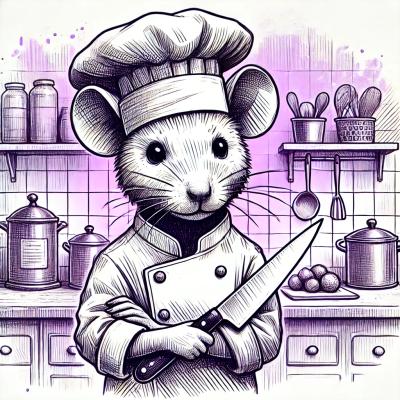
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
express-throttle
Advanced tools
Request throttling middleware for Express framework
$ npm install express-throttle
The throttling is done using the canonical token bucket algorithm, where tokens are "refilled" in a sliding window manner (as opposed to a fixed time interval). This means that if we set maximum rate of 10 requests / minute, a client will not be able to send 10 requests 0:59, and 10 more 1:01. However, if the client sends 10 requests at 0:30, he will be able to send a new request at 0:36 (as tokens are regenerated continuously 1 every 6 seconds).
var express = require("express");
var throttle = require("express-throttle");
var app = express();
// Throttle to 5 reqs/s
app.post("/search", throttle("5/s"), function(req, res, next) {
// ...
});
Combine it with a burst capacity of 10, meaning that the client can make 10 requests at any rate. The capacity is "refilled" with the specified rate (in this case 5/s).
app.post("/search", throttle({ "rate": "5/s", "burst": 10 }), function(req, res, next) {
// ...
});
// Decimal values for rate values are allowed
app.post("/search", throttle({ "rate": "0.5/s", "burst": 5 }), function(req, res, next) {
// ...
});
By default, throttling is done on a per ip-address basis (respecting the X-Forwarded-For header). This can be configured by providing a custom key-function:
var options = {
"rate": "5/s",
"burst": 10,
"key": function(req) {
return req.session.username;
}
};
app.post("/search", throttle(options), function(req, res, next) {
// ...
});
The "cost" per request can also be customized, making it possible to, for example whitelist certain requests:
var whitelist = ["ip-1", "ip-2", ...];
var options = {
"rate": "5/s",
"burst": 10,
"cost": function(req) {
var ip_address = req.connection.remoteAddress;
if (whitelist.indexOf(ip_address) >= 0) {
return 0;
} else if (req.session.is_privileged_user) {
return 0.5;
} else {
return 1;
}
}
};
app.post("/search", throttle(options), function(req, res, next) {
// ...
});
var options = {
"rate": "1/s",
"burst": 10,
"cost": 2.5 // fixed costs are also supported
};
app.post("/expensive", throttle(options), function(req, res, next) {
// ...
});
Throttled requests will simply be responded with an empty 429 response. This can be overridden:
var options = {
"rate": "5/s",
"burst": 10,
"on_throttled": function(req, res) {
// Possible course of actions:
// 1) Add client ip address to a ban list
// 2) Send back more information
res.set("X-Rate-Limit-Limit", 5);
res.status(503).send("System overloaded, try again at a later time.");
}
};
Throttling can be applied across multiple processes. This requires an external storage mechanism which can be configured as follows:
function ExternalStorage(connection_settings) {
// ...
}
// These methods must be implemented
ExternalStorage.prototype.get = function(key, callback) {
fetch(key, function(entry) {
// First argument should be null if no errors occurred
callback(null, entry);
});
}
ExternalStorage.prototype.set = function(key, value, callback) {
save(key, value, function(err) {
// err should be null if no errors occurred
callback(err);
});
}
var options = {
"rate": "5/s",
"store": new ExternalStorage()
}
app.post("/search", throttle(options), function(req, res, next) {
// ...
});
rate
: Determines the number of requests allowed within the specified time unit before subsequent requests get throttled. Must be specified according to the following format: decimal/time-unit
decimal: A non-negative decimal value. This value is internally stored as a float, but scientific notation is not supported (e.g 10e2).
time-unit: Any of the following: s, sec, second, m, min, minute, h, hour, d, day
burst
: The number of requests that can be made at any rate. The burst quota is refilled with the specified rate
.
store
: Custom storage class. Must implement a get
and set
method with the following signatures:
// callback in both methods must be called with an error (if any) as first argument
function get(key, callback) {
fetch(key, function(err, value) {
if (err) callback(err);
else callback(null, value);
});
}
function set(key, value, callback) {
// value will be an object with the following structure:
// { "tokens": Number, "accessed": Number }
save(key, value, function(err) {
callback(err);
}
}
Defaults to an LRU cache with a maximum of 10000 entries.
key
: Function used to identify clients. It will be called with an express request object. Defaults to:
function(req) {
return req.headers["x-forwarded-for"] || req.connection.remoteAddress;
}
cost
: Number or function used to calculate the cost for a request. It will be called with an express request object. Defaults to 1.
on_throttled
: A function called when the request is throttled. It will be called with an express request object and express response object. Defaults to:
function(req, res) {
res.status(429).end();
};
FAQs
Request throttling middleware for Express
The npm package express-throttle receives a total of 3,210 weekly downloads. As such, express-throttle popularity was classified as popular.
We found that express-throttle demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.