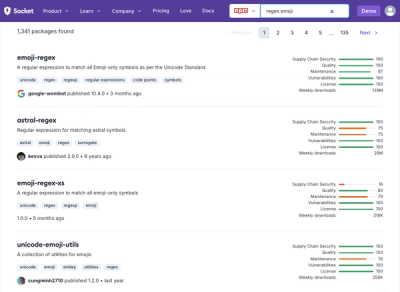
Security News
Weekly Downloads Now Available in npm Package Search Results
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
cleave.js
Advanced tools
Cleave.js is a JavaScript library that helps you format input fields in real-time. It provides a simple way to format various types of input fields such as credit card numbers, phone numbers, dates, and more.
Credit Card Number Formatting
This feature automatically formats credit card numbers as the user types. It adds spaces between every four digits for better readability.
new Cleave('.input-credit-card', { creditCard: true });
Phone Number Formatting
This feature formats phone numbers according to the specified region code. In this example, it formats phone numbers in the US format.
new Cleave('.input-phone', { phone: true, phoneRegionCode: 'US' });
Date Formatting
This feature formats date inputs according to the specified pattern. In this example, it formats the date in the 'YYYY-MM-DD' format.
new Cleave('.input-date', { date: true, datePattern: ['Y', 'm', 'd'] });
Numeral Formatting
This feature formats numerical inputs with thousand separators. It makes large numbers more readable by adding commas.
new Cleave('.input-numeral', { numeral: true, numeralThousandsGroupStyle: 'thousand' });
Custom Delimiter
This feature allows custom delimiters and block sizes for input formatting. In this example, it formats the input with dashes every four characters.
new Cleave('.input-custom', { delimiter: '-', blocks: [4, 4, 4, 4] });
Inputmask is a JavaScript library that creates input masks for various types of input fields. It is highly configurable and supports a wide range of input types. Compared to Cleave.js, Inputmask offers more customization options but may require more configuration.
Vanilla Text Mask is a library for creating input masks in vanilla JavaScript. It is lightweight and easy to use, similar to Cleave.js. However, it may not offer as many built-in formatting options as Cleave.js.
IMask is a versatile input masking library that supports a wide range of input types and custom masks. It is similar to Cleave.js in terms of functionality but offers more advanced features like dynamic masks and pattern matching.
Cleave.js has a simple purpose: to help you format input text content automatically.
TL;DR the demo page
The idea is to provide an easy way to increase input field readability by formatting your typed data. By using this library, you won't need to write any mind-blowing regular expressions or mask patterns to format input text.
However, this isn't meant to replace any validation or mask library, you should still sanitize and validate your data in backend.
npm install --save cleave.js
Grab file from dist directory
Simply include
<script src="cleave.min.js"></script>
<script src="cleave-phone.{country}.js"></script>
cleave-phone.{country}.js
addon is only required when phone shortcut mode is enabled. See more in documentation: phone lib addon section
Then have a text field
<input class="input-phone" type="text"/>
Now in your JavaScript
var cleave = new Cleave('.input-element', {
phone: true,
phoneRegionCode: '{country}'
});
.input-element
here is a unique DOM element. If you want to apply Cleave for multiple elements, you need to give different css selectors and apply to each of them
More examples: the demo page
var Cleave = require('cleave.js');
require('cleave.js/dist/addons/cleave-phone.{country}');
var cleave = new Cleave(...)
require(['cleave.js/dist/cleave.min', 'cleave.js/dist/addons/cleave-phone.{country}'], function (Cleave) {
var cleave = new Cleave(...)
});
import React from 'react';
import ReactDOM from 'react-dom';
import Cleave from 'cleave.js/react';
Then in JSX:
class MyComponent extends React.Component {
constructor(props, context) {
super(props, context);
this.onCreditCardChange = this.onCreditCardChange.bind(this);
this.onCreditCardFocus = this.onCreditCardFocus.bind(this);
}
onCreditCardChange(event) {
// formatted pretty value
console.log(event.target.value);
// raw value
console.log(event.target.rawValue);
}
onCreditCardFocus(event) {
// update some state
}
render() {
return (
<Cleave placeholder="Enter your credit card number"
options={{creditCard: true}}
onFocus={this.onCreditCardFocus}
onChange={this.onCreditCardChange} />
);
}
}
As you can see, here you simply use <Cleave/>
as a normal <input/>
field
<input/>
attributesoptions
proponChange
event listenerAdvanced usage:
Usage for Webpack
, Browserify
and more in documentation: ReactJS component usage
First include the directive module:
<script src="cleave.js/dist/cleave-angular.min.js"></script>
<script src="cleave.js/dist/addons/cleave-phone.{country}.js"></script>
And in your model:
angular.module('app', ['cleave.js'])
.controller('AppController', function($scope) {
$scope.onCreditCardTypeChanged = function(type) {
$scope.model.creditCardType = type;
};
$scope.model = {
rawValue: ''
};
$scope.options = {
creditCard: {
creditCard: true,
onCreditCardTypeChanged: $scope.onCreditCardTypeChanged
}
};
});
Then easily you can apply cleave
directive to input
field:
<div ng-controller="AppController">
<input ng-model="model.rawValue" ng-whatever="..." type="text" placeholder="Enter credit card number"
cleave="options.creditCard"/>
</div>
More usage in documentation: Angular directive usage
npm install
Build assets
gulp build
Run tests
gulp test
Lint
gulp eslint
Publish (build, tests & lint)
gulp publish
For contributors, please run
gulp publish
to ensure your PR passes tests and lint, also we have a not in the plan list you may concern.
Cleave.js is licensed under the Apache License Version 2.0
FAQs
JavaScript library for formatting input text content when you are typing
The npm package cleave.js receives a total of 200,776 weekly downloads. As such, cleave.js popularity was classified as popular.
We found that cleave.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.
Security News
A Stanford study reveals 9.5% of engineers contribute almost nothing, costing tech $90B annually, with remote work fueling the rise of "ghost engineers."
Research
Security News
Socket’s threat research team has detected six malicious npm packages typosquatting popular libraries to insert SSH backdoors.