(foo, foo); // true
check.same(foo, bar); // false
// primitives are compared by value
check.same(0, 0); // true
check.same('foo', 'foo'); // true
check.same
should produce same result as ===
.
check.unit(0); // true
check.unit(1); // true
check.unit(0.1); // true
check.unit(1.2); // false
check.unit(-0.1); // false
check.hexRgb('#FF00FF'); // true
check.hexRgb('#000'); // true
check.hexRgb('#aaffed'); // true
check.hexRgb('#00aaffed'); // false
check.hexRgb('aaffed'); // false
check.bool(true); // true
check.bool(false); // true
check.bool(0); // false
check.bool(1); // false
check.bool('1'); // false
check.bool(2); // false
check.empty([]); // true
check.empty(''); // true
check.empty({}); // true
check.empty(0); // false
check.empty(['foo']); // false
check.unempty([]); // false
check.unempty(''); // false
check.unempty({}); // false
check.unempty(0); // true
check.unempty(['foo']); // true
check.unempty('foo'); // true
check.unemptyArray(null); // false
check.unemptyArray(1); // false
check.unemptyArray({}); // false
check.unemptyArray([]); // false
check.unemptyArray(root.doesNotExist); // false
check.unemptyArray([1]); // true
check.unemptyArray(['foo', 'bar']); // true
// second argument is checkLowerCase
check.arrayOfStrings(['foo', 'Foo']); // true
check.arrayOfStrings(['foo', 'Foo'], true); // false
check.arrayOfStrings(['foo', 'bar'], true); // true
check.arrayOfStrings(['FOO', 'BAR'], true); // false
// second argument is checkLowerCase
check.arrayOfArraysOfStrings([['foo'], ['bar'}}); // true
check.arrayOfArraysOfStrings([['foo'], ['bar'}}, true); // true
check.arrayOfArraysOfStrings([['foo'], ['BAR'}}, true); // false
check.lowerCase('foo bar'); // true
check.lowerCase('*foo ^bar'); // true
check.lowerCase('fooBar'); // false
// non-strings return false
check.lowerCase(10); // false
var obj = {
foo: 'foo',
bar: 0
};
check.has(obj, 'foo'); // true
check.has(obj, 'bar'); // true
check.has(obj, 'baz'); // false
// non-object returns false
check.has(5, 'foo'); // false
check.has('foo', 'length'); // true
var obj = {
foo: 'foo',
bar: 'bar',
baz: 'baz'
};
var predicates = {
foo: check.unemptyString,
bar: function(value) {
return value === 'bar';
}
};
check.all(obj, predicates); // true
var obj = {
foo: 'foo',
bar: 'bar',
baz: 'baz'
};
var schema = {
foo: check.unemptyString,
bar: function(value) {
return value === 'bar';
}
};
check.schema(schema, obj); // true
check.schema(schema, {}); // false
check.spec
is equivalent to check.all
but with arguments reversed.
This makes it very convenient to create new validator functions using partial
argument application
var personSchema = {
name: check.unemptyString,
age: check.positiveNumber
};
var isValidPerson = check.schema.bind(null, personSchema);
var h1 = {
name: 'joe',
age: 10
};
var h2 = {
name: 'ann'
// missing age property
};
isValidPerson(h1); // true
isValidPerson(h2); // false
Because bound schema parameter generates a valid function, you can nest checks using
schema composition. For example let us combine the reuse isValidPerson
as part of
another check
var teamSchema = {
manager: isValidPerson,
members: check.unemptyArray
};
var team = {
manager: {
name: 'jim',
age: 20
},
members: ['joe', 'ann']
};
check.schema(teamSchema, team); // true
function foo() {
throw new Error('foo');
}
function bar() {}
function isValidError(err) {
return err.message === 'foo';
}
function isInvalid(err) {
check.instance(err, Error); // true
return false;
}
check.raises(foo); // true
check.raises(bar); // false
check.raises(foo, isValidError); // true
check.raises(foo, isInvalid); // false
Every predicate function is also added to check.maybe
object.
