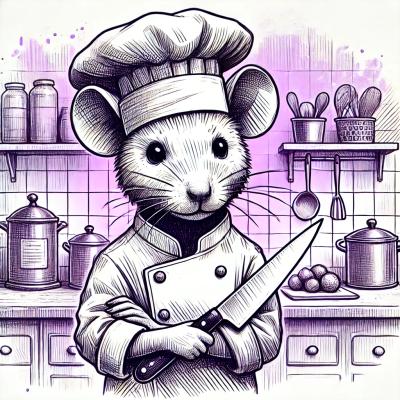
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
env-to-object
Advanced tools
Maps environment variables to a configuration object.
$ npm install env-to-object
var env = require( 'env-to-object' );
Maps environment variables to a configuration object
.
var map = {
'NODE_ENV': {
'keypath': 'env',
'type': 'string'
},
'PORT': {
'keypath': 'server.port',
'type': 'number'
},
'SSL': {
'keypath': 'server.ssl',
'type': 'boolean'
},
'LOGLEVEL': {
'keypath': 'logger.level',
'type': 'string'
}
};
var out = env( map );
/*
{
'env': <string>,
'server': {
'port': <number>,
'ssl': <boolean>
},
'logger': {
'level': <string>
}
}
*/
An environment variable mapping must include a keypath
, which is a dot-delimited object
path. By default, this module parses an environment variable value as a string
. The following types are supported:
The function
accepts the following options
:
parsers: an object
containing environment variable parsers. Each key
should correspond to a defined type
, and each value
should be a function
which accepts an environment variable value and any associated options
.
var map = {
'CUSTOM_TYPE': {
'keypath': 'custom',
'type': 'custom',
... // => options
}
};
var parsers = {
'custom': custom
};
function custom( str, opts ) {
var v = parseInt( str, 10 );
if ( v !== v ) {
return new TypeError( 'invalid value. Value must be an integer. Value: `' + str + '`.' );
}
return v * 6;
}
process.env[ 'CUSTOM_TYPE' ] = '5';
var out = env( map, parsers );
/*
{
'custom': 30
}
*/
(default) Coerce an environment variable value to a string
.
var map = {
'STR': {
'keypath': 'str',
'type': 'string'
}
};
process.env[ 'STR' ] = 'beep';
var out = env( map );
/*
{
'str': 'beep'
}
*/
process.env[ 'STR' ] = '1234';
var out = env( map );
/*
{
'str': '1234'
}
*/
===
Coerce an environment variable value to a number
.
var map = {
'NUM': {
'keypath': 'num',
'type': 'number'
}
};
process.env[ 'NUM' ] = '3.14';
var out = env( map );
/*
{
'num': 3.14
}
*/
process.env[ 'NUM' ] = 'bop';
var out = env( map );
// => throws
===
Coerce an environment variable value to an integer
.
var map = {
'INT': {
'keypath': 'int',
'type': 'integer'
}
};
process.env[ 'INT' ] = '2';
var out = env( map );
/*
{
'int': 2
}
*/
process.env[ 'INT' ] = 'beep';
var out = env( map );
// => throws
The integer
type has the following options
:
radix: an integer
on the interval [2,36]
.
var map = {
'INT': {
'keypath': 'int',
'type': 'integer',
'radix': 2
}
};
process.env[ 'INT' ] = '1';
var out = env( map );
/*
{
'int': 1
}
*/
process.env[ 'INT' ] = '2';
var out = env( map );
// => throws
===
Coerce an environment variable value to a boolean
. The boolean
type supports the following options:
boolean
indicating whether to accept only 'true'
and 'false'
as acceptable boolean
strings. Default: false
.In non-strict mode, the following values are supported:
TRUE
, True
, true
, T
, t
FALSE
, False
, false
, F
, f
var map = {
'BOOL': {
'keypath': 'bool',
'type': 'boolean'
}
};
process.env[ 'BOOL' ] = 'TRUE';
var out = env( map );
/*
{
'bool': true
}
*/
process.env[ 'BOOL' ] = 'beep';
var out = env( map );
// => throws
To restrict the set of allowed values, set the strict
option to true
.
