easy-baileys π€

easy-baileys is a Node.js package designed to streamline WhatsApp connectivity and automate message handling, offering robust flexibility. It leverages the powerful capabilities of @whiskeysockets/baileys.
β¨ GIVE A STAR ON GITHUB β
easy-baileys
IF YOU FOUND ANY ISSUE OR NEED A NEW FEATURES FEEL FREE TO OPEN A ISSUE ON GITHUB
easy-baileys
Installation π¦
npm install easy-baileys
Usage π οΈ
1. Importing the Module
const { WhatsAppClient } = require('easy-baileys');
2. Creating a Client Instance with MongoDB Authentication (+ Multiple Session β
)
const { WhatsAppClient } = require('easy-baileys');
const customOptions = {
browser: ["Ubuntu", "Chrome", "20.0.04"],
printQRInTerminal: true,
mobile: false,
};
async function main() {
try {
const clientMongo = await WhatsAppClient.create("mongo", 'YOUR MONGO DB URI', customOptions);
const sockMongo = await clientMongo.getSocket();
sockMongo.ev.on("messages.upsert", async ({ messages }) => {
for (const m of messages) {
console.log(m)
if (m.message?.conversation.toLowerCase() === 'hi') {
await sockMongo.reply(m, 'Hello! π');
sockMongo.sendImage()
}
}
});
} catch (error) {
console.error('Error initializing WhatsApp client with MongoDB authentication:', error.message);
}
}
main()
3. Creating a Client Instance with MySQL Authentication (+ Multiple Session β
)
const { WhatsAppClient } = require('easy-baileys');
const mysqlConfig = {
session: 'session1',
host: 'localhost',
port: 3306,
user: 'your_mysql_user',
password: 'your_mysql_password',
database: 'your_database_name',
tableName: 'auth'
};
const customOptions = {
browser: ["Ubuntu", "Chrome", "20.0.04"],
printQRInTerminal: false,
mobile: false,
};
(async () => {
try {
const client = await WhatsAppClient.create("mysql", mysqlConfig, customOptions);
const sockMySQL = await client.getSocket();
sockMySQL.ev.on("messages.upsert", async ({ messages }) => {
for (const m of messages) {
if (m.message?.conversation.toLowerCase() === 'hi') {
await sockMySQL.reply(m, 'Hello! π');
}
}
});
} catch (error) {
console.error('Error initializing WhatsApp client with MySQL authentication:', error.message);
}
})();
4. Creating a Client Instance with MultiFile Authentication
const { WhatsAppClient } = require('easy-baileys');
const customOptions = {
browser: ["Ubuntu", "Chrome", "20.0.04"],
printQRInTerminal: false,
mobile: false,
};
(async () => {
try {
const clientMulti = await WhatsAppClient.create("multi", './authFiles', customOptions);
const sockMulti = await clientMulti.getSocket();
sockMulti.ev.on("messages.upsert", async ({ messages }) => {
for (const m of messages) {
if (m.message?.conversation.toLowerCase() === 'hi') {
await sockMulti.reply(m, 'Hello! π');
}
}
});
} catch (error) {
console.error('Error initializing WhatsApp client with MultiFile authentication:', error.message);
}
})();
Explanation
- MongoDB Authentication Example: Initializes the
WhatsAppClient
instance using MongoDB credentials and sets up event listeners to respond to messages. - MultiFile Authentication Example: Initializes the
WhatsAppClient
instance using authentication files stored locally and handles incoming messages similarly.
5. Obtain a pairing code (Optional)
const sock = client.getSocket();
const code = await client.getPairingCode(123456789);
console.log(code);
Example: Pairing Code with Validated Phone Number π
const { WhatsAppClient } = require('easy-baileys');
(async () => {
try {
const customOptions = {
browser: ["Ubuntu", "Chrome", "20.0.04"],
printQRInTerminal: false,
mobile: false,
};
const client = await WhatsAppClient.createMultiAuth('./auth', customOptions);
const sock = await client.getSocket();
const code = await client.getPairingCode(123456789);
console.log(code);
} catch (error) {
console.error('Error initializing WhatsApp client:', error.message);
}
})();
Example: Display QR Code in Terminal π±
(async () => {
try {
const customOptions = {
printQRInTerminal: true,
};
const client = await WhatsAppClient.createMultiAuth('./auth', customOptions);
const sock = await client.getSocket();
} catch (error) {
console.error('Error initializing WhatsApp client:', error.message);
}
})();
The QR code will be printed directly in your terminal.
