colorful-chalk-logger
is a colorful logger tool based on chalk(so you can use a lot of colors) and commander(so you can use command line parameters to customized the logger's behavior).

Install
you can use colorful-chalk-logger
either in typescript
or javascript
.
yarn add colorful-chalk-logger
// or
npm install --save colorful-chalk-logger
cli-options
--log-level <debug|verbose|info|warn|error|fatal>
: specify global logger level.--log-flag <[no-](date|inline|colorful)>
: the prefix no-
represent negation.
date
: whether to print date. default value is falseinline
: each log record output in one line. default value is false.colorful
: whether to print with colors. default value is true.
--log-output <filepath>
: specify the output path (default behavior is output directory to stdout).
- suggest: set
colorful = false
and inline = true
if you want to output logs into a file.
--log-encoding <encoding>
: specify the log file's encoding.
Example
import { ColorfulChalkLogger, ERROR } from 'colorful-chalk-logger'
const logger = new ColorfulChalkLogger('demo', {
level: ERROR,
date: false,
colorful: true,
}, process.argv)
logger.debug('A', 'B', 'C')
logger.verbose('A', 'B', 'C')
logger.info('a', 'b', 'c')
logger.warn('X', 'Y', 'Z', { a: 1, b: 2})
logger.error('x', 'y', 'z', { c: { a: 'hello' }, b: { d: 'world' } })
logger.fatal('1', '2', '3')
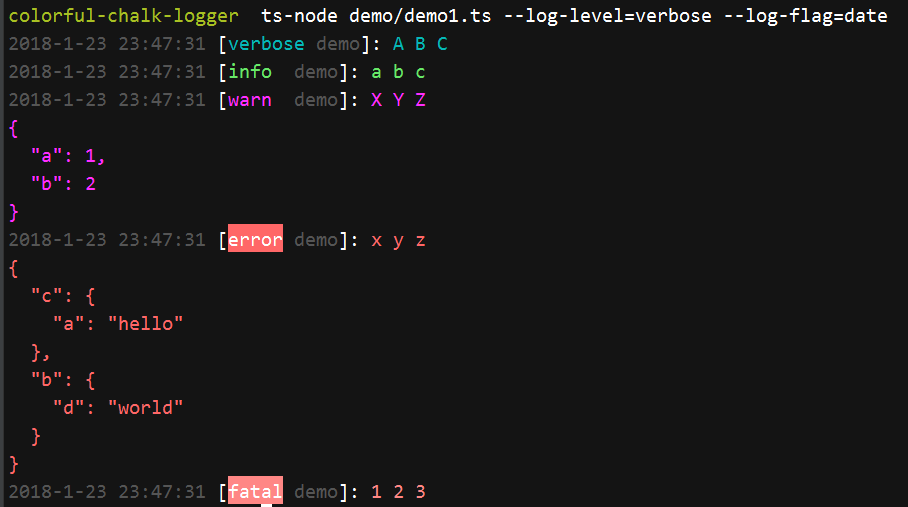
Custom output format:
import chalk from 'chalk'
import { ColorfulChalkLogger, ERROR, Level } from 'colorful-chalk-logger'
let logger = new ColorfulChalkLogger('demo', {
level: ERROR,
date: false,
colorful: true,
}, process.argv)
logger.formatHeader = function (level: Level, date: Date): string {
let { desc } = level
let { name } = this
if( this.flags.colorful ) {
desc = level.headerChalk.fg(desc)
if (level.headerChalk.bg != null) desc = level.headerChalk.bg(desc)
name = chalk.gray(name)
}
let header = `${desc} ${name}`
if( !this.flags.date) return `[${header}]`
let dateString = date.toLocaleTimeString()
if( this.flags.colorful ) dateString = chalk.gray(dateString)