var map = {
'BOOL': {
'keypath': 'bool',
'type': 'boolean',
'strict': true
}
};
process.env[ 'BOOL' ] = 'false';
var out = env( map );
/*
{
'bool': false
}
*/
process.env[ 'BOOL' ] = 'TRUE';
var out = env( map );
// => throws
===
Parse an environment variable value as a JSON object
. Note that a value must be valid JSON.
var map = {
'OBJ': {
'keypath': 'obj',
'type': 'object'
}
};
process.env[ 'OBJ' ] = '{"beep":"boop"}';
var out = env( map );
/*
{
'obj': {
'beep': 'boop'
}
}
*/
process.env[ 'OBJ' ] = '[1,2,3,"4",null]';
var out = env( map );
/*
{
'obj': [ 1, 2, 3, '4', null ]
}
*/
process.env[ 'OBJ' ] = '{"beep:"boop"}';
var out = env( map );
// => throws
===
Coerce an environment variable to a Date
object.
var map = {
'DATE': {
'keypath': 'date',
'type': 'date'
}
};
process.env[ 'DATE' ] = '2015-10-17';
var out = env( map );
/*
{
'date': <Date>
}
*/
process.env[ 'DATE' ] = 'beep';
var out = env( map );
// => throws
===
Parse an environment variable as a RegExp
.
var map = {
'REGEXP': {
'keypath': 're',
'type': 'regexp'
}
};
process.env[ 'RE' ] = '/\\w+/';
var out = env( map );
/*
{
're': /\w+/
}
*/
process.env[ 'RE' ] = 'beep';
var out = env( map );
// => throws
If an environment variable does not exist, the corresponding configuration keypath
will not exist in the output object
.
var map = {
'UNSET_ENV_VAR': {
'keypath': 'a.b.c'
}
};
var out = env( map );
// returns {}
var env = require( 'env-to-object' );
var map = {
'DEFAULT': {
'keypath': 'default'
},
'STR': {
'keypath': 'str',
'type': 'string'
},
'NUM': {
'keypath': 'num',
'type': 'number'
},
'BOOL': {
'keypath': 'bool',
'type': 'boolean',
'strict': true
},
'ARR': {
'keypath': 'arr',
'type': 'object'
},
'NESTED': {
'keypath': 'a.b.c.d',
'type': 'object'
},
"DATE": {
"keypath": "date",
"type": "date"
},
"REGEX": {
"keypath": "re",
"type": "regexp"
},
"INT": {
"keypath": "int",
"type": "integer"
}
};
process.env[ 'DEFAULT' ] = 'beep';
process.env[ 'STR' ] = 'boop';
process.env[ 'NUM' ] = '1234.5';
process.env[ 'BOOL' ] = 'true';
process.env[ 'ARR' ] = '[1,2,3,4]';
process.env[ 'NESTED' ] = '{"hello":"world"}';
process.env[ 'DATE' ] = '2015-10-18T07:00:01.000Z';
process.env[ 'REGEX'] = '/\\w+/';
process.env[ 'INT' ] = '1234';
var out = env( map );
/*
{
'default': 'beep',
'str': 'boop',
'num': 1234.5,
'bool': true,
'arr': [1,2,3,4],
'a': {
'b': {
'c': {
'd': {
'hello': 'world'
}
}
}
},
'date': <date>,
're': /\w+/,
'int': 1234
}
*/
To run the example code from the top-level application directory,
$ node ./examples/index.js
or, alternatively,
$ DEFAULT=boop STR=beep NUM='5432.1' BOOL='false' ARR='[4,3,2,1]' NESTED='{"world":"hello"}' DATE='2015-10-19T06:59:59.000Z' REGEX='/\\.+/' node ./examples/index.js
Unit tests use the Mocha test framework with Chai assertions. To run the tests, execute the following command in the top-level application directory:
$ make test
All new feature development should have corresponding unit tests to validate correct functionality.
This repository uses Istanbul as its code coverage tool. To generate a test coverage report, execute the following command in the top-level application directory:
$ make test-cov
Istanbul creates a ./reports/coverage
directory. To access an HTML version of the report,
$ make view-cov
Copyright © 2015. Athan Reines.
FAQs
Maps environment variables to a configuration object.
The npm package env-to-object receives a total of 8 weekly downloads. As such, env-to-object popularity was classified as not popular.
We found that env-to-object demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.