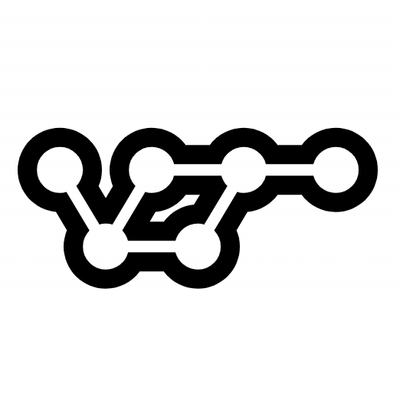
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
craco-alias
Advanced tools
A craco plugin for automatic aliases generation for Webpack and Jest.
Install craco
Install craco-alias
:
npm i -D craco-alias
yarn add -D craco-alias
Edit craco.config.js
:
const CracoAlias = require("craco-alias");
module.exports = {
plugins: [
{
plugin: CracoAlias,
options: {
// see in examples section
}
}
]
};
Go to Examples section
source
:
One of "options"
, "jsconfig"
, "tsconfig"
Optional, defaults to "options"
baseUrl
:
A base url for aliases. (./src
for example)
Optional, defaults to ./
(project root directory)
aliases
:
An object with aliases names and paths
Only required when source
is set to "options"
tsConfigPath
:
A path to tsconfig file
Only required when source
is set to "tsconfig"
filter
:
A function of type ([key, value]) => boolean
Optional, used to remove some aliases from the resulting config
Example: ([key]) => !key.startsWith('node_modules')
unsafeAllowModulesOutsideOfSrc
:
Allow importing modules outside of ./src
folder.
Disables webpack ModuleScopePlugin
.
debug
:
Enable it if you ran into a problem. It will log a useful info in console.
Optional, defaults to false
Note: you don't need to add
/*
part for directories in this case
/* craco.config.js */
const CracoAlias = require("craco-alias");
module.exports = {
plugins: [
{
plugin: CracoAlias,
options: {
source: "options",
baseUrl: "./",
aliases: {
"@file": "./src/file.js",
"@dir": "./src/some/dir",
// you can alias packages too
"@material-ui": "./node_modules/@material-ui-ie10"
}
}
}
]
};
/* craco.config.js */
const CracoAlias = require("craco-alias");
module.exports = {
plugins: [
{
plugin: CracoAlias,
options: {
source: "jsconfig",
// baseUrl SHOULD be specified
// plugin does not take it from jsconfig
baseUrl: "./src"
}
}
]
};
Note: your jsconfig should always have
compilerOptions.paths
property.baseUrl
is optional for plugin, but some IDEs and editors require it for intellisense.
/* jsconfig.json */
{
"compilerOptions": {
"baseUrl": "./src",
"paths": {
// file aliases
"@baz": ["./baz.js"],
"@boo": ["./boo.jsx"],
// folder aliases
"@root": ["./"],
"@root/*": ["./*"],
"@lib": ["./lib"],
"@lib/*": ["./lib/*"],
// package aliases (types is optional without ts)
"@my-react-select": [
"../node_modules/react-select",
"../node_modules/@types/react-select"
],
"@my-react-select/*": [
"../node_modules/react-select/*",
"../node_modules/@types/react-select"
]
}
}
}
Go to project's root directory.
Create tsconfig.extend.json
.
Edit it as follows:
{
"compilerOptions": {
"baseUrl": "./src",
"paths": {
// file aliases
"@baz": ["./baz.ts"],
"@boo": ["./boo.tsx"],
// folder aliases
"@root": ["./"],
"@root/*": ["./*"],
"@lib": ["./lib"],
"@lib/*": ["./lib/*"],
// package aliases
"@my-react-select": [
"../node_modules/react-select",
"../node_modules/@types/react-select"
],
"@my-react-select/*": [
"../node_modules/react-select/*",
"../node_modules/@types/react-select"
]
}
}
}
Go to tsconfig.json
.
Extend tsconfig.json
from tsconfig.extend.json
:
{
+ "extends": "./tsconfig.extend.json",
"compilerOptions": {
...
},
...
}
Edit craco.config.js
:
const CracoAlias = require("craco-alias");
module.exports = {
plugins: [
{
plugin: CracoAlias,
options: {
source: "tsconfig",
// baseUrl SHOULD be specified
// plugin does not take it from tsconfig
baseUrl: "./src",
// tsConfigPath should point to the file where "baseUrl" and "paths" are specified
tsConfigPath: "./tsconfig.extend.json"
}
}
]
};
Make sure your config is valid.
Set debug
to true
in options.
Run application again.
Copy a printed info.
Here, create an issue describing your problem (do not forget to add the debug info).
Install:
yarn
Use yarn please. npm may fail the dependencies installation.
Run tests:
yarn test
FAQs
A craco plugin for automatic aliases generation
The npm package craco-alias receives a total of 19,473 weekly downloads. As such, craco-alias popularity was classified as popular.
We found that craco-alias demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.