return `<${dateString} ${header}>`
}
logger.debug('A', 'B', 'C')
logger.verbose('A', 'B', 'C')
logger.info('a', 'b', 'c')
logger.warn('X', 'Y', 'Z', { a: 1, b: 2})
logger.error('x', 'y', 'z', { c: { a: 'hello' }, b: { d: 'world' } })
logger.fatal('1', '2', '3')
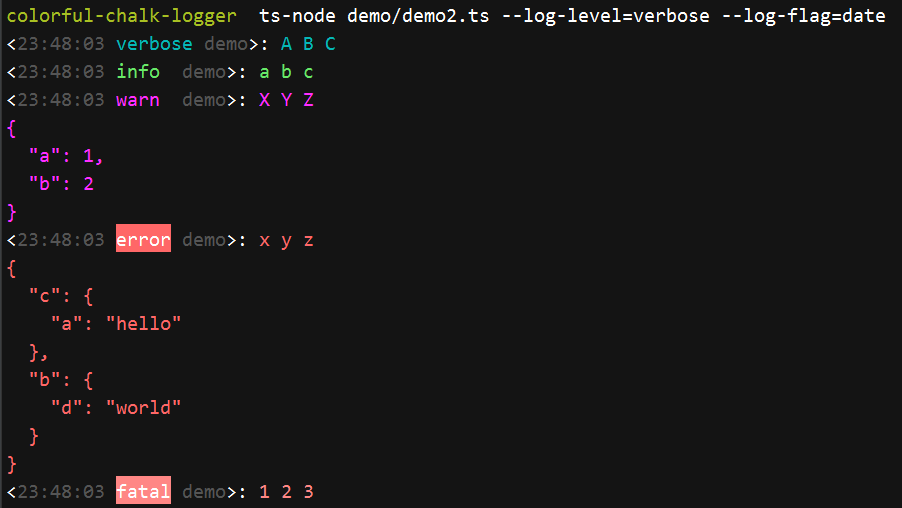
import chalk from 'chalk'
import { ColorfulChalkLogger, ERROR } from 'colorful-chalk-logger'
let logger = new ColorfulChalkLogger('demo', {
level: ERROR,
date: false,
colorful: true,
dateChalk: 'green',
nameChalk: chalk.cyan.bind(chalk),
}, process.argv)
logger.debug('A', 'B', 'C')
logger.verbose('A', 'B', 'C')
logger.info('a', 'b', 'c')
logger.warn('X', 'Y', 'Z', { a: 1, b: 2})
logger.error('x', 'y', 'z', { c: { a: 'hello' }, b: { d: 'world' } })
logger.fatal('1', '2', '3')
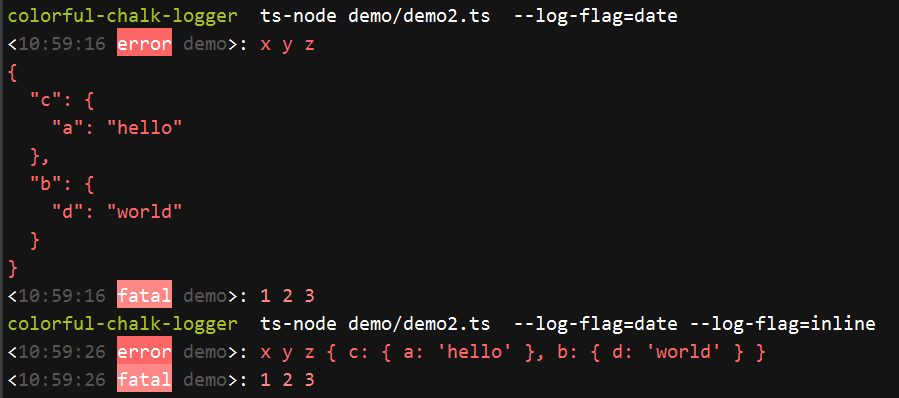
output to file
import path from 'path'
import chalk from 'chalk'
import { ColorfulChalkLogger, DEBUG } from 'colorful-chalk-logger'
let logger = new ColorfulChalkLogger('demo', {
level: DEBUG,
date: true,
inline: true,
colorful: false,
