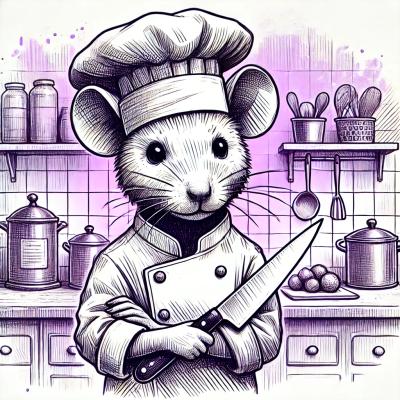
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
embla-carousel
Advanced tools
Embla Carousel is a lightweight, extendable, and customizable carousel library for modern web applications. It provides a simple API for creating responsive and touch-friendly carousels with various customization options.
Basic Carousel Setup
This code initializes a basic Embla Carousel on an HTML element with the class 'embla'.
const emblaNode = document.querySelector('.embla');
const embla = EmblaCarousel(emblaNode);
Custom Options
This code demonstrates how to initialize an Embla Carousel with custom options such as looping and speed.
const emblaNode = document.querySelector('.embla');
const options = { loop: true, speed: 10 };
const embla = EmblaCarousel(emblaNode, options);
Adding Plugins
This code shows how to add plugins to the Embla Carousel, such as the Autoplay plugin for automatic sliding.
import EmblaCarousel from 'embla-carousel';
import Autoplay from 'embla-carousel-autoplay';
const emblaNode = document.querySelector('.embla');
const embla = EmblaCarousel(emblaNode, { loop: true }, [Autoplay()]);
Event Handling
This code demonstrates how to handle events in Embla Carousel, such as logging the selected slide index when a slide is selected.
const emblaNode = document.querySelector('.embla');
const embla = EmblaCarousel(emblaNode);
embla.on('select', () => {
console.log('Slide selected:', embla.selectedScrollSnap());
});
Swiper is a modern touch slider with hardware-accelerated transitions and a wide range of features. It is highly customizable and supports various effects, autoplay, and responsive breakpoints. Compared to Embla Carousel, Swiper offers more built-in features and effects but may be heavier in terms of bundle size.
Slick Carousel is a popular and fully-featured carousel library that supports multiple breakpoints, lazy loading, and various transition effects. It is easy to set up and use but may not be as lightweight or modular as Embla Carousel.
React Slick is a React wrapper for the Slick Carousel library. It provides the same features as Slick Carousel but is designed specifically for React applications. It offers a simple API for integrating carousels into React components. Compared to Embla Carousel, React Slick is more tailored for React but may not offer the same level of customization and modularity.
Extensible bare bone carousels for the web. Build awesome carousels by extending them with your own CSS and JavaScript. Embla Carousel is dependency free and 100% open source.
NPM
npm install embla-carousel
HTML
<div class="embla">
<div class="embla__container">
<div class="embla__slide">
Slide 1
</div>
<div class="embla__slide">
Slide 2
</div>
<div class="embla__slide">
Slide 3
</div>
<div class="embla__slide">
Slide 4
</div>
</div>
</div>
CSS
.embla {
overflow: hidden;
}
.embla__container {
display: flex;
}
.embla__slide {
position: relative; /* Needed if loop: true */
flex: 0 0 100%; /* Choose any slide width */
}
JavaScript
import EmblaCarousel from 'embla-carousel'
const emblaNode = document.querySelector('.embla')
const options = { loop: true }
const embla = EmblaCarousel(emblaNode, options)
Configure Embla by passing an options object as the second argument. Default values are:
const embla = EmblaCarousel(emblaNode, {
align: 'center',
containerSelector: '*',
slidesToScroll: 1,
containScroll: false,
draggable: true,
dragFree: false,
loop: false,
speed: 10,
startIndex: 0,
selectedClass: 'is-selected',
draggableClass: 'is-draggable',
draggingClass: 'is-dragging',
})
align
start
, center
and end
, you can provide a number to align the slides. For example, if you pass 0.2
, slides will be aligned 20% from the viewport start edge. Note that slide alignments will be overrided for slides at the start and end when used together with
containScroll
,
that prevents excessive scrolling at the beginning or end.
string
| number
start
center
end
number
center
Usage
const options = { align: 'start' }
const embla = EmblaCarousel(emblaNode, options)
containerSelector
string
any
*
Usage
const options = { containerSelector: '.my-container-selector' }
const embla = EmblaCarousel(emblaNode, options)
<div class="embla">
<div class="my-container-selector">
...slides
</div>
</div>
slidesToScroll
2
, every two slides will be treated as a single slide.
