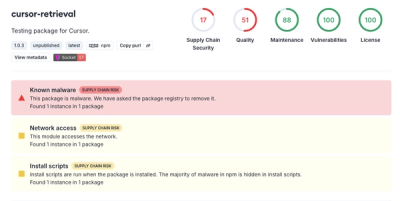
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
effection
Advanced tools
Effortlessly composable structured concurrency primitive for JavaScript
Effortlessly composable structured concurrency primitive for JavaScript
See examples
To run an example with NAME:
$ node -r ./tests/setup examples/NAME
Note: For an general introduction to the concept of structured concurrency, and why it is so important, see this excellent primer on the subject by Nathaniel Smith.
There's an entire hive of bugs that occur when asynchronous processes outlive their welcome. The concept of structured concurrency eliminates these altogether by providing the following guarantees:
For example, if you have the following processes:
+ - parent
|
+ --- child A
|
+ --- child B
We can make the following assertions based on these guarantees :
a. If the code associated with parent
finishes running, but either A
or B
are pending, then parent
is still considered pending.
b. If parent
is halted, then both A
and B
are halted.
c. If an error is raised in parent
, then both A
and B
are
halted.
d. if an error is raised in A
, then that error is also raised in
parent
, and child B
is halted.
scenario d
is of particular importance. It means that if a child
throws an error and its parent doesn't catch it, then all of its
siblings are immediately halted.
The process primitive is the Execution
. To create (and start) an
Execution
, use the execute
function and pass it a generator. This
simplest example waits for 1 second, then prints out "hello world" to
the console.
import { execute, timeout } from 'effection';
let process = execute(function*() {
yield timeout(1000);
return 'hello world';
});
process.isRunning //=> true
// 1000ms passes
// process.isRunning //=> false
// process.result //=> 'hello world'
Child processes can be composed freely. So instead of yielding for 1000 ms, we could instead, yield 10 times for 100ms.
execute(function*() {
yield function*() {
for (let i = 0; i < 10; i++) {
yield timeout(100);
}
}
return 'hello world';
})
And in fact, processes can be easily and arbitrarly deeply nested:
let process = execute(function*() {
return yield function*() {
return yield function*() {
return yield function*() {
return 'hello world';
}
};
};
});
process.isCompleted //=> true
process.result //=> "hello world"
In order to abstract a process so that it can take arguments, you can
use the call
function:
import { execute, timeout, call } from 'effection';
function* waitForSeconds(durationSeconds) {
yield timeout(durationSeconds * 1000);
}
execute(function*() {
yield call(waitforseconds, 10);
});
More likely though, you would want to define a higher-order function that took your argument and returned a generator:
function waitForSeconds(durationSeconds) {
return function*() {
yield timeout(durationSeconds * 1000);
}
}
Sometimes you want to execute some processes in parallel and not
necessarily block further execution on them. You still want the
guarantees associated with structured concurrency however. For
example, you might want to create a couple of different servers as
part of your main process. To do this, you would use the fork
method
on the execution:
import { execute } from 'effection';
execute(function*() {
this.fork(createFileServer);
this.fork(createHttpServer);
});
Even though it exits almost immediately, the main process is not considered completed until both servers shutdown. More importantly though, if we shutdown the main process, then both servers will be halted.
yarn install
$ yarn
run tests:
$ yarn test
FAQs
Structured concurrency and effects for JavaScript
The npm package effection receives a total of 3,172 weekly downloads. As such, effection popularity was classified as popular.
We found that effection demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.