dbl-api.js
wrapper for doing anything with all major botlists
npm install dbl-api.js
yarn add dbl-api.js
pnpm add dbl-api.js
Table of Contents
About
Discord Bot List is a comprehensive library that simplifies interacting with major bot lists. It not only allows you to easily post your bot's statistics to multiple Discord bot lists but also provides various other useful functions. With this library, you can check who has voted for your bot, retrieve detailed information about bots and users, and much more. It supports popular bot listing platforms and is designed to be user-friendly.
Please note that while this library aims to support all major bot lists, some lists may not have been thoroughly tested yet.
Supported Bot Lists
Icon | Name | Website | Class Name |
---|
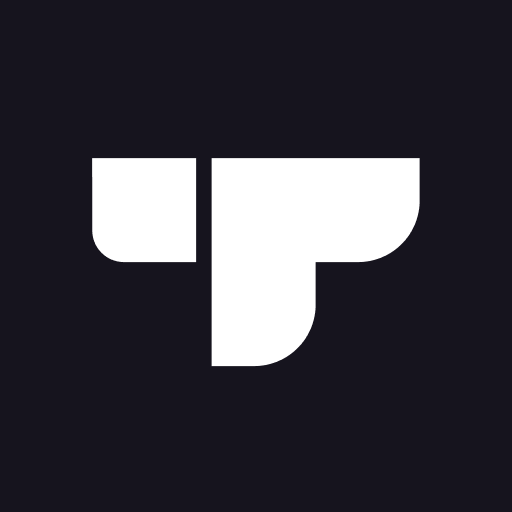 | Top.gg | top.gg | TopGG |
Examples
Posting Bot Stats
const { BotList, BotListPoster } = require('dbl-api.js');
const lists = [
new BotList.TopGG('you-bot-id', 'your-top.gg-api-token')
];
const poster = new BotListPoster(lists, {
shardCount: () => 1,
guildCount: () => 25,
userCount: () => 45778,
voiceConnectionCount: () => 3,
});
poster.postStatistics();
poster.startAutoPosting();
poster.startAutoPosting(5 * 60 * 1000);
poster.stopAutoPoster();
Recieving Webhooks
- This is a beta feature and is not fully tested yet
- Webhook URL:
https://example.com/receive/<list>/vote
const { BotList, Webhook } = require('dbl-api.js');
const lists = [
new BotList.TopGG('you-bot-id', 'your-top.gg-api-token')
];
const poster = new Webhook(lists, {
port: 3000,
handleAfterVote: (req, res) => {
},
});
webhook.on(Webhook.Events.Ready, () => {
console.log("Webhook is ready!")
})
webhook.on(Webhook.Events.NewVote, (botList, vote) => {
console.log(`New vote on ${botList.key} from ${vote.userId}`)
})
Bot List Specific Functions
Top.gg
- Importing
const { BotList : TopGG } = require('dbl-api.js');
- Constructor
const topgg = new TopGG('bot-id', 'api-token', 'webhook-password);
- Methods
topgg.getBot('bot-id').then(response => {
console.log(response);
}).catch(console.error);
topgg.getUser('user-id').then(response => {
console.log(response);
}).catch(console.error);
topgg.hasVoted().then(response => {
console.log(response);
}).catch(console.error);