number
any
1
Usage
const options = { slidesToScroll: 2 }
const embla = EmblaCarousel(emblaNode, options)
.embla__slide {
flex: 0 0 50%; /* Show 2 slides in viewport */
}
containScroll
boolean
true
false
false
Usage
const options = { containScroll: true }
const embla = EmblaCarousel(emblaNode, options)
draggable
boolean
true
false
true
Usage
const options = { draggable: false }
const embla = EmblaCarousel(emblaNode, options)
dragFree
boolean
true
false
false
Usage
const options = { dragFree: true }
const embla = EmblaCarousel(emblaNode, options)
loop
containScroll
will be ignored if loop is enabled because the empty space is already filled with looping slides.
boolean
true
false
false
Usage
const options = { loop: true }
const embla = EmblaCarousel(emblaNode, options)
.embla__slide {
position: relative; /* Needed for loop to work */
}
speed
scrollNext
,
scrollPrev
and
scrollTo
. Use a higher number for faster scrolling. Drag interactions are not affected by this because the speed in these cases is determined by how vigorous the drag gesture was.
number
any
10
Usage
const options = { speed: 15 }
const embla = EmblaCarousel(emblaNode, options)
startIndex
0
. If slides are mapped to groups with the
slidesToScroll
option, some slides share the same scroll snap index. For example, if it's set to 2
slide one and two will be at index 0, while slide three and four will be at index 1 and so on.
number
any
0
Usage
const options = { startIndex: 3 }
const embla = EmblaCarousel(emblaNode, options)
selectedClass
slidesToScroll
is more than 1
and/or
containScroll
is active, slides are mapped to groups. This means that the selected class will be added to multiple slides at a time.
string
any
is-selected
Usage
const options = { selectedClass: 'my-selected-class' }
const embla = EmblaCarousel(emblaNode, options)
draggableClass
draggable
. Use it to style the carousel accordingly. For example, you can show a grab cursor when a draggable carousel is hovered. If no value is provided it will fall back to is-draggable
.
string
any
is-draggable
Usage
const options = { draggableClass: 'my-draggable-class' }
const embla = EmblaCarousel(emblaNode, options)
.my-draggable-class {
cursor: grab;
}
draggingClass
draggable
. Use it to style the carousel accordingly. For example, you can show a grabbing cursor when a pointer is down. If no value is provided it will fall back to is-dragging
.
string
any
is-dragging
Usage
const options = { draggingClass: 'my-dragging-class' }
const embla = EmblaCarousel(emblaNode, options)
.my-dragging-class {
cursor: grabbing;
}
Embla exposes API methods that can be used to control the carousel externally. Example usage:
embla.scrollNext()
embla.scrollTo(2)
embla.changeOptions({ loop: true })
embla.on('select', () => {
console.log(`Selected snap index is ${embla.selectedScrollSnap()}.`)
})
containerNode
containerSelector
option this will return the matching element, otherwise it will return the first immediate child of the Embla node passed to EmblaCarousel.
none
Node.ELEMENT_NODE
Usage
const embla = EmblaCarousel(emblaNode, options)
const emblaContainer = embla.containerNode()
slideNodes
none
Node.ELEMENT_NODE[]
Usage
const embla = EmblaCarousel(emblaNode, options)
const emblaSlides = embla.slideNodes()
scrollNext
loop
option is disabled and the carousel is on the last snap point, this method will do nothing. When loop is enabled, it will always be able to scroll to the next snap point. Useful for creating a scroll next button for example.
none
undefined
Usage
const embla = EmblaCarousel(emblaNode, options)
const nextButton = emblaNode.querySelector('.embla__next')
nextButton.addEventListener('click', embla.scrollNext, false)
<button class="embla__next" type="button">
Scroll Next
</button>
scrollPrev
loop
option is disabled and the carousel is on the first snap point, this method will do nothing. When loop is enabled, it will always be able to scroll to the previous snap point. Useful for creating a scroll previous button for example.
none
undefined
Usage
const embla = EmblaCarousel(emblaNode, options)
const prevButton = emblaNode.querySelector('.embla__prev')
prevButton.addEventListener('click', embla.scrollPrev, false)
<button class="embla__prev" type="button">
Scroll Previous
</button>
scrollTo
loop
option is enabled, the carousel will seek the closest way to the target. Useful for creating dot navigation together with the
scrollSnapList
method.
