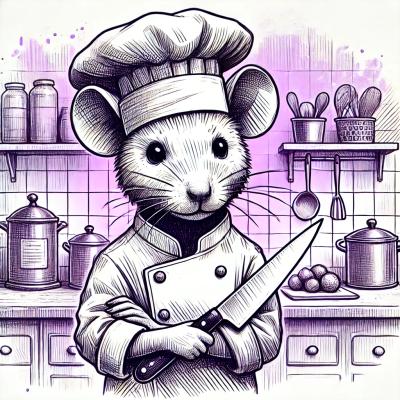
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
A thin, fast, low-level Promise-based wrapper around the Ethereum APIs.
Clone the repo and install dependencies via npm install
. Tests can be executed via
npm run testOnce
(100% covered unit tests)npm run testE2E
(E2E against a running RPC-enabled testnet Parity/Geth instance, parity --testnet
and for WebScokets, geth --testnet --ws --wsorigins '*' --rpc
)DEBUG=true
will display the RPC POST bodies and responses on E2E testsInstall the package with npm install --save ethapi-js
from the npm registry ethapi-js
// import the actual EthApi class
import EthApi from 'ethapi-js';
// do the setup
const transport = new EthApi.Transport.Http('http://localhost:8545'); // or .Ws('ws://localhost:8546')
const ethapi = new EthApi(transport);
You will require native Promises and fetch support (latest browsers only), they can be utilised by
import 'isomorphic-fetch';
import es6Promise from 'es6-promise';
es6Promise.polyfill();
perform a call
ethapi.eth
.coinbase()
.then((coinbase) => {
console.log(`The coinbase is ${coinbase}`);
});
multiple promises
Promise
.all([
ethapi.eth.coinbase(),
ethapi.net.listening()
])
.then(([coinbase, listening]) => {
// do stuff here
});
chaining promises
ethapi.eth
.newFilter({...})
.then((filterId) => ethapi.eth.getFilterChanges(filterId))
.then((changes) => {
console.log(changes);
});
attach contract
const abi = [{ name: 'callMe', inputs: [{ type: 'bool', ...}, { type: 'string', ...}]}, ...abi...];
const contract = new EthApi.Contract(ethapi, abi);
find & call a function
contract.functions
.find((func) => func.name === 'callMe')
.call({ gas: 21000 }, [true, 'someString']) // or estimateGas or sendTransaction
.then((result) => {
console.log(`the result was ${result}`);
});
parse events from transaction receipt
contract
.parseTransactionEvents(txReceipt)
.then((receipt) => {
receipt.logs.forEach((log) => {
console.log('log parameters', log.params);
});
});
For a list of the exposed APIs, along with their parameters, the following exist. These implement the calls as listed on the official JSON Ethereum RPC definition.
The interfaces maps through to the RPC db_<interface>
definition. These are deprecated and may not be available in all clients into the future
getHex(dbName, keyName)
- retrieves a stored data value from the databasegetString(dbName, keyName)
- retrieves a stored string from the databaseputHex(dbName, keyName, hexData)
- stores a data value in the local databaseputString(dbName, keyName, stringData)
- stores a string in the local databaseThe interfaces maps through to the RPC eth_<interface>
definition.
