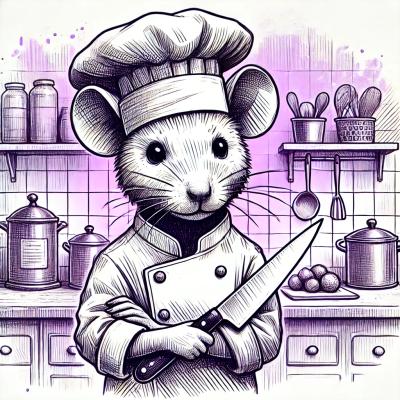
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
commandeer
Advanced tools
Proxy requests through connect and capture JSON responses before they are output
Proxy requests through Connect and capture JSON responses before they are output.
const commandeer = require('commandeer');
const connect = require('connect');
const app = connect();
app.use(commandeer({
contentType: 'application/x-commandeer+json',
dataProperty: 'proxyData',
target: 'http://localhost:1234'
}));
app.use((request, response) => {
response.proxyData.commandeered = true;
response.end(JSON.stringify(response.proxyData));
});
app.listen(3000);
Install Commandeer with npm:
npm install commandeer
Require in Connect and Commandeer:
const commandeer = require('commandeer');
const connect = require('connect');
Create a Connect application:
const app = connect();
Use the Commandeer middleware in your connect application, configuring it with a few options:
app.use(commandeer({
contentType: 'application/x-commandeer+json',
dataProperty: 'proxyData',
target: 'http://localhost:1234'
}));
Add another middleware after Commandeer to handle any JSON responses that are captured:
app.use((request, response) => {
response.proxyData.commandeered = true;
response.end(JSON.stringify(response.proxyData));
});
Start your Connect application:
app.listen(3000);
contentType
(string|array)Any responses with a matching Content-Type
header will not be proxied directly. Instead, the response body will be parsed as JSON and sent to the next middleware in the stack. E.g:
app.use(commandeer({
contentType: 'application/x-foo'
}));
app.use(function (request, response) {
// Only responses from with a Content-Type of 'application/x-foo' will reach this middleware
});
If an array of strings is passed in, the response Content-Type
will be checked against each of them:
app.use(commandeer({
contentType: [
'application/x-foo',
'application/x-bar'
]
}));
app.use((request, response) => {
// Only responses from with a Content-Type of 'application/x-foo' or 'application/x-bar' will reach this middleware
});
Defaults to 'application/x-commandeer+json'
.
dataProperty
(string)The JSON from captured responses will be stored on this property of the response. This property can be used to access the parsed JSON in the next middleware. E.g:
app.use(commandeer({
dataProperty: 'foo'
}));
app.use((request, response) => {
console.log(response.foo);
});
Defaults to 'proxyData'
.
log
(object)An object which implments the methods error
and info
which will be used to report errors and request information.
app.use(commandeer({
log: console
}));
Defaults to a mock object which doesn't output anything.
rewriteHostHeader
(boolean)Whether to rewrite the Host
header of proxied requests to match the target host. This is required for some back-ends to work properly.
Defaults to true
.
target
(string|function)The proxy target for the application. This should point to your back-end application which can serve both regular responses and proxy data reponses to be captured by Content-Type
.
If target
is a function, it will be called with a request object which can be used to decide on a target. This function must return a string.
Defaults to 'http://localhost'
.
Commandeer comes with a few example application/backend examples. To run these examples you'll need to install Foreman, or look into the Procfiles for the examples and spin up each process separately.
Simple JSON transform. Commandeerable JSON has a new property added before output.
foreman start -d example/basic
Render the JSON with a templating engine. Commandeerable JSON gets passed into Hogan.js to be rendered as a template.
foreman start -d example/hogan
Proxying to multiple backends from the same application, by using a target function rather than a string.
foreman start -d example/multiple-backends
To contribute to Commandeer, clone this repo locally and commit your code on a separate branch.
Please write unit tests for your code, and check that everything works by running the following before opening a pull-request:
make ci
Commandeer is licensed under the MIT license.
Copyright © 2015, Rowan Manning
FAQs
Proxy requests through connect and capture JSON responses before they are output
The npm package commandeer receives a total of 4 weekly downloads. As such, commandeer popularity was classified as not popular.
We found that commandeer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.