Simple Example Code
const { WhatsAppClient } = require('easy-baileys');
(async () => {
try {
const customOptions = {
browser: ["Ubuntu", "Chrome", "20.0.04"],
printQRInTerminal: true,
mobile: false,
};
const client = await WhatsAppClient.createMultiAuth('./hacxk', customOptions);
const conn = await client.getSocket();
conn.ev.on("messages.upsert", async ({ messages }) => {
for (const m of messages) {
if (m.message?.conversation.toLowerCase() === 'hi') {
await conn.reply(m, 'Hello! π');
}
}
});
} catch (error) {
console.error('Error initializing WhatsApp client:', error.message);
}
})();```
Handling Message Upserts
One of the key features of @whiskeysockets/baileys
is the ability to handle incoming messages. The library provides an event called messages.upsert
that you can listen to for new messages. Hereβs how you can set up a listener for this event:
sock.ev.on("messages.upsert", async ({ messages }) => {
for (const m of messages) {
console.log(m);
}
});
Real-World Use Case
In a real-world scenario, you might want to do more than just log the messages. Here's an extended example that checks if the message contains text and replies to the sender:
sock.ev.on("messages.upsert", async ({ messages }) => {
for (const m of messages) {
console.log(m);
if (m.message && m.message.conversation) {
const sender = m.key.remoteJid;
const messageText = m.message.conversation;
console.log(`Message from ${sender}: ${messageText}`);
}
}
});
Overview: π±π¬
The connMessage
class provides methods to interact with WhatsApp messages, including sending various media types, replying, reacting, editing, and deleting messages.
Function | Parameters | Description |
---|
sendSticker | m , bufferOrUrl | Sends a sticker message. |
sendStickerReply | m , bufferOrUrl | Sends a sticker as a reply to a message. |
sendImage | m , bufferOrUrl , caption | Sends an image message with an optional caption. |
sendImageReply | m , bufferOrUrl , caption | Sends an image as a reply to a message. |
sendVideo | m , bufferOrUrl , caption | Sends a video message with an optional caption. |
sendVideoReply | m , bufferOrUrl , caption | Sends a video as a reply to a message. |
sendDocument | m , bufferOrUrl , mimetype , fileName , caption | Sends a document message. |
sendDocumentReply | m , bufferOrUrl , mimetype , fileName , caption | Sends a document as a reply to a message. |
sendAudio | m , bufferOrUrl , ptt | Sends an audio message, optionally as push-to-talk. |
sendAudioReply | m , bufferOrUrl , ptt | Sends an audio message as a reply, optionally as push-to-talk. |
sendGif | m , bufferOrUrl , playback | Sends a GIF message. |
sendGifReply | m , bufferOrUrl , playback | Sends a GIF as a reply to a message. |
reply | m , text | Replies to a message with text. |
send | m , text | Sends a text message. |
react | m , emoji | Reacts to a message with an emoji. |
editMsg | m , sentMessage , newMessage | Edits a sent message. |
deleteMsgGroup | m | Deletes a message in a group chat. |
deleteMsg | m | Deletes a message. |
findValue | obj , targetValue , currentPath | Recursively finds a path to a value in an object. |
findObject | obj , targetValue | Recursively finds an object containing a specific value. |
add | groupJid , participantJid | Adds a participant to a group. |
remove | groupJid , participantJid | Removes a participant from a group. |
isAdmin | groupJid | Checks if the bot is an admin in a group. |
updateParticipantStatus | groupJid , participantJid , action | Promotes or demotes a participant in a group. |
updateGroupSettings | groupJid , settings | Updates group settings. |
banUser | groupJid , userJid | Bans a user from joining a group. |
unbanUser | groupJid , userJid | Unbans a user from a group. |
generateInviteLink | groupJid | Generates a new invite link for a group. |
revokeInviteLink | groupJid | Revokes the current invite link for a group. |
updateGroupSubject | groupJid , newSubject | Updates the group subject (name). |
updateGroupDescription | groupJid , newDescription | Updates the group description. |
updateGroupMessagesSettings | groupJid , setting | Updates who can send messages in the group. |
scheduleMessage | jid , content , sendTime | Schedules a message to be sent at a specific time. |
cancelScheduledMessage | index | Cancels a scheduled message. |
sendBulkMessage | jids , content | Sends a message to multiple recipients. |
downloadMedia | m | Downloads media from a message. |
createPoll | groupJid , question , options | Creates a poll in a group chat. |
updateStatus | status | Updates the bot's status. |
Methods
See API Documentation for detailed method explanations.
