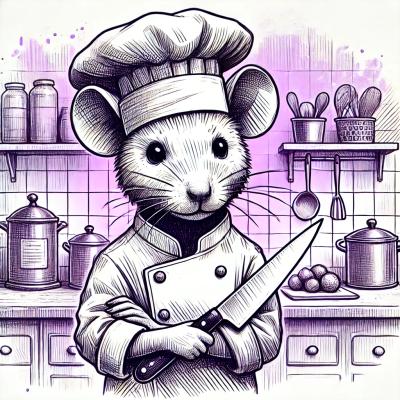
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
cordova-plugin-bluetoothclassic-serial
Advanced tools
This plugin enables serial communication over Bluetooth. It is a fork of https://github.com/soltius/BluetoothClassicSerial. The core difference is that this plugin has updated the configuration dependencies to handle the latest cordova-android and cordova-ios breaking changes.
Install with Cordova cli
$ cordova plugin add cordova-plugin-bluetoothclassic-serial
Note that this plugin's id changed from 'cordova-plugin-bluetooth-serial' to 'cordova-plugin-bluetoothclassic-serial' as part of the fork.
iOS requires that the device's protocol string is in the p-list. This plugin has a dependency on cordova-custom-config which enables plist entries to be created from entries the application's cordova config.xml file.
The Phonegap Build service doesn't use this plugin, and instead appears to have it's own method for passing entries into the iOS plist. Examples below.
Replace the text 'first.device.protocol.string' with the protocol string for the Bluetooth accessory you are connecting to. Create a new line for each protocol string. Some devices may have more than one. The plugin only allows for connection to one device and one protocol. If you need to connect to another, disconnect, then connect to the required device and protocol.
<platform name="ios">
<config-file target="*-Info.plist" parent="UISupportedExternalAccessoryProtocols">
<array>
<string>first.device.protocol.string</string>
<string>second.device.protocol.string</string>
</array>
</config-file>
</platform>
<config-file platform="ios" parent="UISupportedExternalAccessoryProtocols">
<array>
<string>first.device.protocol.string</string>
<string>second.device.protocol.string</string>
</array>
</config-file>
Connect to a Bluetooth device.
bluetoothClassicSerial.connect(deviceId, interfaceIdArray, connectSuccess, connectFailure);
Function connect
connects to a Bluetooth device. The callback is long running. Success will be called when the connection is successful. Failure is called if the connection fails, or later if the connection disconnects. An error message is passed to the failure callback. If a device has multiple interfaces then you can connect to them by providind the inteface Ids.
For iOS, connect
takes the ConnectionID as the deviceID, and the Protocol String as the interfaceId.
Connect insecurely to a Bluetooth device.
bluetoothClassicSerial.connectInsecure(deviceId, interfaceIdArray, connectSuccess, connectFailure);
Function connectInsecure
works like connect, but creates an insecure connection to a Bluetooth device. See the Android docs for more information.
For Android, see connect.
connectInsecure
is not supported.
bluetoothClassicSerial.disconnect(success, failure);
Function disconnect
disconnects the current connection.
Writes data to the serial port.
bluetoothClassicSerial.write(interfaceId, data, success, failure);
Function write
data to the serial port. Data can be an ArrayBuffer, string, array of integers, or a Uint8Array.
Internally string, integer array, and Uint8Array are converted to an ArrayBuffer. String conversion assume 8bit characters.
// string
bluetoothClassicSerial.write("00001101-0000-1000-8000-00805F9B34FB", "hello, world", success, failure);
// array of int (or bytes)
bluetoothClassicSerial.write("00001101-0000-1000-8000-00805F9B34FB", [186, 220, 222], success, failure);
// Typed Array
var data = new Uint8Array(4);
data[0] = 0x41;
data[1] = 0x42;
data[2] = 0x43;
data[3] = 0x44;
bluetoothClassicSerial.write(interfaceId, data, success, failure);
// Array Buffer
bluetoothClassicSerial.write(interfaceId, data.buffer, success, failure);
Gets the number of bytes of data available.
bluetoothClassicSerial.available(interfaceId, success, failure);
Function available
gets the number of bytes of data available. The bytes are passed as a parameter to the success callback.
available
is not supported.
bluetoothClassicSerial.available("00001101-0000-1000-8000-00805F9B34FB", function (numBytes) { console.log("There are " + numBytes + " available to read."); }, failure);
Reads data from the buffer.
