TypeScript types for Enonic XP

This library contains TypeScript types for Enonic XP. You can get an introduction to usage in this recording from the
Enonic Meetup 13 Apr 2021:
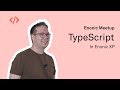
Installing enonic-types
Install enonic-types from npm by running:
npm i --save-dev enonic-types
Add support to use typed import
updating your tsconfig.json file (or src/main/resources/tsconfig.server.json if
you are using the webpack-starter) with the "types"
field:
{
"compilerOptions": {
"types": ["node", "enonic-types"]
}
}
And you're ready to start coding!
Code generation
We recommend using this library together with the xp-codegen-plugin Gradle plugin. xp-codegen-plugin will create TypeScript interfaces
for your content-types. Those interfaces will be very useful together with this library.
Example
We have an Enonic service that returns an article by id.
import { Article } from "../../site/content-types/article/article";
import * as contentLib from "/lib/xp/content";
export function get(req: XP.Request): XP.Response {
const content = contentLib.get<Article>({
key: req.params.id!
});
if (content !== null) {
const article: Article = content.data;
return {
status: 200,
body: article
}
} else {
return {
status: 404
};
}
}
- We import an
interface Article { ... }
generated by xp-codegen-plugin. - If you added enonic-types to the types in your tsconfig.json or tsconfig.server.json (see above), TypeScript should now
be able to add types to all the standard XP-libraries.
- We use
XP.Request
and XP.Response
to control the shape of our controller. content
is of the type Content<Article> | null
, so we have to do a null check before proceeding.
Using __non_webpack_require__
If your project is using __non_webpack_require__
, you should update your types.ts file to add type support to it.
If you add (or replace the existing)
__non_webpack_require__()
function with the following code, it will automatically look up the correct interfaces for
Enonic XP-libraries.
type LibMap = import("enonic-types/libs").EnonicLibraryMap;
declare const __non_webpack_require__: <K extends keyof LibMap | string = string>(path: K) => K extends keyof LibMap
? LibMap[K]
: any;
Supported libraries