dateChalk: 'green',
nameChalk: chalk.cyan.bind(chalk),
filepath: path.resolve(__dirname, 'orz.log'),
encoding: 'utf-8',
}, process.argv)
logger.debug('A', 'B', 'C')
logger.verbose('A', 'B', 'C')
logger.info('a', 'b', 'c')
logger.warn('X', 'Y', 'Z', { a: 1, b: 2})
logger.error('x', 'y', 'z', { c: { a: 'hello' }, b: { d: 'world' } })
logger.fatal('1', '2', '3')
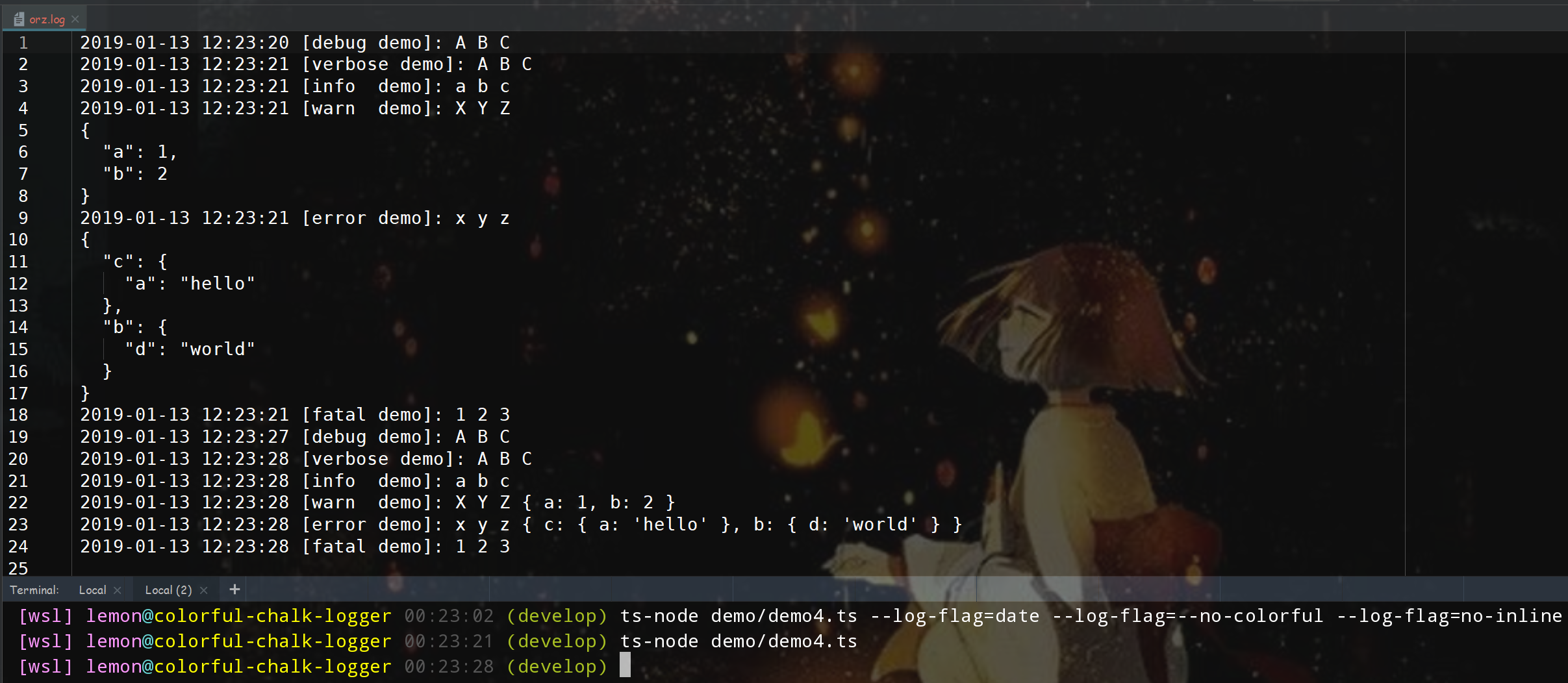
register to commander
import chalk from 'chalk'
import commander from 'Commander'
import { ColorfulChalkLogger, ERROR } from '../src'
let logger = new ColorfulChalkLogger('demo', {
level: ERROR,
date: false,
colorful: true,
dateChalk: 'green',
nameChalk: chalk.cyan.bind(chalk),
}, process.argv)
commander
.version('v1.0.0')
.arguments('[orz]')
logger.registerToCommander(commander)
commander
.option('-e, --encoding <encoding>', 'specified <filepath>\'s encoding')
.parse(process.argv)
logger.debug('A', 'B', 'C')
logger.verbose('A', 'B', 'C')
logger.info('a', 'b', 'c')
logger.warn('X', 'Y', 'Z', { a: 1, b: 2})