index: number
undefined
Usage
const embla = EmblaCarousel(emblaNode, options)
const rewindButton = emblaNode.querySelector('.embla__rewind')
rewindButton.addEventListener('click', () => embla.scrollTo(0), false)
<button class="embla__rewind" type="button">
Rewind
</button>
scrollToProgress
scrollProgress
from 0 to 1 by directly setting it. For example, assuming that the carousel is positioned on the first snap point, 0.5
will scroll the carousel half of its scrollable length. Scroll to target is smooth. The second parameter allows for snapping the carousel to the closest snap point based on the target scroll progress (note that this will alter the desired progress a bit in order to snap it).
progress: number
snap: boolean
undefined
Usage
const embla = EmblaCarousel(emblaNode, options)
embla.scrollToProgress(0.5)
scrollBy
scrollProgress
from 0 to 1 by either adding to it or subtracting from it. For example, assuming that the carousel is positioned on the first snap point, 0.5
will scroll the carousel half of its scrollable length. Scroll to target is smooth. The second parameter allows for snapping the carousel to the closest snap point based on the target scroll progress (note that this will alter the desired progress a bit in order to snap it).
progress: number
snap: boolean
undefined
Usage
const embla = EmblaCarousel(emblaNode, options)
embla.scrollBy(0.5)
canScrollPrev
loop
option is enabled it will always return true. For example, it can be used to disable or enable a scroll to previous button.
none
boolean
Usage
const embla = EmblaCarousel(emblaNode, options)
const prevButton = emblaNode.querySelector('.embla__prev')
const togglePrevButtonEnabled = () => {
if (embla.canScrollPrev()) {
prevButton.removeAttribute('disabled')
} else {
prevButton.setAttribute('disabled', 'disabled')
}
}
embla.on('init', togglePrevButtonEnabled)
embla.on('select', togglePrevButtonEnabled)
<button class="embla__prev" type="button">
Scroll Previous
</button>
canScrollNext
loop
option is enabled it will always return true. For example, it can be used to disable or enable a scroll to next button.
none
boolean
Usage
const embla = EmblaCarousel(emblaNode, options)
const nextButton = emblaNode.querySelector('.embla__next')
const toggleNextButtonEnabled = () => {
if (embla.canScrollNext()) {
nextButton.removeAttribute('disabled')
} else {
nextButton.setAttribute('disabled', 'disabled')
}
}
embla.on('init', toggleNextButtonEnabled)
embla.on('select', toggleNextButtonEnabled)
<button class="embla__next" type="button">
Scroll Next
</button>
selectedScrollSnap
slidesToScroll
option is more than 1
some slides will be grouped together and share the same index. For example, when it's set to 2
, every two slides will share the same index. In this case, slide 1
and 2
will share index 0
and slide 3
and 4
will share index 1
and so on.
none
number
Usage
const embla = EmblaCarousel(emblaNode, options)
embla.on('select', () => {
const currentSnapIndex = embla.selectedScrollSnap()
alert(`Selected index has changed to ${currentSnapIndex}.`)
})
previousScrollSnap
slidesToScroll
option is more than 1
some slides will be grouped together and share the same index. For example, when it's set to 2
, every two slides will share the same index. In this case, slide 1
and 2
will share index 0
and slide 3
and 4
will share index 1
and so on.
none
number
Usage
const embla = EmblaCarousel(emblaNode, options)
embla.on('select', () => {
const previousSnapIndex = embla.previousScrollSnap()
alert(`Previously selected index was ${previousSnapIndex}.`)
})
scrollSnapList
slideNodes
and slideIndexes
. For example, it's useful for getting the snap point count or creating a dot navigation together with the
scrollTo
method.
none
scrollSnap[]
Usage
const embla = EmblaCarousel(emblaNode, options)
const scrollSnaps = embla.scrollSnapList()
const slidesInFirstScrollSnap = scrollSnaps[0].slideNodes
const indexesInFirstScrollSnap = scrollSnaps[0].slideIndexes
scrollProgress
0
at the beginning to 1
at the end. For example, it's useful for creating a progress bar together with the
scroll
event. When invoking scrollProgress
without the target parameter, the carousel returns the scroll progress of its current location. However, if you want to grab the target scroll progress the target parameter has to be true
.