bluetoothClassicSerial.read(interfaceId, success, failure);
Function read
reads the data from the buffer. The data is passed to the success callback as a String. Calling read
when no data is available will pass an empty String to the callback.
bluetoothClassicSerial.read("00001101-0000-1000-8000-00805F9B34FB", function (data) { console.log(data);}, failure);
Reads data from the buffer until it reaches a delimiter.
bluetoothClassicSerial.readUntil(interfaceId, '\n', success, failure);
Function readUntil
reads the data from the buffer until it reaches a delimiter. The data is passed to the success callback as a String. If the buffer does not contain the delimiter, an empty String is passed to the callback. Calling read
when no data is available will pass an empty String to the callback.
bluetoothClassicSerial.readUntil("00001101-0000-1000-8000-00805F9B34FB", '\n', function (data) {console.log(data);}, failure);
Subscribe to be notified when data is received.
bluetoothClassicSerial.subscribe(interfaceId, '\n', success, failure);
Function subscribe
registers a callback that is called when data is received. A delimiter must be specified. The callback is called with the data as soon as the delimiter string is read. The callback is a long running callback and will exist until unsubscribe
is called.
// the success callback is called whenever data is received
bluetoothClassicSerial.subscribe("00001101-0000-1000-8000-00805F9B34FB", '\n', function (data) {
console.log(data);
}, failure);
Unsubscribe from a subscription.
bluetoothClassicSerial.unsubscribe(interfaceId, success, failure);
Function unsubscribe
removes any notification added by subscribe
and kills the callback.
bluetoothClassicSerial.unsubscribe();
Subscribe to be notified when data is received.
bluetoothClassicSerial.subscribeRawData(interfaceId, success, failure);
Function subscribeRawData
registers a callback that is called when data is received. The callback is called immediately when data is received. The data is sent to callback as an ArrayBuffer. The callback is a long running callback and will exist until unsubscribeRawData
is called.
// the success callback is called whenever data is received
bluetoothClassicSerial.subscribeRawData(function (data) {
var bytes = new Uint8Array(data);
console.log(bytes);
}, failure);
Unsubscribe from a subscription.
bluetoothClassicSerial.unsubscribeRawData(interfaceId, success, failure);
Function unsubscribeRawData
removes any notification added by subscribeRawData
and kills the callback.
bluetoothClassicSerial.unsubscribeRawData("00001101-0000-1000-8000-00805F9B34FB");
Clears data in the buffer.
bluetoothClassicSerial.clear(interfaceId, success, failure);
Function clear
removes any data from the receive buffer.
Lists bonded devices
bluetoothClassicSerial.list(success, failure);
Function list
lists the paired Bluetooth devices. The success callback is called with a list of objects.
Example list passed to success callback. See BluetoothDevice and BluetoothClass#getDeviceClass.
[{
"class": 276,
"id": "10:BF:48:CB:00:00",
"address": "10:BF:48:CB:00:00",
"name": "Nexus 7"
}, {
"class": 7936,
"id": "00:06:66:4D:00:00",
"address": "00:06:66:4D:00:00",
"name": "RN42"
}]
Function list
lists the paired Bluetooth devices. The success callback is called with a list of objects.
Example list passed to success callback for iOS.
TBC
id
is the generic name for connection Id
or [mac]address
so that code can be platform independent.
bluetoothClassicSerial.list(function(devices) {
devices.forEach(function(device) {
console.log(device.id);
})
}, failure);
Reports the connection status. If all interfaces are connected then the success callback is called. If one interface is not connected then the failure callback is called. The connect method does not allow the status of a single interface to be determined (unless you have only specified a single interfaceId in the prior connect method).
bluetoothClassicSerial.isConnected(success, failure);
Function isConnected
calls the success callback when connected to a peer and the failure callback when not connected.
bluetoothClassicSerial.isConnected(
function() {
console.log("Bluetooth is connected");
},
function() {
console.log("Bluetooth is *not* connected");
}
);
Reports if bluetooth is enabled.
bluetoothClassicSerial.isEnabled(success, failure);
Function isEnabled
calls the success callback when bluetooth is enabled and the failure callback when bluetooth is not enabled.
bluetoothClassicSerial.isEnabled(
function() {
console.log("Bluetooth is enabled");
},
function() {
console.log("Bluetooth is *not* enabled");
}
);
Show the Bluetooth settings on the device.
bluetoothClassicSerial.showBluetoothSettings(success, failure);
Function showBluetoothSettings
opens the Bluetooth settings on the operating systems.
showBluetoothSettings
is not supported.
bluetoothClassicSerial.showBluetoothSettings();
Enable Bluetooth on the device.
bluetoothClassicSerial.enable(success, failure);
Function enable
prompts the user to enable Bluetooth. If enable
is called when Bluetooth is already enabled, the user will not prompted and the success callback will be invoked.
enable
is not supported.
enable
is only supported on Android and does not work on iOS.