logger.error('x', 'y', 'z', { c: { a: 'hello' }, b: { d: 'world' } })
logger.fatal('1', '2', '3')
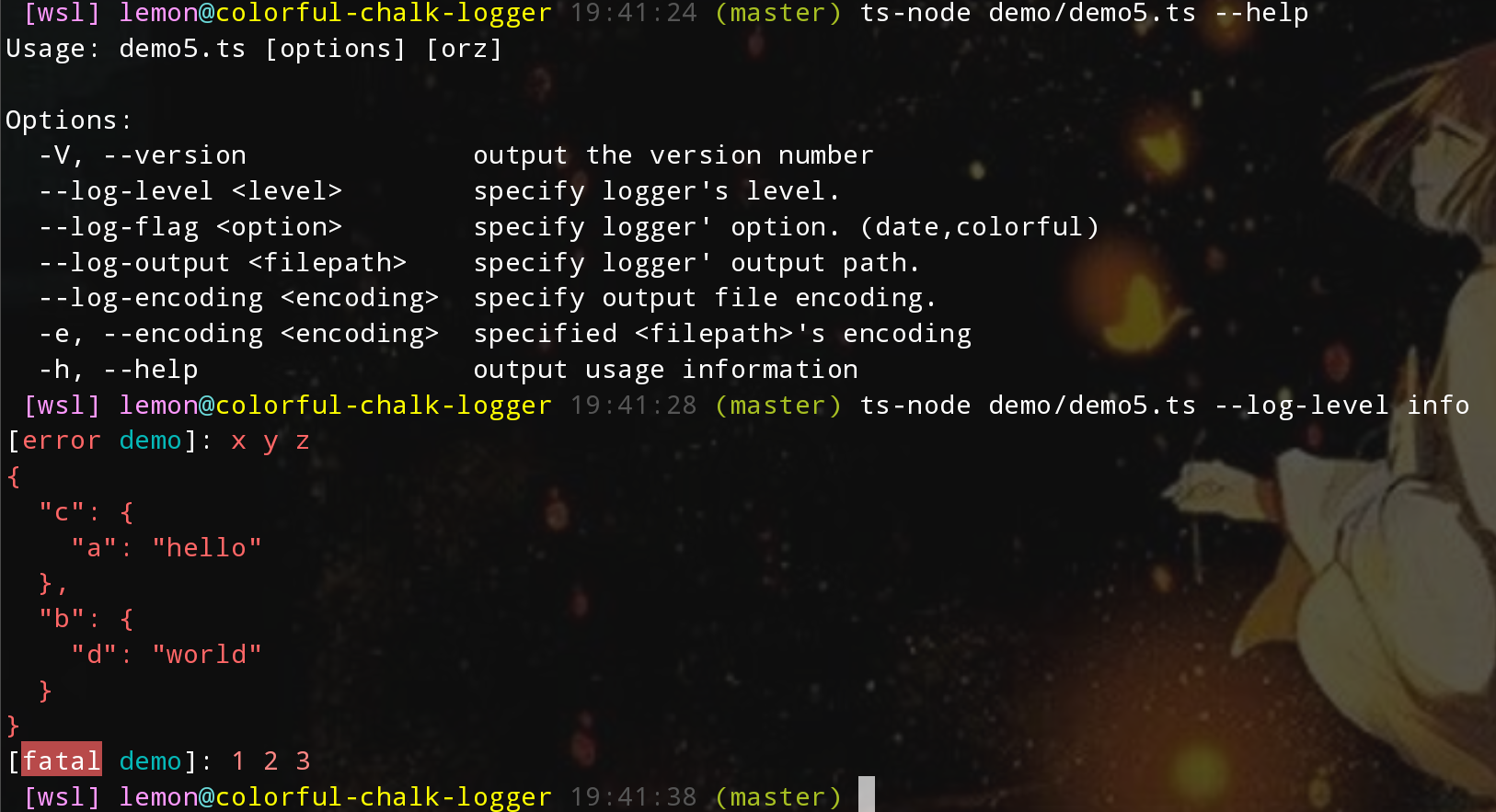
string format
import { ColorfulChalkLogger, DEBUG } from '../src'
let logger = new ColorfulChalkLogger('demo', {
level: DEBUG,
date: true,
colorful: true,
inline: true,
}, process.argv)
logger.verbose('user({})', { username: 'lemon-clown', avatar: 'https://avatars0.githubusercontent.com/u/42513619?s=400&u=d878f4532bb5749979e18f3696b8985b90e9f78b&v=4' })
logger.error('bad argument ({}). error({})', { username: 123 }, new Error('username is invalid'))
let logger2 = new ColorfulChalkLogger('demo', {
level: DEBUG,
date: true,
colorful: true,
inline: true,
placeholderRegex: /(?<!\\)\<\>/g
}, process.argv)
logger2.verbose('user(<>)', { username: 'lemon-clown', avatar: 'https://avatars0.githubusercontent.com/u/42513619?s=400&u=d878f4532bb5749979e18f3696b8985b90e9f78b&v=4' })
logger2.error('bad argument (<>). error({})', { username: 123 }, new Error('username is invalid'))
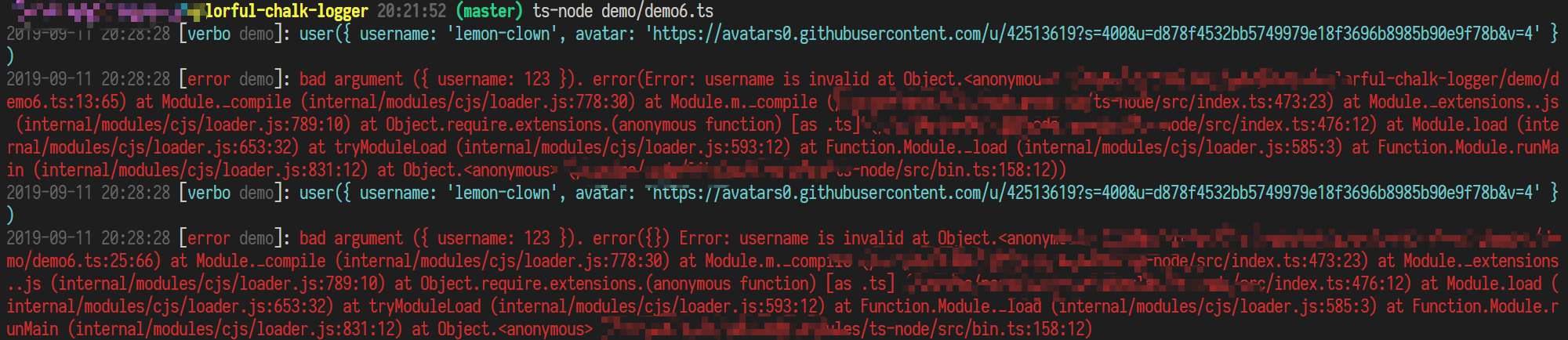