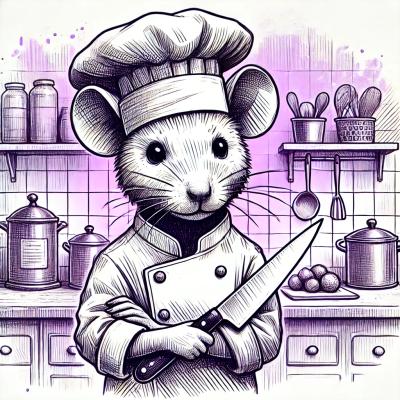
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
danbooru api wrapper
My api wrapper is super simple! You just require it, then refer to Danbooru's lovely api documentation and make requests!
var Danbooru = require('danbooru');
Danbooru.get('posts', {limit: 5, tags: 'cat_ears'}, function(err, data) {
if(err) throw err;
console.log(data); // All of your cute kittehgirls!
});
You can also use Danbooru.post()
, Danbooru.put()
, and Danbooru.delete()
. They all have the same parameters and give you the same callback!
Perform a http request on Danbooru's api!
method
property. One of get
, post
, put
, or delete
, depending on what type of request you would like to make.path
string. The API documentation mentions a base URL. You put that here! The slash and .json
are optional. In fact, the entire thing is optional, but you won't get anything useful from omitting path.params
object. Just provide your parameters as an object!callback
function(err, data). What do you wannya do after you get your api request?
err
Error. Node.js callbacks always give you an error for some reason. Here you go!data
object. Parsing JSON output is an extra step, so you don't hafta do it! Here's an already-parsed object for you!A simple wrapper function for request, with baseUrl
always set to Danbooru. Refer to their documentation for details.
options
string. object. A url or options for your request.callback
function(err, response, body). Callback function. response
is a usual node HTTP response, while body
is the body of that response, ready for using.I'm providing this to give you an easy way to download images and make custom requests without having to type Danbooru's url:
Danbooru.request('/data/9b5d16968321eff393fea8d735d69de3.jpg')
.pipe(fs.create-write-stream('cutiefox.jpg'));
You know what's a pain? Having to type the same stuff over and over again. You know what you hafta do if you want to be authenticated on Danbooru? Send your login
and api_key
over and over again.
var authedBooru = new Danbooru({login: 'topsecret', api_key: 'evenmoresecret'});
authedBooru.post('favorites', {post_id: 2288637}, function(err, data) {
if(err) throw err;
console.log('Successfully favorited!'); // Wow, you do like kittehgirls!
});
If that's still too much typing for you, you can use a shortcut!
var shortBooru = Danbooru('topsecret', 'evenmoresecret');
Save parameters for later. Returns a new Danbooru object that you can use to make requests with those saved parameters.
object
object. string. If you provide an object, it'll be used as default parameters for all requests you make! If you provide a string, it'll set your default login
parameter to whatever you provide! If you provide neither, you'll create a new, empty Danbooru
object.api_key
string. If (and only if) you provided a string for object
, this will be used as your default api_key
parameter!"But wait," you say, "APIs are supposed to help make my life easier! Why do I still have to type so much?" Well, I made a helper function called .search()
for you, and as a bonus, its data object even gives you extra helper functions to get around more easily! The helper functions are also returned by .search()
, so you can chain more nicely!
Danbooru.search('1girl fox_ears', function(err, page1) {
if(err) throw err;
console.log(page1); // Foxgirls!