The maybe
predicate passes if the argument is null or undefined,
or the predicate returns true.
check.maybe.bool(); // true
check.maybe.bool('true'); // false
var empty;
check.maybe.lowerCase(empty); // true
check.maybe.unemptyArray(); // true
check.maybe.unemptyArray([]); // false
check.maybe.unemptyArray(['foo', 'bar']); // true
Every function has a negated predicate in check.not
object
check.not.bool(4); // true
check.not.bool('true'); // true
check.not.bool(true); // false
Every predicate can also throw an exception if it fails
check.verify.arrayOfStrings(['foo', 'bar']);
check.verify.bit(1);
function nonStrings() {
check.verify.arrayOfStrings(['Foo', 1]);
}
check.raises(nonStrings); // true
function nonLowerCase() {
check.verify.lowerCase('Foo');
}
check.raises(nonLowerCase); // true
You can add new predicates to check
, check.maybe
, etc. by using check.mixin(predicate)
method
function isBar(a) {
return a === 'bar';
}
check.mixin(isBar, 'bar');
check.bar('bar'); // true
check.bar('anything else'); // false
// supports modifiers
check.maybe.bar(); // true
check.maybe.bar('bar'); // true
check.not.bar('foo'); // true
check.not.bar('bar'); // false
Mixin will not override existing functions
function isFoo(a) {
return a === 'foo';
}
function isBar(a) {
return a === 'bar';
}
check.mixin(isFoo, 'isFoo');
check.isFoo; // isFoo
check.mixin(isBar, 'isFoo');
check.isFoo; // isFoo
Using check-more-types you can separate the inner function logic from checking input arguments. Instead of this
function add(a, b) {
la(check.number(a), 'first argument should be a number', a);
la(check.number(a), 'second argument should be a number', b);
return a + b;
}
you can use check.defend
function
function add(a, b) {
return a + b;
}
var safeAdd = check.defend(add, check.number, check.number);
add('foo', 2); // 'foo2'
// calling safeAdd('foo', 2) raises an exception
check.raises(safeAdd.bind(null, 'foo', 2)); // true
function add(a, b) {
if (typeof b === 'undefined') {
return 'foo';
}
return a + b;
}
add(2); // 'foo'
var safeAdd = check.defend(add, check.number, check.maybe.number);
safeAdd(2, 3); // 5
safeAdd(2); // 'foo'
This works great when combined with JavaScript module pattern as in this example
var add = (function() {
// inner private function without any argument checks
function add(a, b) {
return a + b;
}
// return defended function
return check.defend(add, check.number, check.number);
}());
add(2, 3); // 5
// trying to call with non-numbers raises an exception
function callAddWithNonNumbers() {
return add('foo', 'bar');
}
check.raises(callAddWithNonNumbers); // true
Author: Kensho © 2014
Support: if you find any problems with this library, open issue on Github
This documentation was generated using grunt-xplain and grunt-readme.
The MIT License (MIT)
Copyright (c) 2014 Kensho
Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated documentation files (the "Software"), to deal in the Software without restriction, including without limitation the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software, and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
The above copyright notice and this permission notice shall be included in all copies or substantial portions of the Software.
THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE SOFTWARE.
FAQs
Large collection of predicates.
The npm package check-more-types receives a total of 3,490,447 weekly downloads. As such, check-more-types popularity was classified as popular.
We found that check-more-types demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Research
The Socket Research Team breaks down a malicious wrapper package that uses obfuscation to harvest credentials and exfiltrate sensitive data.
Research
Security News
Attackers used a malicious npm package typosquatting a popular ESLint plugin to steal sensitive data, execute commands, and exploit developer systems.
Security News
The Ultralytics' PyPI Package was compromised four times in one weekend through GitHub Actions cache poisoning and failure to rotate previously compromised API tokens.