JAVASCRIPT
-
sendSticker(m, bufferOrUrl)
π¨
-
sendStickerReply(m, bufferOrUrl)
π
-
sendImage(m, bufferOrUrl, caption)
πΌοΈ
-
sendImageReply(m, bufferOrUrl, caption)
π
-
sendVideo(m, bufferOrUrl, caption)
πΉ
-
sendVideoReply(m, bufferOrUrl, caption)
π₯
-
sendDocument(m, bufferOrUrl, mimetype, fileName, caption)
π
- Description: Sends a document (file) message with an optional caption.
- Parameters:
m
(object
): Message object containing chat information.bufferOrUrl
(string
, Buffer
, or filepath
): URL, buffer, or filepath containing the document data.mimetype
(string
): MIME type of the document.fileName
(string
): Name of the file.caption
(string
): Optional caption for the document.
- Usage Example:
await conn.sendDocument(m, 'https://example.com/document.pdf', 'application/pdf', 'document.pdf', 'Check out this document!');
-
sendDocumentReply(m, bufferOrUrl, mimetype, fileName, caption)
π
- Description: Sends a document as a reply to a specific message.
- Parameters: Same as
sendDocument
. - Usage Example:
await conn.sendDocumentReply(m, 'https://example.com/document.pdf', 'application/pdf', 'document.pdf', 'Replying with a document.');
-
sendAudio(m, bufferOrUrl, ptt)
π΅
-
sendAudioReply(m, bufferOrUrl, ptt)
π€
-
sendGif(m, bufferOrUrl, playback)
π¬
-
sendGifReply(m, bufferOrUrl, playback)
ποΈ
-
reply(m, text)
π¬
-
send(m, text)
βοΈ
-
react(m, emoji)
π
-
editMsg(m, sentMessage, newMessage)
π
-
deleteMsgGroup(m)
ποΈ
-
deleteMsg(m)
π«
- Description: finds a path in an object where a specified value is located.
- Parameters: Same as
deleteMsgGroup
. - Usage Example:
await conn.deleteMsg(m);
-
findValue(m, theValue)
π«
add(groupJid, participantJid)
β
Description: Invites a specified participant to join a WhatsApp group. Requires admin privileges.
Parameters:
groupJid
(string): The group's JID (e.g., 1234567890-123456@g.us
).participantJid
(string): The participant's JID (e.g., 9876543210@s.whatsapp.net
).
TYPESCRIPT
ConnMessage Class Documentation Typescript
The ConnMessage
class extends the functionality of the WASocket
class from the @whiskeysockets/baileys
library, providing a variety of methods for sending different types of messages in a WhatsApp chat application.
Table of Contents
- sendTextMessage
- reply
- react
- send
- sendImage
- sendImageReply
- sendVideo
- sendVideoReply
- sendDocument
- sendDocumentReply
- sendSticker
- sendStickerReply
- sendGIF
- sendGIFReply
- sendAudio
- sendAudioReply
- sendContact
- sendContactReply
- sendPoll
- sendPollReply
- editMessage
- deleteMessage
- sendLocation
- sendLocationReply
- sendLiveLocation
- sendButton
- sendListMessage
- sendTemplateMessage
Method Descriptions
sendTextMessage
Sends a simple text message to a specified JID (WhatsApp ID).
Arguments:
jid: string
- The recipient's WhatsApp IDtext: string
- The message text to send
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendTextMessage("1234567890@s.whatsapp.net", "Hello, World!");
reply
Replies to a received message with a text message.
Arguments:
m: proto.IWebMessageInfo
- The received message to reply totext: string
- The reply text
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.reply(receivedMessage, "Thanks for your message!");
react
Sends a reaction to a message using an emoji.
