What is command-line-args?
The command-line-args npm package is a library for parsing command-line arguments in Node.js applications. It allows developers to define options and parse them from the command line, making it easier to handle user inputs and configurations.
What are command-line-args's main functionalities?
Basic Argument Parsing
This feature allows you to define and parse basic command-line arguments. In this example, the 'file' option expects a string value, and the 'verbose' option is a boolean flag.
const commandLineArgs = require('command-line-args');
const optionDefinitions = [
{ name: 'file', type: String },
{ name: 'verbose', type: Boolean }
];
const options = commandLineArgs(optionDefinitions);
console.log(options);
Default Values
This feature allows you to set default values for options. If the user does not provide a value for 'file' or 'verbose', the defaults 'default.txt' and 'false' will be used, respectively.
const commandLineArgs = require('command-line-args');
const optionDefinitions = [
{ name: 'file', type: String, defaultValue: 'default.txt' },
{ name: 'verbose', type: Boolean, defaultValue: false }
];
const options = commandLineArgs(optionDefinitions);
console.log(options);
Multiple Values
This feature allows you to handle multiple values for a single option. In this example, the 'files' option can accept multiple string values.
const commandLineArgs = require('command-line-args');
const optionDefinitions = [
{ name: 'files', type: String, multiple: true, defaultOption: true }
];
const options = commandLineArgs(optionDefinitions);
console.log(options);
Aliases
This feature allows you to define aliases for options. In this example, 'help' can be accessed with '-h' and 'version' with '-v'.
const commandLineArgs = require('command-line-args');
const optionDefinitions = [
{ name: 'help', alias: 'h', type: Boolean },
{ name: 'version', alias: 'v', type: Boolean }
];
const options = commandLineArgs(optionDefinitions);
console.log(options);
Other packages similar to command-line-args
yargs
Yargs is a popular library for parsing command-line arguments in Node.js. It provides a rich set of features, including command handling, argument validation, and automatic help generation. Compared to command-line-args, yargs offers more advanced features and a more user-friendly API.
commander
Commander is another widely-used library for building command-line interfaces in Node.js. It supports option parsing, command definitions, and automatic help generation. Commander is known for its simplicity and ease of use, making it a good alternative to command-line-args for simpler use cases.
minimist
Minimist is a lightweight library for parsing command-line arguments. It provides basic functionality for handling options and arguments, but lacks some of the advanced features found in command-line-args, yargs, and commander. Minimist is a good choice for projects that require minimal overhead.

Upgraders, please read the release notes
command-line-args
A mature, feature-complete library to parse command-line options.
Synopsis
You can set options using the main notation standards (learn more). These commands are all equivalent, setting the same values:
$ example --verbose --timeout=1000 --src one.js --src two.js
$ example --verbose --timeout 1000 --src one.js two.js
$ example -vt 1000 --src one.js two.js
$ example -vt 1000 one.js two.js
To access the values, first create a list of option definitions describing the options your application accepts. The type
property is a setter function (the value supplied is passed through this), giving you full control over the value received.
const optionDefinitions = [
{ name: 'verbose', alias: 'v', type: Boolean },
{ name: 'src', type: String, multiple: true, defaultOption: true },
{ name: 'timeout', alias: 't', type: Number }
]
Next, parse the options using commandLineArgs():
const commandLineArgs = require('command-line-args')
const options = commandLineArgs(optionDefinitions)
options
now looks like this:
{
src: [
'one.js',
'two.js'
],
verbose: true,
timeout: 1000
}
Advanced usage
Beside the above typical usage, you can configure command-line-args to accept more advanced syntax forms.
-
Command-based syntax (git style) in the form:
$ executable <command> [options]
For example.
$ git commit --squash -m "This is my commit message"
-
Command and sub-command syntax (docker style) in the form:
$ executable <command> [options] <sub-command> [options]
For example.
$ docker run --detached --image centos bash -c yum install -y httpd
Usage guide generation
A usage guide (typically printed when --help
is set) can be generated using command-line-usage. See the examples below and read the documentation for instructions how to create them.
A typical usage guide example.