accounts()
- returns a list of accounts associated with the current running instanceblockNumber()
- returns the current blockNumbercall(options, blockNumber = 'latest')
- performs a callcoinbase()
- returns the current coinbase (base account) for the running instancecompile[LLL|Serpent|Solidity](code)
- compiles the supplied code using the required compilerestimateGas(options)
- performs a fake call using the options, returning the used gasfetchQueuedTransactions()
flush()
gasPrice()
- returns the current gas pricegetBalance(address, blockNumber = 'latest')
- returns the current address balance as at blockNumbergetBlockByHash(hash, full = false)
- gets the block by the specified hashgetBlockByNumber(blockNumber = 'latest', full = false)
- gets the block by blockNumbergetBlockTransactionCountByHash(hash)
- transaction count in specified hashgetBlockTransactionCountByNumber(blockNumber = 'latest')
- transaction count by blockNumbergetCode(address, blockNumber = 'latest')
- get the deployed code at addressgetCompilers()
- get a list of the available compilersgetFilterChanges(filterId)
- returns the changes to a specified filterIdgetFilterChangesEx(filterId)
getFilterLogs(filterId)
- returns the logs for a specified filterIdgetFilterLogsEx(filterId)
getLogs(options)
- returns logsgetLogsEx(options)
getStorageAt(address, index = 0, blockNumber = 'latest')
- retrieve the store at addressgetTransactionByBlockHashAndIndex(hash, index = 0)
getTransactionByBlockNumberAndIndex(blockNumber = 'latest', index = 0)
getTransactionByHash(hash)
getTransactionCount(address, blockNumber = 'latest')
getTransactionReceipt(txhash)
getUncleByBlockHashAndIndex(hash, index = 0)
getUncleByBlockNumberAndIndex(blockNumber = 'latest', index = 0)
getUncleCountByBlockHash(hash)
getUncleCountByBlockNumber(blockNumber = 'latest')
getWork()
hashrate()
inspectTransaction()
mining()
newBlockFilter()
newFilter(options)
newFilterEx(options)
notePassword()
newPendingTransactionFilter()
pendingTransactions()
protocolVersion()
register()
sendRawTransaction(data)
sendTransaction(options)
sign()
signTransaction()
submitHashrate(hashrate, clientId)
submitWork(nonce, powHash, mixDigest)
syncing()
uninstallFilter(filterId)
unregister()
TODO complete the descriptions where not available
The interfaces maps through to the RPC ethcore_<interface>
definition, extensions done by Ethcore on the Parity client.
extraData()
- returns currently set extra datagasFloorTarget()
- returns current target for gas floorminGasPrice()
- returns currently set minimal gas pricenetChain()
- returns the name of the connected chainnetMaxPeers()
- returns maximal number of peersnetPort()
- returns network port the node is listening onnodeName()
- returns node name (identity)setAuthor(address)
- changes author (coinbase) for mined blockssetExtraData(data)
- changes extra data for newly mined blockssetGasFloorTarget(quantity)
- changes current gas floor targetsetMinGasPrice(quantity)
- changes minimal gas price for transaction to be accepted to the queuesetTransactionsLimit(quantity)
- changes limit for transactions in queuetransactionsLimit()
- returns limit for transactions in queuerpcSettings()
- returns basic settings of rpc (enabled, port, interface)The interfaces maps through to the RPC net_<interface>
definition.
listening()
- returns the listening status of the networkpeerCount()
- returns the number of connected peersversion()
- returns the network versionThe interfaces maps through to the RPC personal_<interface>
definition.
listAccounts()
- list all the available accountsnewAccount(password)
- creates a new accountsignAndSendTransaction(txObject, password)
- sends and signs a transaction given account passphraseunlockAccount(account, password, duration = 5)
- unlocks the accountThe interfaces maps through to the RPC shh_<interface>
definition.
addToGroup(identity)
getFilterChanges(filterId)
getMessages(filterId)
hasIdentity(identity)
newFilter(options)
newGroup()
newIdentity()
post(options)
uninstallFilter(filterId)
version()
TODO complete the descriptions where not available
The interfaces maps through to the RPC trace_<interface>
definition.
filter(filterObj)
- returns traces matching given filterget(txHash, position)
- returns trace at given positiontransaction(txHash)
- returns all traces of given transactionblock(blockNumber = 'latest')
- returns traces created at given blockThe interfaces maps through to the RPC web3_<interface>
definition.
clientVersion()
- returns the version of the RPC clientsha3(hexStr)
- returns a keccak256 of the hexStr inputFAQs
A thin, fast, low-level Promise-based wrapper around the Ethereum APIs.
The npm package ethapi-js receives a total of 12 weekly downloads. As such, ethapi-js popularity was classified as not popular.
We found that ethapi-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.