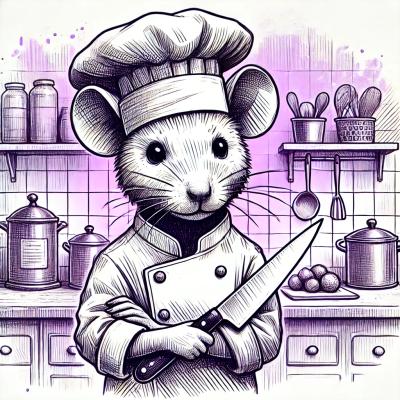
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
An ES6+ sourced DOMMatrix shim for Node.js apps and legacy browsers.
The constructor is almost equivalent with the DOMMatrix in most respects. In contrast with the original source there have been a series of changes to the prototype for consistency, performance as well as requirements to better accomodate the DOMMatrix interface:
toFloat64Array()
calls (which also returns items in the expected order);afine
property, it's a very old WebKitCSSMatrix defined property;inverse()
instance method, will be re-added later for other implementations;transform()
instance method, replaced with something that actually works;toFullString()
instance method, probably something also from WebKitCSSMatrix;translate()
, scale()
and rotate()
instance methods to work with one axis transformation, also inline with DOMMatrix;toString()
instance method to work with the new method toArray()
described below;setMatrixValue()
instance method to do all the heavy duty work with parameters;is2D
(getter and setter) property;isIdentity
(getter and setter) property;fromMatrix()
, fromFloat64Array()
and fromFloat32Array()
instance methods;toArray()
, toFloat64Array()
and toFloat32Array()
instance methods;transformPoint()
instance method which works like the original and replaces the old transform()
method.npm install dommatrix
The initialization doesn't support CSS syntax strings with transform functions like rotate()
or translate()
only matrix()
and matrix3d()
, or 6/16 elements arrays.
Basics
// ES6+
import CSSMatrix from 'dommatrix'
// init
let myMatrix = new CSSMatrix('matrix(1,0.25,-0.25,1,0,0)')
OR
// Node.js
var CSSMatrix = require('dommatrix');
// init
let myMatrix = new CSSMatrix()
Advanced API Examples
import CSSMatrix from 'dommatrix'
// init
let myMatrix = new CSSMatrix('matrix(1,0.25,-0.25,1,0,0)')
// the above is equivalent with providing the values are arguments
let myMatrix = new CSSMatrix(1,0.25,-0.25,1,0,0)
// or by providing an Array, Float32Array, Float64Array
let myMatrix = new CSSMatrix([1,0.25,-0.25,1,0,0])
// call methods to apply transformations
let myMatrix = new CSSMatrix().translate(15)
// equivalent to
let myMatrix = new CSSMatrix().translate(15,0)
// equivalent to
let myMatrix = new CSSMatrix().translate(15,0,0)
// rotations work as expected
let myMatrix = new CSSMatrix().rotate(15)
// equivalent to
let myMatrix = new CSSMatrix().rotate(0,0,15)
translate(x, y, z)
The translate method returns a new matrix which is this matrix post multiplied by a translation matrix containing the passed values. If the z
parameter is undefined, a 0 value is used in its place. This matrix is not
modified.
Parameters:
x
the X axis component of the translation value.y
the Y axis component of the translation value.z
the Z axis component of the translation value.rotate(rx, ry, rz)
The rotate method returns a new matrix which is this matrix post multiplied by each of 3 rotation matrices about the major axes, first X, then Y, then Z. If the y
and z
components are undefined, the x
value is used to rotate the
object about the z
axis, as though the vector (0,0,x) were passed. All rotation values are expected to be in degrees. This matrix is not modified.
Parameters:
rx
the X axis component of the rotation value.ry
the Y axis component of the rotation value.rz
the Z axis component of the rotation value.rotateAxisAngle(x, y, z, angle)
The rotateAxisAngle method returns a new matrix which is this matrix post multiplied by a rotation matrix with the given axis and angle
. The right-hand rule is used to determine the direction of rotation. All rotation values are
in degrees. This matrix is not modified.
Parameters:
x
The X component of the axis vector.y
The Y component of the axis vector.z
The Z component of the axis vector.angle
The angle of rotation about the axis vector, in degrees.scale(x, y, z)
The scale method returns a new matrix which is this matrix post multiplied by a scale matrix containing the passed values. If the z
component is undefined, a 1 value is used in its place. If the y
component is undefined, the x
component value is used in its place. This matrix is not modified.