Arguments:
m: proto.IWebMessageInfo
- The message to react toemoji: string
- The emoji to use as a reaction
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.react(receivedMessage, "π");
send
A generic method to send any type of content to a specified JID.
Arguments:
jid: string
- The recipient's WhatsApp IDcontent: AnyMessageContent
- The content to sendoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.send("1234567890@s.whatsapp.net", { text: "Hello" }, { ephemeralExpiration: 86400 });
sendImage
Sends an image to a specified JID.
Arguments:
jid: string
- The recipient's WhatsApp IDimage: WAMediaUpload
- The image to sendcaption?: string
- Optional caption for the imageoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendImage("1234567890@s.whatsapp.net", "./image.jpg", "Check out this picture!");
sendImageReply
Sends an image as a reply to a received message.
Arguments:
m: proto.IWebMessageInfo
- The received message to reply toimage: WAMediaUpload
- The image to sendcaption?: string
- Optional caption for the imageoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendImageReply(receivedMessage, "./image.jpg", "Here's the image you requested.");
sendVideo
Sends a video to a specified JID.
Arguments:
jid: string
- The recipient's WhatsApp IDvideo: WAMediaUpload
- The video to sendcaption?: string
- Optional caption for the videooptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendVideo("1234567890@s.whatsapp.net", "./video.mp4", "Check out this video!");
sendVideoReply
Sends a video as a reply to a received message.
Arguments:
m: proto.IWebMessageInfo
- The received message to reply tovideo: WAMediaUpload
- The video to sendcaption?: string
- Optional caption for the videooptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendVideoReply(receivedMessage, "./video.mp4", "Here's the video you asked for.");
sendDocument
Sends a document to a specified JID.
Arguments:
jid: string
- The recipient's WhatsApp IDdocument: WAMediaUpload
- The document to sendfilename: string
- The filename for the documentmimeType: string
- The MIME type of the documentcaption?: string
- Optional caption for the documentoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendDocument("1234567890@s.whatsapp.net", "./document.pdf", "report.pdf", "application/pdf", "Monthly Report");
sendDocumentReply
Sends a document as a reply to a received message.
Arguments:
m: proto.IWebMessageInfo
- The received message to reply todocument: WAMediaUpload
- The document to sendfilename: string
- The filename for the documentmimeType: string
- The MIME type of the documentcaption?: string
- Optional caption for the documentoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendDocumentReply(receivedMessage, "./document.pdf", "report.pdf", "application/pdf", "Here's the report you requested.");
sendSticker
Sends a sticker to a specified JID.
Arguments:
jid: string
- The recipient's WhatsApp IDsticker: WAMediaUpload
- The sticker to sendoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendSticker("1234567890@s.whatsapp.net", "./sticker.webp");
sendStickerReply
Sends a sticker as a reply to a received message.
Arguments:
m: proto.IWebMessageInfo
- The received message to reply tosticker: WAMediaUpload
- The sticker to sendoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendStickerReply(receivedMessage, "./sticker.webp");
sendGIF
Sends a GIF to a specified JID.
Arguments:
jid: string
- The recipient's WhatsApp IDgif: WAMediaUpload
- The GIF to sendcaption?: string
- Optional caption for the GIFoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendGIF("1234567890@s.whatsapp.net", "./animation.gif", "Check out this cool GIF!");
sendGIFReply
Sends a GIF as a reply to a received message.
Arguments:
m: proto.IWebMessageInfo
- The received message to reply togif: WAMediaUpload
- The GIF to sendcaption?: string
- Optional caption for the GIFoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendGIFReply(receivedMessage, "./animation.gif", "Here's a funny GIF for you!");
sendAudio
Sends an audio file to a specified JID.
Arguments:
jid: string
- The recipient's WhatsApp IDaudio: WAMediaUpload
- The audio file to sendoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendAudio("1234567890@s.whatsapp.net", "./audio.mp3");
sendAudioReply
Sends an audio file as a reply to a received message.
Arguments:
m: proto.IWebMessageInfo
- The received message to reply toaudio: WAMediaUpload
- The audio file to sendoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendAudioReply(receivedMessage, "./audio.mp3");
sendContact
Sends a contact card to a specified JID.
