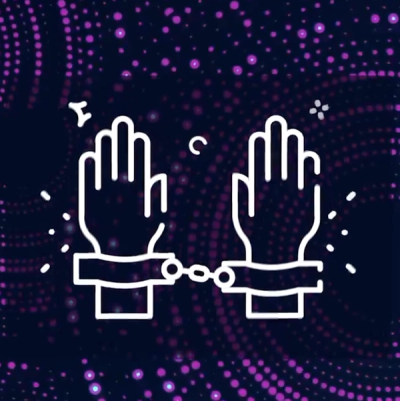
Security News
Dutch National Police Disrupt Redline and Meta Malware Operations
Dutch National Police and FBI dismantle Redline and Meta infostealer malware-as-a-service operations in Operation Magnus, seizing servers and source code.
The dset npm package is a tiny utility for safely setting deep values in JavaScript objects. It is designed to be fast and efficient, making it ideal for performance-sensitive applications.
Set deep values
This feature allows you to set a value deep within an object using a dot-separated string path. The dset function will create any necessary intermediate objects.
const dset = require('dset');
let obj = {};
dset(obj, 'a.b.c', 42);
console.log(obj); // { a: { b: { c: 42 } } }
Array index support
dset supports setting values within arrays by using bracket notation in the path string. This allows for complex nested structures involving both objects and arrays.
const dset = require('dset');
let obj = {};
dset(obj, 'a.b[0].c', 42);
console.log(obj); // { a: { b: [ { c: 42 } ] } }
Custom separator
You can specify a custom separator for the path string, allowing for flexibility in how paths are defined.
const dset = require('dset');
let obj = {};
dset(obj, 'a/b/c', 42, '/');
console.log(obj); // { a: { b: { c: 42 } } }
Lodash is a popular utility library that provides a set function for setting deep values in objects. While lodash.set is more feature-rich and part of a larger library, it is also larger in size compared to dset.
dot-prop is another utility for managing deep properties in JavaScript objects. It offers similar functionality to dset but includes additional features like getting and deleting properties. However, it is slightly larger in size.
set-value is a utility for setting nested values in objects using dot notation. It is similar in functionality to dset but includes additional options for customizing behavior. It is also larger in size compared to dset.
A tiny (197B) utility for safely writing deep Object values~!
For accessing deep object properties, please see dlv
.
Using GraphQL? You may want
dset/merge
– see Merging for more info.
$ npm install --save dset
There are two "versions" of dset
available:
dset
Size (gzip): 197 bytes
Availability: CommonJS, ES Module, UMD
import { dset } from 'dset';
dset/merge
Size (gzip): 307 bytes
Availability: CommonJS, ES Module, UMD
import { dset } from 'dset/merge';
import { dset } from 'dset';
let foo = { abc: 123 };
dset(foo, 'foo.bar', 'hello');
// or: dset(foo, ['foo', 'bar'], 'hello');
console.log(foo);
//=> {
//=> abc: 123,
//=> foo: { bar: 'hello' },
//=> }
dset(foo, 'abc.hello', 'world');
// or: dset(foo, ['abc', 'hello'], 'world');
console.log(foo);
//=> {
//=> abc: { hello: 'world' },
//=> foo: { bar: 'hello' },
//=> }
let bar = { a: { x: 7 }, b:[1, 2, 3] };
dset(bar, 'b.1', 999);
// or: dset(bar, ['b', 1], 999);
// or: dset(bar, ['b', '1'], 999);
console.log(bar);
//=> {
//=> a: { x: 7 },
//=> b: [1, 999, 3],
//=> }
dset(bar, 'a.y.0', 8);
// or: dset(bar, ['a', 'y', 0], 8);
// or: dset(bar, ['a', 'y', '0'], 8);
console.log(bar);
//=> {
//=> a: {
//=> x: 7,
//=> y: [8],
//=> },
//=> b: [1, 999, 3],
//=> }
let baz = {};
dset(baz, 'a.0.b.0', 1);
dset(baz, 'a.0.b.1', 2);
console.log(baz);
//=> {
//=> a: [{ b: [1, 2] }]
//=> }
The main/default dset
module forcibly writes values at the assigned key-path. However, in some cases, you may prefer to merge values at the key-path. For example, when using GraphQL's @stream
and @defer
directives, you will need to merge the response chunks into a single object/list. This is why dset/merge
exists~!
Below is a quick illustration of the difference between dset
and dset/merge
:
let input = {
hello: {
abc: 123
}
};
dset(input, 'hello', { world: 123 });
console.log(input);
// via `dset`
//=> {
//=> hello: {
//=> world: 123
//=> }
//=> }
// via `dset/merge`
//=> {
//=> hello: {
//=> abc: 123,
//=> world: 123
//=> }
//=> }
As shown in the examples above, all dset
interactions mutate the source object.
If you need immutable writes, please visit clean-set
(182B).
Alternatively, you may pair dset
with klona
, a 366B utility to clone your source(s). Here's an example pairing:
import { dset } from 'dset';
import { klona } from 'klona';
export function deepset(obj, path, val) {
let copy = klona(obj);
dset(copy, path, val);
return copy;
}
Returns: void
Type: Object
The Object to traverse & mutate with a value.
Type: String
or Array
The key path that should receive the value. May be in x.y.z
or ['x', 'y', 'z']
formats.
Note: Please be aware that only the last key actually receives the value!
Important: New Objects are created at each segment if there is not an existing structure.
However, when integers are encounted, Arrays are created instead!
Type: Any
The value that you want to set. Can be of any type!
For benchmarks and full results, check out the bench
directory!
# Node 10.13.0
Validation:
✔ set-value
✔ lodash/set
✔ dset
Benchmark:
set-value x 1,701,821 ops/sec ±1.81% (93 runs sampled)
lodash/set x 975,530 ops/sec ±0.96% (91 runs sampled)
dset x 1,797,922 ops/sec ±0.32% (94 runs sampled)
dset
in 182 bytesMIT © Luke Edwards
FAQs
A tiny (194B) utility for safely writing deep Object values~!
We found that dset demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Dutch National Police and FBI dismantle Redline and Meta infostealer malware-as-a-service operations in Operation Magnus, seizing servers and source code.
Research
Security News
Socket is tracking a new trend where malicious actors are now exploiting the popularity of LLM research to spread malware through seemingly useful open source packages.
Security News
Research
Noxia, a new dark web bulletproof host, offers dirt cheap servers for Python, Node.js, Go, and Rust, enabling cybercriminals to distribute malware and execute supply chain attacks.