If enable
is called when Bluetooth is already enabled, the user will not prompted and the success callback will be invoked.
bluetoothClassicSerial.enable(
function() {
console.log("Bluetooth is enabled");
},
function() {
console.log("The user did *not* enable Bluetooth");
}
);
Discover unpaired devices
bluetoothClassicSerial.discoverUnpaired(success, failure);
The behaviour of this method varies between Android and iOS.
Function discoverUnpaired
discovers unpaired Bluetooth devices. The success callback is called with a list of objects similar to list
, or an empty list if no unpaired devices are found.
Example list passed to success callback.
[{
"class": 276,
"id": "10:BF:48:CB:00:00",
"address": "10:BF:48:CB:00:00",
"name": "Nexus 7"
}, {
"class": 7936,
"id": "00:06:66:4D:00:00",
"address": "00:06:66:4D:00:00",
"name": "RN42"
}]
The discovery process takes a while to happen. You can register notify callback with setDeviceDiscoveredListener. You may also want to show a progress indicator while waiting for the discover process to finish, and the success callback to be invoked.
Calling connect
on an unpaired Bluetooth device should begin the Android pairing process.
Function discoverUnpaired
will launch a native iOS window showing all devices which match the protocol string defined in the application's cordova config.xml file. Choosing a device from the list will initiate pairing and the details of that device will not trigger the success callback function. The device discovered listener must be used. Once paired the device is available for connection.
bluetoothClassicSerial.discoverUnpaired(function(devices) {
devices.forEach(function(device) {
console.log(device.id);
})
}, failure);
Register a notify callback function to be called during bluetooth device discovery.
Register a notify callback function to be called during bluetooth device discovery. For callback to work, discovery process must be started with discoverUnpaired. There can be only one registered callback.
Example object passed to notify callback.
{
"class": 276,
"id": "10:BF:48:CB:00:00",
"address": "10:BF:48:CB:00:00",
"name": "Nexus 7"
}
When a device is paired from the discoverUnpaired function it's details will be passed to the callback function. Unlike Android this will only be fired once for the selected device, not for all the available devices.
bluetoothClassicSerial.setDeviceDiscoveredListener(function(device) {
console.log('Found: ',device.id);
});
Clears notify callback function registered with setDeviceDiscoveredListener.
bluetoothClassicSerial.clearDeviceDiscoveredListener();
Development Devices include
Development Devices include
This project is a fork of Don Coleman's https://github.com/don/BluetoothSerial so all the big props to him.
The multi interface implementation for Android borrowed ideas from Shikoruma's pull request https://github.com/don/BluetoothSerial/pull/205 to Don Coleman's Cordova BluetoothSerial Plugin.
Most of the Bluetooth implementation was borrowed from the Bluetooth Chat example in the Android SDK.
The API for available, read, readUntil was influenced by the BtSerial Library for Processing for Arduino
If you don't need serial over Bluetooth, try the PhoneGap Bluetooth Plugin for Android or perhaps phonegap-plugin-bluetooth.
If you need generic Bluetooth Low Energy support checkout Don Colemans's Cordova BLE Plugin.
If you need BLE for RFduino checkout Don Colemans's RFduino Plugin.
For Windows Phone 8 support see the original project, Don Coleman's Cordova BluetoothSerial Plugin
An example a properly formatted mac address is "AA:BB:CC:DD:EE:FF"
Try the code. If you find an problem or missing feature, file an issue or create a pull request.
FAQs
Bluetooth Serial Communication Plugin
The npm package cordova-plugin-bluetoothclassic-serial receives a total of 199 weekly downloads. As such, cordova-plugin-bluetoothclassic-serial popularity was classified as not popular.
We found that cordova-plugin-bluetoothclassic-serial demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.