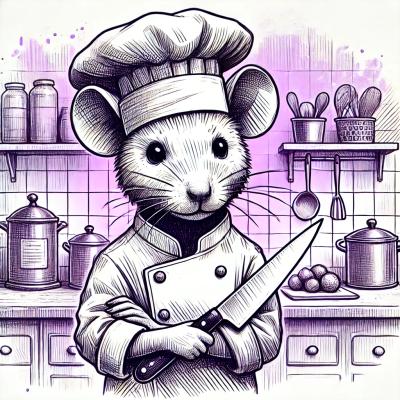
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
compute-erfinv
Advanced tools
The inverse error function is defined in terms of the Maclaurin series
where c_0 = 1
and
$ npm install compute-erfinv
For use in the browser, use browserify.
var erfinv = require( 'compute-erfinv' );
Evaluates the inverse error function. x
may be either a number
, an array
, a typed array
, or a matrix
. All numeric values must reside on the interval [-1,1]
.
var matrix = require( 'dstructs-matrix' ),
data,
mat,
out,
i;
out = erfinv( 0.5 );
// returns ~0.47694
out = erfinv( [ 0, 0.2, 0.5, 0.8, 1 ] );
// returns [ 0, 0.17914, 0.47694, 0.90619, +infinity ]
data = [ 0, 0.5, 1 ];
out = erfinv( data );
// returns [ 0, 0.47694, +infinity ]
data = new Float32Array( data );
out = erfinv( data );
// returns Float64Array( [ 0, 0.47694, +infinity ] )
data = new Float64Array( 4 );
for ( i = 0; i < 4; i++ ) {
data[ i ] = i / 2;
}
mat = matrix( data, [2,2], 'float64' );
/*
[ 0 0.25
0.5 0.75 ]
*/
out = erfinv( mat );
/*
[ 0 ~0.2253
~0.4769 ~0.8134 ]
*/
The function accepts the following options
:
function
for accessing array
values.typed array
or matrix
data type. Default: float64
.boolean
indicating if the function
should return a new data structure. Default: true
.'.'
.For non-numeric arrays
, provide an accessor function
for accessing array
values.
var data = [
['beep', 0],
['boop', 0.2],
['bip', 0.5],
['bap', 0.8],
['baz', 1]
];
function getValue( d, i ) {
return d[ 1 ];
}
var out = erfinv( data, {
'accessor': getValue
});
// returns [ 0, 0.17914, 0.47694, 0.90619, +infinity ]
To deepset an object array
, provide a key path and, optionally, a key path separator.
var data = [
{'x':[0,0]},
{'x':[1,0.25]},
{'x':[2,0.5]},
{'x':[3,0.75]},
{'x':[4,1]}
];
var out = erfinv( data, 'x|1', '|' );
/*
[
{'x':[0,0]},
{'x':[1,0.2253]},
{'x':[2,0.4769]},
{'x':[3,0.8134]},
{'x':[4,+infinity]}
]
*/
var bool = ( data === out );
// returns true
By default, when provided a typed array
or matrix
, the output data structure is float64
in order to preserve precision. To specify a different data type, set the dtype
option (see matrix
for a list of acceptable data types).
var data, out;
data = new float64Array( [0, 0.25, 0.5] );
out = erfinv( data, {
'dtype': 'int32'
});
// returns Int32Array( [0,0,0] )
// Works for plain arrays, as well...
out = erfinv( [0, 0.25, 0.5], {
'dtype': 'uint8'
});
// returns Uint8Array( [0,0,0] )
By default, the function returns a new data structure. To mutate the input data structure (e.g., when input values can be discarded or when optimizing memory usage), set the copy
option to false
.
var data,
bool,
mat,
out,
i;
var data = [ 0, 0.2, 0.5, 0.8, 1 ];
var out = erfinv( data, {
'copy': false
});
// returns [ 0, 0.17914, 0.47694, 0.90619, +infinity ]
bool = ( data === out );
// returns true
data = new Float64Array( 4 );
for ( i = 0; i < 4; i++ ) {
data[ i ] = i / 4;
}
mat = matrix( data, [2,2], 'float64' );
/*
[ 0 0.25
0.5 0.75 ]
*/
out = erfinv( mat, {
'copy': false
});
/*
[ 0 ~0.2253
~0.4769 ~0.8134 ]
*/
bool = ( mat === out );
// returns true
If an element is not a numeric value, the evaluated inverse error function is NaN
.
var data, out;
out = erfinv( null );
// returns NaN
out = erfinv( true );
// returns NaN
out = erfinv( {'a':'b'} );
// returns NaN
out = erfinv( [ true, null, [] ] );
// returns [ NaN, NaN, NaN ]
function getValue( d, i ) {
return d.x;
}
data = [
{'x':true},
{'x':[]},
{'x':{}},
{'x':null}
];
out = erfinv( data, {
'accessor': getValue
});
// returns [ NaN, NaN, NaN, NaN ]
out = erfinv( data, {
'path': 'x'
});
/*
[
{'x':NaN},
{'x':NaN},
{'x':NaN,
{'x':NaN}
]
*/
Be careful when providing a data structure which contains non-numeric elements and specifying an integer
output data type, as NaN
values are cast to 0
.
var out = erfinv( [ true, null, [] ], {
'dtype': 'int8'
});
// returns Int8Array( [0,0,0] );
var matrix = require( 'dstructs-matrix' ),
erfinv = require( 'compute-erfinv' );
var data,
mat,
out,
tmp,
i;
// Plain arrays...
data = new Array( 100 );
for ( i = 0; i < data.length; i++ ) {
data[ i ] = ( Math.random() - 0.5 ) * 2;
}
out = erfinv( data );
// Object arrays (accessors)...
function getValue( d ) {
return d.x;
}
for ( i = 0; i < data.length; i++ ) {
data[ i ] = {
'x': data[ i ]
};
}
out = erfinv( data, {
'accessor': getValue
});
// Deep set arrays...
for ( i = 0; i < data.length; i++ ) {
data[ i ] = {
'x': [ i, data[ i ].x ]
};
}
out = erfinv( data, {
'path': 'x/1',
'sep': '/'
});
// Typed arrays...
data = new Int32Array( 10 );
for ( i = 0; i < data.length; i++ ) {
data[ i ] = ( Math.random() - 0.5 ) * 2;
}
tmp = erfinv( data );
out = '';
for ( i = 0; i < data.length; i++ ) {
out += tmp[ i ];
if ( i < data.length-1 ) {
out += ',';
}
}
// Matrices...
mat = matrix( data, [5,2], 'int32' );
out = erfinv( mat );
// Matrices (custom output data type)...
out = erfinv( mat, {
'dtype': 'uint8'
});
To run the example code from the top-level application directory,
$ node ./examples/index.js
Unit tests use the Mocha test framework with Chai assertions. To run the tests, execute the following command in the top-level application directory:
$ make test
All new feature development should have corresponding unit tests to validate correct functionality.
This repository uses Istanbul as its code coverage tool. To generate a test coverage report, execute the following command in the top-level application directory:
$ make test-cov
Istanbul creates a ./reports/coverage
directory. To access an HTML version of the report,
$ make view-cov
Copyright © 2014-2015. The Compute.io Authors.
FAQs
Inverse error function.
We found that compute-erfinv demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.