What is countries-and-timezones?
The 'countries-and-timezones' npm package provides utilities for working with country and timezone data. It allows you to retrieve information about countries, their timezones, and perform various operations related to timezones.
What are countries-and-timezones's main functionalities?
Get Country Information
This feature allows you to retrieve information about a specific country using its ISO 3166-1 alpha-2 code. The returned object includes details such as the country's name, timezones, and other relevant data.
const ctz = require('countries-and-timezones');
const country = ctz.getCountry('US');
console.log(country);
Get Timezone Information
This feature allows you to retrieve information about a specific timezone using its IANA timezone identifier. The returned object includes details such as the timezone's name, offset, and other relevant data.
const ctz = require('countries-and-timezones');
const timezone = ctz.getTimezone('America/New_York');
console.log(timezone);
Get All Countries
This feature allows you to retrieve a list of all countries supported by the package. The returned object includes details for each country.
const ctz = require('countries-and-timezones');
const countries = ctz.getAllCountries();
console.log(countries);
Get All Timezones
This feature allows you to retrieve a list of all timezones supported by the package. The returned object includes details for each timezone.
const ctz = require('countries-and-timezones');
const timezones = ctz.getAllTimezones();
console.log(timezones);
Get Timezones for a Country
This feature allows you to retrieve a list of all timezones for a specific country using its ISO 3166-1 alpha-2 code. The returned array includes details for each timezone.
const ctz = require('countries-and-timezones');
const timezones = ctz.getTimezonesForCountry('US');
console.log(timezones);
Other packages similar to countries-and-timezones
moment-timezone
The 'moment-timezone' package is an extension for the 'moment' library to handle timezones. It provides utilities for parsing, manipulating, and displaying dates and times in various timezones. Compared to 'countries-and-timezones', 'moment-timezone' focuses more on date and time manipulation rather than country-specific data.
timezone-support
The 'timezone-support' package provides utilities for working with timezones and converting dates between different timezones. It includes features for parsing and formatting dates, as well as handling daylight saving time. Compared to 'countries-and-timezones', 'timezone-support' is more focused on date and time operations rather than providing country-specific information.
world-countries
The 'world-countries' package provides a comprehensive list of countries with various details such as names, codes, and regions. It is similar to 'countries-and-timezones' in terms of providing country data, but it does not include timezone information.
countries-and-timezones

This is a minimalistic library to work with countries and timezones data.
Install
npm install --save countries-and-timezones
Data models
Country
A country is defined by the following parameters:
Parameter | Type | Description |
---|
id | String | The country ISO 3166-1 code. |
name | String | Preferred name of the country. |
timezones | Array[String] | The list of timezones used in the country. |
{
id: 'MX',
name: 'Mexico',
timezones: [
'America/Bahia_Banderas',
'America/Cancun',
'America/Chihuahua',
'America/Ensenada',
'America/Hermosillo',
'America/Matamoros',
'America/Mazatlan',
'America/Merida',
'America/Mexico_City',
'America/Monterrey',
'America/Ojinaga',
'America/Santa_Isabel',
'America/Tijuana'
]
}
Timezone
A timezone is defined by the following parameters:
Parameter | Type | Description |
---|
name | String | The name of the timezone, from tz database. |
country | String | The ISO 3166-1 code of the country where it's used. Etc/* , GMT and UTC timezones don't have and associated country. |
utcOffset | Number | The difference in minutes between the timezone and UTC. |
utcOffsetStr | String | The difference in hours and minutes between the timezone and UTC, expressed as string with format: ±[hh]:[mm] . |
dstOffset | Number | The difference in minutes between the timezone and UTC during daylight saving time (DST). When utcOffset and dstOffset are the same, means that the timezone does not observe a daylight saving time. |
dstOffsetStr | String | The difference in hours and minutes between the timezone and UTC during daylight saving time (DST, expressed as string with format: ±[hh]:[mm] . |
aliasOf | String | The name of a primary timezone in case this is an alias. null means this is a primary timezone. |
{
name: 'Asia/Tel_Aviv',
country: 'IL',
utcOffset: 120,
utcOffsetStr: '+02:00',
dstOffset: 180,
dstOffsetStr: '+03:00',
aliasOf: 'Asia/Jerusalem'
}
API
.getCountry(id)
Returns a country referenced by its id
.
Example
const ct = require('countries-and-timezones');
const country = ct.getCountry('DE');
console.log(country);
.getTimezone(name)
Returns a timezone referenced by its name
.
Example
const ct = require('countries-and-timezones');
const timezone = ct.getTimezone('America/Los_Angeles');
console.log(timezone);
.getAllCountries()
Returns an object with the data of all countries.
Example
const ct = require('countries-and-timezones');
const countries = ct.getAllCountries();
console.log(countries);
.getAllTimezones()
Returns an object with the data of all timezones.
Example
const ct = require('countries-and-timezones');
const timezones = ct.getAllTimezones();
console.log(timezones);
.getTimezonesForCountry(id)
Returns an array with all the timezones of a country given its id
.
Example
const ct = require('countries-and-timezones');
const timezones = ct.getTimezonesForCountry('MX');
console.log(timezones);
.getCountryForTimezone(name)
Returns the country that uses a timezone given its name
.
Example
const ct = require('countries-and-timezones');
const timezone = ct.getCountryForTimezone('Asia/Tokyo');
console.log(timezone);
Related projects
Working on something more complex?
Meet Spott:
- Search any city, country or administrative division in the world. By full strings or autocompletion.
- Find a place by an IP address.
- Access to more than 240,000 geographical places. In more than 20 languages.
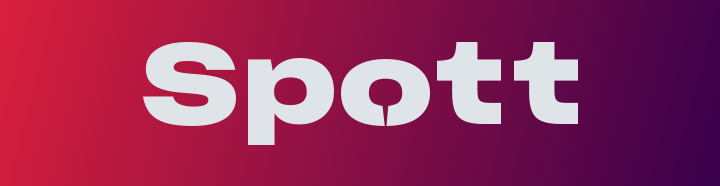
License
MIT