Parameters:
x
the X axis component of the scale value.y
the Y axis component of the scale value.z
the Z axis component of the scale value.skewX(angle)
Specifies a skew transformation along the x-axis
by the given angle. This matrix is not modified.
The angle
parameters sets the amount in degrees to skew.
skewY(angle)
Specifies a skew transformation along the y-axis
by the given angle. This matrix is not modified.
The angle
parameters sets the amount in degrees to skew.
toString()
Creates and returns a string representation of the matrix in CSS matrix syntax, using the appropriate CSS matrix notation. The 16 items in the array 3D matrix array are transposed in row-major order.
Depending on the value of is2D
, the method will return the CSS matrix syntax in one of the two formats:
matrix3d(m11,m12,m13,m14,m21,m22,m23,m24,m31,m32,m33,m34,m41,m42,m43,m44)
matrix(a, b, c, d, e, f)
transformPoint(point)
Transforms the specified point using the matrix, returning a new DOMPoint
like Object containing the transformed point.
Neither the matrix nor the original point are altered.
The method is equivalent with transformPoint()
method of the DOMMatrix
constructor.
The point
parameter expects a vector Object with x
, y
, z
and w
properties or a DOMPoint
multiply(m2)
The multiply method returns a new CSSMatrix
which is the result of this matrix multiplied by the passed matrix, with the passed matrix to the right. This matrix as well as the one passed are not modified.
The m2
parameter is expecting a CSSMatrix
or DOMMatrix
instance.
setMatrixValue(string)
The setMatrixValue method replaces the existing matrix with one computed in the browser. EG: matrix(1,0.25,-0.25,1,0,0)
.
The method also accepts 6/16 elements Float64Array / Float32Array / Array values, the result of DOMMatrix
/ CSSMatrix
instance method calls toFloat64Array()
/ toFloat32Array()
.
For simplicity reasons, this method expects only valid matrix() / matrix3d() string values, which means other transform functions like translate(), rotate() are not supported.
Parameter:
source
parameter is either the String representing the CSS syntax of the matrix, which is also the result of getComputedStyle()
.source
can also be an Array resulted from toFloat64Array()
method calls.setIdentity()
Set the current CSSMatrix
instance to the identity form and returns it.
toArray()
Returns an Array containing all 16 elements which comprise the 3D matrix. The method can return either the elements in default column major order or row major order (what we call the transposed matrix, used by toString
).
If the matrix attribute is2D
is true
, the 6 elements array matrix is returned.
Other methods make use of this method to feed their output values from this matrix.
The transposed
parameter changes the order of the elements in the output. By default the column major order is used, which is the standard representation of a typical 4x4 3D transformation matrix, however the CSS
syntax requires the row major order, so we can set this parameter to true
to facilitate that.
toFloat64Array() and toFloat32Array()
Both return a new Float64Array
/ toFloat32Array
containing all 6/16 elements which comprise the matrix. The elements are stored into the array as double-precision floating-point numbers (Float64Array
) or single-precision floating-point numbers (Float32Array
), in column-major (colexographical access access or "colex") order. Both these methods utilize the above described method.
The result can be immediatelly fed as parameter for the initialization of a new matrix.
fromMatrix(m2)
Creates a new mutable CSSMatrix
object given an existing matrix or a DOMMatrix
Object which provides the values for its properties. The m2
parameter is the matrix instance passed into the method and neither this matrix or the one passed are modified.
fromArray(array), fromFloat64Array(array) and fromFloat32Array(array)
Each of these methods create a new mutable CSSMatrix
object given an array of values. The fromFloat64Array
and fromFloat32Array
are only aliases for fromArray
for now, but will be updated accordingly later if required.
If the array has six values, the result is a 2D matrix; if the array has 16 values, the result is a 3D matrix. Otherwise, a console.error
is thrown and returns the current matrix.
The array
parameter is the source to feed the values for the new matrix.
isIdentity
A Boolean
whose value is true
if the matrix is the identity matrix. The identity matrix is one in which every value is 0 except those on the main diagonal from top-left to bottom-right corner (in other words, where the offsets in each direction are equal).
is2D
A Boolean
flag whose value is true
if the matrix was initialized as a 2D matrix and false
if the matrix is 3D.
DOMMatrix shim is MIT Licensed.
FAQs
ES6+ shim for DOMMatrix
The npm package dommatrix receives a total of 366,257 weekly downloads. As such, dommatrix popularity was classified as popular.
We found that dommatrix demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.