Arguments:
jid: string
- The recipient's WhatsApp IDcontact: { name: string, number: string }
- The contact informationoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendContact("1234567890@s.whatsapp.net", { name: "John Doe", number: "+1234567890" });
sendContactReply
Sends a contact card as a reply to a received message.
Arguments:
m: proto.IWebMessageInfo
- The received message to reply tocontact: { name: string, number: string }
- The contact informationoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendContactReply(receivedMessage, { name: "Jane Doe", number: "+9876543210" });
sendPoll
Sends a poll to a specified JID.
Arguments:
jid: string
- The recipient's WhatsApp IDname: string
- The question or title of the pollvalues: string[]
- An array of poll optionsselectableCount?: number
- Optional number of selectable options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendPoll("1234567890@s.whatsapp.net", "What's your favorite color?", ["Red", "Blue", "Green"], 1);
sendPollReply
Sends a poll as a reply to a received message.
Arguments:
m: proto.IWebMessageInfo
- The received message to reply toname: string
- The question or title of the pollvalues: string[]
- An array of poll optionsselectableCount?: number
- Optional number of selectable options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendPollReply(receivedMessage, "What's your favorite fruit?", ["Apple", "Banana", "Orange"], 2);
editMessage
Edits a previously sent message.
Arguments:
jid: string
- The chat's WhatsApp IDm: proto.IWebMessageInfo
- The message to editnewContent: string | { text?: string, caption?: string }
- The new content for the message
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.editMessage("1234567890@s.whatsapp.net", sentMessage, "Updated message content");
deleteMessage
Deletes a message.
Arguments:
jid: string
- The chat's WhatsApp IDm: proto.IWebMessageInfo
- The message to delete
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.deleteMessage("1234567890@s.whatsapp.net", messageToDelete);
sendLocation
Sends a location to a specified JID.
Arguments:
jid: string
- The recipient's WhatsApp IDlatitude: number
- The latitude of the locationlongitude: number
- The longitude of the locationoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendLocation("1234567890@s.whatsapp.net", 40.7128, -74.0060);
sendLocationReply
Sends a location as a reply to a received message.
Arguments:
m: proto.IWebMessageInfo
- The received message to reply tolatitude: number
- The latitude of the locationlongitude: number
- The longitude of the locationoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendLocationReply(receivedMessage, 51.5074, -0.1278);
sendLiveLocation
Sends a live location to a specified JID.
Arguments:
jid: string
- The recipient's WhatsApp IDlatitude: number
- The latitude of the locationlongitude: number
- The longitude of the locationdurationMs: number
- The duration of the live location in millisecondsoptions?: MiscMessageGenerationOptions & { comment?: string }
- Optional message generation options and comment
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const result = await sock.sendLiveLocation("1234567890@s.whatsapp.net", 40.7128, -74.0060, 3600000, { comment: "I'm here!" });
sendButton
Sends a message with buttons to a specified JID.
Arguments:
jid: string
- The recipient's WhatsApp IDcontentText: string
- The main text content of the messagebuttons: proto.Message.ButtonsMessage.IButton[]
- An array of button objectsoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const buttons = [
{ buttonId: '1', buttonText: { displayText: 'Button 1' }, type: 1 },
{ buttonId: '2', buttonText: { displayText: 'Button 2' }, type: 1 },
];
const result = await sock.sendButton("1234567890@s.whatsapp.net", "Please choose an option:", buttons);
sendListMessage
Sends a list message to a specified JID.
Arguments:
jid: string
- The recipient's WhatsApp IDmessage: proto.Message.ListMessage
- The list message objectoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const listMessage: proto.Message.ListMessage = {
title: "Menu",
description: "Please select an item",
buttonText: "View Menu",
listType: 1,
sections: [
{
title: "Section 1",
rows: [
{ title: "Option 1", description: "Description for Option 1" },
{ title: "Option 2", description: "Description for Option 2" },
],
},
],
};
const result = await sock.sendListMessage("1234567890@s.whatsapp.net", listMessage);
sendTemplateMessage
Sends a template message with buttons to a specified JID.