}).next(function(err, page2) {
if(err) throw err;
console.log(page2); // More foxgirls!
page2.next(function(err, page3) {
if(err) throw err;
console.log(page3); // So many foxgirls~ ♥
});
});
Perform a search on Danbooru. A shortcut for Danbooru.get('posts', {tags: tags, limit: 100, ...params}, callback)
, but also adds on extra methods to the data object you get. Visit an api result in your browser to inspect the data object provided.
tags
string. A space separated list of tags, and basically your Danbooru search query. You can try out your query visually on Danbooru or look up their searching reference if you're not sure what to type.params
object. These are just parameters that will be directly passed to Danbooru's API. It will contain limit: 100
by default, but you can change the number of posts you want by specifying it. Trying to specify tags
won't do anything, because the tags
parameter always overwrites the value of tags
here, even if tags
is empty or missing (which makes it default to empty).callback
function(err, searchData) Do something after your request comes back.
err
Error. Like always, an error object if there was one.searchData
object. Whatever Danbooru's API returns, but with some extra methods and properties. More details below!searchData
. Also details below~Like I've said probably three times already, this data object is the one that Danbooru's api gives you, but with some nice helper functions! You can see sample API output by visiting Danbooru. I haven't told you what the methods are yet, so~
This is a property that tells you what your current page number is. You can't change it.
Calls Danbooru.search()
again with the same tags and parameters as last time, but with the page of results you want! Trying to load a page number less than 1 will just set it to 1.
page
number. What page of results would you like? Defaults to your current page, so you can actually use searchData.load(callback)
to refresh your data.callback
function(err, searchData). This literally gives you the same type of object as the searchData
you're currently looking at.Calls searchData.load(this.page + modifier, callback)
, which basically increases your page number by your specified modifier. This will usually give you older posts (unless you specify a negative modifier for some reason).
modifier
number. What number would you like to increment your page number by?callback
function(err, searchData). Same as above.Calls searchData.load(this.page - modifier, callback)
, which basically decreases your page number by your specified modifier. This will usually give you newer posts (unless you specify a negative modifier for some reason).
modifier
number. What number would you like to decrement your page number by?callback
function(err, searchData). Same as above.This is a property that tells you what tags your search is currently for. You can't change it.
Calls Danbooru.search()
again with the same parameters as last time, but with your tagMod
added to the end of your tags
and page
set back to 1.
tagMod
string. This basically just takes your current tags and then adds these tags to it. In other words, effectively Danbooru.search(searchData.tags + " " + tagMod, params, callback)
.callback
function(err, searchData). Meow.Calls Danbooru.search()
again with the same parameters as last time, removing the tags in your tagMod
from tags
and page
set back to 1.
tagMod
string. This list of tags is separated by spaces, and then any tags here are removed from your tags for your new search.callback
function(err, searchData). Nyaa.Gives you a random post from the set of posts you've found. Doesn't return anything when you don't have any posts.
searchData
.When you're searching, you're probably looking for posts. When you're looking for posts, you probably wannya make get requests to their image URLs to be able to use them. Rather than make you write Danbooru.request(post.file_url)
over and over again, how about we make things simpler for you? This example uses random
, but you could also just go to any valid index and it would still work.
var authBooru = new Danbooru('maidlover', 'maidsarecute');
authBooru.search('maid', function(err, data) {
if(err) throw err;
data.random().favorite(); // Favoriting a random maid~ H-how bold!
});
This does a get request to your post's file_url
, which is usually your post's full size image. This also uses request, just like Danbooru.request()
.
callback
function(err, response, body). Callback function. response
is a usual node HTTP response, while body
is your image data.This does a get request to your post's large_file_url
, which is a large image appropriate for browsers, but not original size. It's sometimes the same image as file_url
. This also uses request, just like Danbooru.request()
.
callback
function(err, response, body). Callback function. response
is a usual node HTTP response, while body
is your image data.This does a get request to your post's preview_file_url
, which is usually your post's tiny preview image, useful for thumbnails. This also uses request, just like Danbooru.request()
.
callback
function(err, response, body). Callback function. response
is a usual node HTTP response, while body
is your image data.This function only really works with an authenticated Danbooru object, but you can use it to quickly favorite or unfavorite a post!
yes
boolean. Defaults to yes. Pass false
to perform an unfavorite instead.callback
function(err, data). It's a callback.This property contains a human friendly post url. This is where you would find this post if you were manually browsing Danbooru in a browser.
FAQs
danbooru api wrapper
The npm package danbooru receives a total of 21 weekly downloads. As such, danbooru popularity was classified as not popular.
We found that danbooru demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.