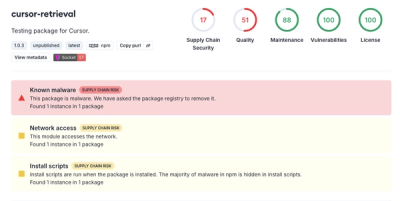
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
A lightweight drop-in Error
replacement with take-it-or-leave-it HTTP status code support.
Useful for preserving associations between errors and their appropriate HTTP status codes when decoupling application logic from middleware plumbing.
# npm
npm i errdrop
# yarn
yarn add errdrop
const Error = require('errdrop')
// Good practice to decouple application logic
// from middleware plumbing (req/res)
function sayHello(name) {
switch (name) {
case 'Dwight':
throw new Error.Forbidden(`Go away, ${name}.`)
case 'Michael':
throw new Error.MovedPermanently()
default:
return `Hello, ${name}!`
}
}
app.get('/say-hello/:name', (req, res) => {
try {
res.send(sayHello(req.params.name))
}
catch (err) {
res.status(err.statusCode || 500).send(err.message)
}
})
Subclasses are generated for each entry in http.STATUS_CODES
. See here for the full list.
These classes pass all arguments through to Error
for full drop-in compatibility.
Custom or nonstandard status codes are supported via the generic Error.StatusCode
class, which prepends the Error
class signature with a status code parameter:
throw new Error.StatusCode(218, 'This is fine')
thinware
supportThis module pairs well with thinware, which honors status codes attached to thrown Error
objects:
/*
/say-hello/Dwight => 403 Forbidden "Go away, Dwight."
/say-hello/Michael => 301 Moved Permanently "Moved Permanently"
/say-hello/Pam => 200 OK "Hello, Pam!"
*/
app.get('/say-hello/:name',
thinware(sayHello, req => req.params.name)
)
FAQs
HTTP status enabled Error class maintaining backward compatibility
The npm package errdrop receives a total of 22 weekly downloads. As such, errdrop popularity was classified as not popular.
We found that errdrop demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.