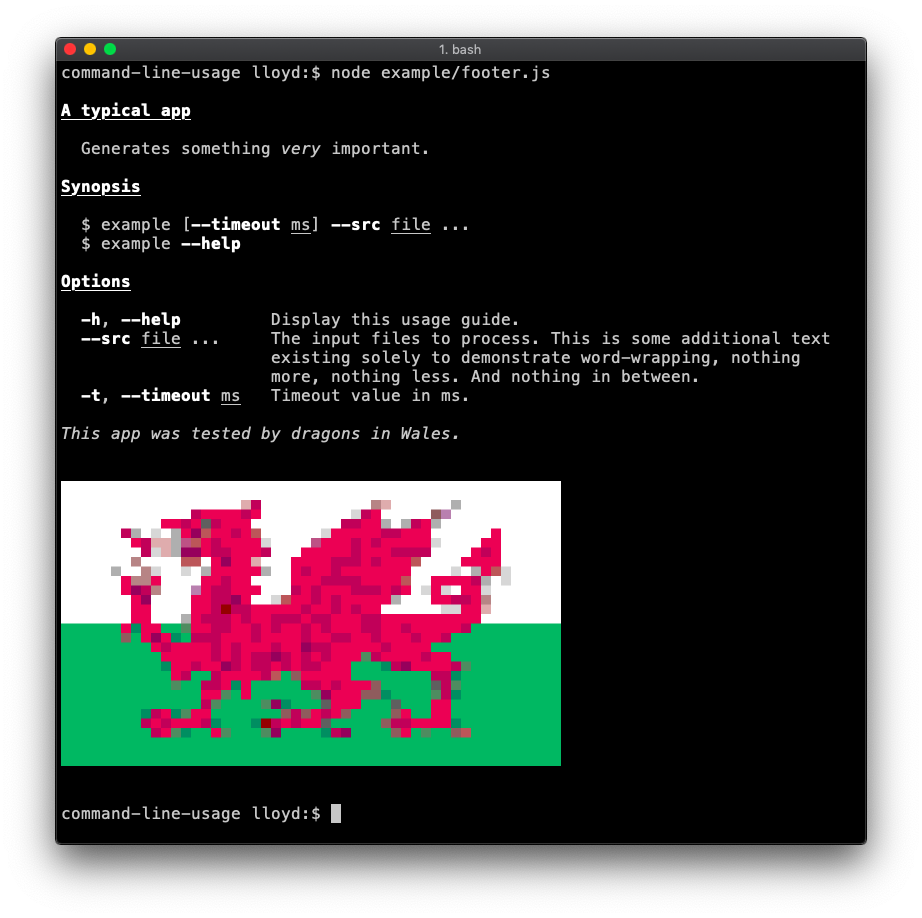
The polymer-cli usage guide is a good real-life example.
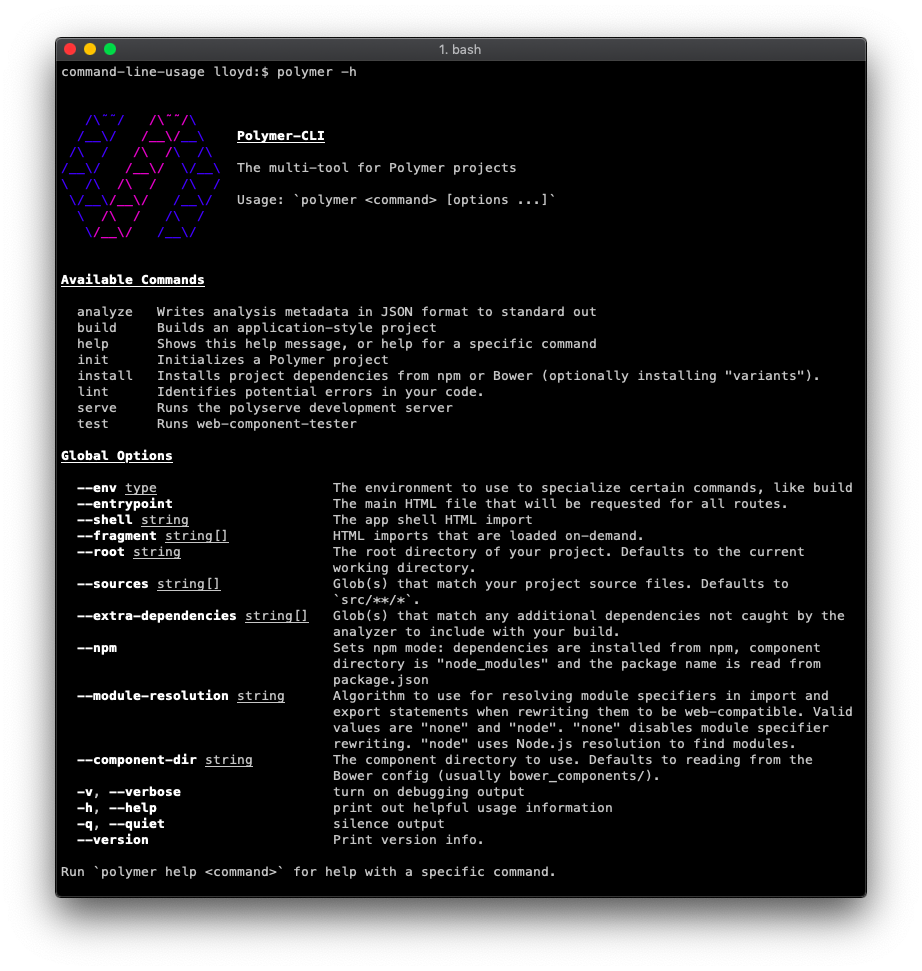
Further Reading
There is plenty more to learn, please see the wiki for examples and documentation.
Install
$ npm install command-line-args --save
© 2014-21 Lloyd Brookes <75pound@gmail.com>. Documented by jsdoc-to-markdown.