target: boolean
number
Usage
const embla = EmblaCarousel(emblaNode, options)
embla.on('scroll', () => {
const scrollPercentage = embla.scrollProgress() * 100
console.log(`The carousel has scrolled ${scrollPercentage}%.`)
})
clickAllowed
true
if a drag interaction in any direction didn't occur before the mouse was released. Touch events also require the carousel to not be in a scrolling state in order to accept the click.
none
boolean
Usage
const embla = EmblaCarousel(emblaNode, options)
const emblaSlides = embla.slideNodes()
const alertClickedSlide = index => {
return () => {
if (embla.clickAllowed()) {
alert(`Slide with index ${index} was clicked.`)
}
}
}
emblaSlides.forEach((slide, index) => {
const alertClickedSlideIndex = alertClickedSlide(index)
slide.addEventListener('click', alertClickedSlideIndex, false)
})
changeOptions
options:
EmblaOptions
undefined
Usage
const embla = EmblaCarousel(emblaNode, options)
embla.changeOptions({ loop: true })
destroy
none
undefined
Usage
const embla = EmblaCarousel(emblaNode, options)
embla.destroy()
on
events
. For example, it's useful for changing styles whenever a new target snap point has been selected or when the carousel is scrolling. Use it together with the
off
method to remove added event listeners without destroying the carousel. However, when the
destroy
method is invoked, any added event listeners will be destroyed.
event: EmblaEvent
callback: function
undefined
Usage
const embla = EmblaCarousel(emblaNode, options)
const onInitCallback = () => {
console.log('The carousel is ready to rock.')
}
embla.on('init', onInitCallback)
off
events
. It's useful for removing added event listeners without destroying the carousel. Note that you don't have to remove event listeners added using the
on
method when invoking
destroy
, because it will destroy all added event listeners for you.
event: EmblaEvent
callback: function
undefined
Usage
const embla = EmblaCarousel(emblaNode, options)
const logIndex = () => {
const selectedIndex = embla.selectedScrollSnap()
console.log(`Selected index has changed to ${selectedIndex}.`)
}
const addLogIndexListener = () => embla.on('select', logIndex)
const removeLogIndexListener = () => embla.off('select', logIndex)
Embla exposes custom events that can be hooked on to. Example usage:
embla.on('select', () => {
console.log(`Selected snap index is ${embla.selectedScrollSnap()}.`)
})
embla.on('scroll', () => {
console.log(`Scroll progress is ${embla.scrollProgress()}.`)
})
init
API
is ready to use. Note that the init event only fires once upon the first initialisation and won't trigger when invoking
changeOptions
or similar.
Usage
const embla = EmblaCarousel(emblaNode, options)
const onInitCallback = () => {
console.log('The carousel is ready to rock.')
})
embla.on('init', onInitCallback)
destroy
Usage
const embla = EmblaCarousel(emblaNode, options)
select
Usage
const embla = EmblaCarousel(emblaNode, options)
scroll
Usage
const embla = EmblaCarousel(emblaNode, options)
embla.on('scroll', () => {
const scrollPercentage = embla.scrollProgress() * 100
console.log(`The carousel has scrolled ${scrollPercentage}%.`)
})
settle
Usage
const embla = EmblaCarousel(emblaNode, options)
embla.on('settle', () => {
console.log(`The carousel has stopped scrolling.`)
})
resize
Usage
const embla = EmblaCarousel(emblaNode, options)
dragStart
Usage
const embla = EmblaCarousel(emblaNode, options)
dragEnd
Usage
const embla = EmblaCarousel(emblaNode, options)
Get started instantly with one of the CodeSandboxes below.
Basic Setup
- With Previous, Next & Dot buttons.
Autoplay
- Example of how to setup Autoplay.
Embla has been tested in the browsers listed below. Special thanks goes to BrowserStack.
IE - 11
Edge - Latest 2 versions
Chrome - Latest 2 versions
Firefox - Latest 2 versions
Safari - Latest 2 versions
Thank you to all contributors for making Embla Carousel awesome! Contributions are welcome.
Copyright © 2019-present, David Cetinkaya.
Embla is MIT licensed 💖
· · ·
FAQs
A lightweight carousel library with fluid motion and great swipe precision
The npm package embla-carousel receives a total of 1,059,226 weekly downloads. As such, embla-carousel popularity was classified as popular.
We found that embla-carousel demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.