Arguments:
jid: string
- The recipient's WhatsApp IDcontent: Templatable
- The content object containing text, footer, and template buttonsoptions?: MiscMessageGenerationOptions
- Optional message generation options
Returns: Promise<proto.WebMessageInfo | undefined>
Example:
const templateContent: Templatable = {
text: "Hello! Please choose an option:",
footer: "Footer text",
templateButtons: [
{ index: 1, urlButton: { displayText: "Visit Website", url: "https://example.com" } },
{ index: 2, callButton: { displayText: "Call us", phoneNumber: "+1234567890" } },
{ index: 3, quickReplyButton: { displayText: "Quick Reply", id: "quick-reply-id" } },
],
};
const result = await sock.sendTemplateMessage("1234567890@s.whatsapp.net", templateContent);
Example:
try {
await conn.add('1234567890-123456@g.us', '9876543210@s.whatsapp.net');
console.log('Participant added successfully!');
} catch (err) {
console.error(`Failed to add participant: ${err.message}`);
}
remove(groupJid, participantJid)
β
Description: Removes a specified participant from a WhatsApp group. Requires admin privileges.
Parameters:
groupJid
(string): The group's JID (e.g., 1234567890-123456@g.us
).participantJid
(string): The participant's JID (e.g., 9876543210@s.whatsapp.net
).
Example:
try {
await conn.remove('1234567890-123456@g.us', '9876543210@s.whatsapp.net');
console.log('Participant removed successfully!');
} catch (err) {
console.error(`Failed to remove participant: ${err.message}`);
}
Setting Up Commands with easy-baileys
To create and manage commands for your WhatsApp bot using easy-baileys
, follow these steps:
-
Import loadCommands
and getCommand
from easy-baileys
to manage your bot commands:
const { loadCommands, getCommand } = require('easy-baileys');
-
Loading Commands:
Use loadCommands
to load commands from a specified directory. Example:
await loadCommands('./commands');
-
Handling Commands:
Use getCommand
to retrieve and execute commands based on incoming messages. Example:
sock.ev.on("messages.upsert", async ({ messages }) => {
for (const m of messages) {
const { message } = m;
const messageTypes = ['extendedTextMessage', 'conversation', 'imageMessage', 'videoMessage'];
let text = messageTypes.reduce((acc, type) =>
acc || (message[type] && (message[type].text || message[type].caption || message[type])) || '', '');
const response = text.toLowerCase();
const prefix = ['!'];
if (!prefix.some(p => response.startsWith(p))) {
continue;
}
const [commandName, ...args] = response.slice(prefix.length).trim().split(/\s+/);
const command = await getCommand(commandName);
if (!command) {
console.log(`Command not found: ${commandName}`);
continue;
}
try {
await command.execute(sock, m, args);
} catch (cmdError) {
console.error(`Error executing command '${commandName}':`, cmdError.message);
}
}
});
Example Command File Structure
Here's an example structure for a Ping
command (command/Ping.js
):
module.exports = {
usage: ["ping"],
desc: "Checks the bot's response time.",
commandType: "Bot",
isGroupOnly: false,
isAdminOnly: false,
isPrivateOnly: false,
emoji: 'π',
async execute(sock, m) {
try {
const startTime = Date.now();
const latency = Date.now() - startTime;
await sock.reply(m, `π Pong! ${latency}ms`);
} catch (error) {
await sock.reply(m, "β An error occurred while checking the ping: " + error);
}
}
};
Command Metadata Explanation:
usage
: Array of strings defining how users can invoke the command.desc
: Brief description of what the command does.commandType
: Type of command (e.g., Bot, Admin).isGroupOnly
: Whether the command works only in group chats.isAdminOnly
: Whether the command is restricted to admins.isPrivateOnly
: Whether the command can only be used in private chats.emoji
: Emoji associated with the command for visual appeal.
Now you're all set to create and manage commands for your WhatsApp bot using easy-baileys
! πβ¨
Configuration Options βοΈ
browser
: An array specifying the browser information (e.g., ["Ubuntu", "Chrome", "20.0.04"]
).printQRInTerminal
: (Boolean) Display the QR code in the terminal (default: false
).mobile
: (Boolean) Set to true
if connecting from a mobile device.- Refer to the
@whiskeysockets/baileys
documentation for additional options.
Contributing π€
Contributions are welcome! Please feel free to submit issues and pull requests.
Thanks to
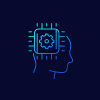
License π
This project is licensed under the MIT License. See the LICENSE file for details.