Comparing version 0.2.2 to 0.3.0
const fs = require('fs'); | ||
const request = require('request'); | ||
const createFolder = require('./createFolder.js'); | ||
const createFolder = require('./functions/createFolder.js'); | ||
@@ -23,3 +23,3 @@ function downloadImages() { | ||
const imageName = Object.keys(_data)[index]; | ||
download(image, `specs/images/${imageName}.jpg`, function () { | ||
download(image, `specs/images/${imageName}.jpg`, function() { | ||
console.log(`Downloaded image: ${imageName}`); | ||
@@ -32,4 +32,4 @@ }); | ||
const download = function (uri, filename, callback) { | ||
request.head(uri, function (err, res, body) { | ||
const download = function(uri, filename, callback) { | ||
request.head(uri, function(err, res, body) { | ||
request(uri) | ||
@@ -36,0 +36,0 @@ .pipe(fs.createWriteStream(filename)) |
@@ -7,6 +7,10 @@ const fs = require('fs'); | ||
const keys = require('./meta/keys.js'); | ||
const figmaUrl = keys.url; | ||
const figmaToken = keys.token; | ||
const options = { | ||
url: `https://api.figma.com/v1/images/{FILE}?ids=${resolvedImageIds}&format=png&scale=2`, | ||
url: `https://api.figma.com/v1/images/${figmaUrl}?ids=${resolvedImageIds}&format=png&scale=2`, | ||
headers: { | ||
'X-Figma-Token': '{TOKEN}' | ||
'X-Figma-Token': figmaToken | ||
} | ||
@@ -13,0 +17,0 @@ }; |
#! /usr/bin/env node | ||
const createPage = require('./createPage.js'); | ||
const writeTokens = require('./writeTokens.js'); | ||
const writeComponents = require('./writeComponents.js'); | ||
const setupComponents = require('./setupComponents.js'); | ||
// Function requires | ||
const createIds = require('./functions/createIds.js'); | ||
const createPage = require('./functions/createPage.js'); | ||
const writeTokens = require('./functions/writeTokens.js'); | ||
const writeComponents = require('./functions/writeComponents.js'); | ||
const setupComponents = require('./functions/setupComponents.js'); | ||
// Refs | ||
const figmaDocument = require(`${process.cwd()}/figma/figma.json`); | ||
@@ -12,28 +15,21 @@ const figmaPages = figmaDocument.document.children; | ||
let designtokensPage = createPage(figmaPages, 'designtokens'); | ||
let componentsPage = createPage(figmaPages, 'components'); | ||
// Page setup | ||
let pageNames = ['grid', 'designtokens', 'components']; | ||
let pages = {}; | ||
/* TODO | ||
** Add null checking | ||
*/ | ||
initIds(); | ||
createTokens(); | ||
createComponents(); | ||
pageNames.forEach(page => { | ||
pages[page] = createPage(figmaPages, page); | ||
}); | ||
function initIds() { | ||
const createIds = require('./createIds.js'); | ||
createIds(figmaComponents); | ||
} | ||
// Create IDs for components | ||
createIds(figmaComponents); | ||
function createTokens() { | ||
const designTokens = designtokensPage.children; | ||
writeTokens(designTokens); | ||
} | ||
// Create grid, tokens, components | ||
writeTokens(pages.grid[0], true); | ||
writeTokens(pages.designtokens.children); | ||
writeComponents(setupComponents(figmaComponents, pages.components)); | ||
function createComponents() { | ||
const fixedFigmaComponents = setupComponents(figmaComponents, componentsPage); | ||
writeComponents(fixedFigmaComponents); | ||
} | ||
/* TODO | ||
** Add null checking | ||
/* | ||
function displayWarning() { | ||
@@ -40,0 +36,0 @@ console.warn( |
{ | ||
"name": "figmagic", | ||
"version": "0.2.2", | ||
"version": "0.3.0", | ||
"author": "Mikael Vesavuori", | ||
@@ -11,8 +11,10 @@ "main": "index.js", | ||
"config": { | ||
"figmagicPath": "node_modules/figmagic" | ||
"figmagicPath": "./node_modules/figmatic/" | ||
}, | ||
"scripts": { | ||
"figmagic": "yarn figmagic:clean && yarn run figmagic:saveFromApi && yarn figmagic:build && yarn figmagic:getImages && yarn figmagic:downloadImages", | ||
"figmagic": | ||
"yarn figmagic:clean && yarn run figmagic:saveFromApi && yarn figmagic:build && yarn figmagic:getImages && yarn figmagic:downloadImages", | ||
"figmagic:clean": "rm -rf specs/ && rm -rf tokens/ && rm -rf figma", | ||
"figmagic:saveFromApi": "mkdir -p figma && wget 'https://api.figma.com/v1/files/{FILE}' --header='X-Figma-Token: {TOKEN}' -O figma/figma.json", | ||
"figmagic:saveFromApi": | ||
"mkdir -p figma && wget 'https://api.figma.com/v1/files/{FILE}' --header='X-Figma-Token: {TOKEN}' -O figma/figma.json", | ||
"figmagic:tokens": "yarn figmagic:clean && yarn figmagic:saveFromApi && yarn figmagic:build", | ||
@@ -19,0 +21,0 @@ "figmagic:build": "node $npm_package_config_figmagicPath/bin/index.js", |
@@ -8,2 +8,4 @@ # Figmagic | ||
Note: Requires that your document structure is identical to what I show in the template site at [https://www.figma.com/file/KLLDK9CBSg7eAayiTY3kVqC8/Figmagic-Design-System-Example](https://www.figma.com/file/KLLDK9CBSg7eAayiTY3kVqC8/Figmagic-Design-System-Example). | ||
## Approach and use cases | ||
@@ -44,2 +46,7 @@ | ||
## Key/token locations | ||
1. `package.json`: In the scripts block you will need to change the blanks to your actual file and token | ||
2. `meta/keys.js`: In the options object you will also need to change to your actual values; these are then used by Figmagic's functions | ||
## Commands | ||
@@ -66,4 +73,14 @@ | ||
Your structure needs to correspond to the following: | ||
* Pages need to exist and be called "Components", "Design tokens", and "Grid" | ||
* Further, inside "Design tokens", frames need to be called "Colors", "Font sizes", "Font families", "Font weights", "Line heights", and "Spacing" – exact casing is not important, however the **spelling is important!** | ||
* All items on a page need to be contained within one or more frames | ||
See a demo/template at [https://www.figma.com/file/KLLDK9CBSg7eAayiTY3kVqC8/Figmagic-Design-System-Example](https://www.figma.com/file/KLLDK9CBSg7eAayiTY3kVqC8/Figmagic-Design-System-Example). | ||
Note: You must follow the document structure as seen in the image below and in the template linked above. | ||
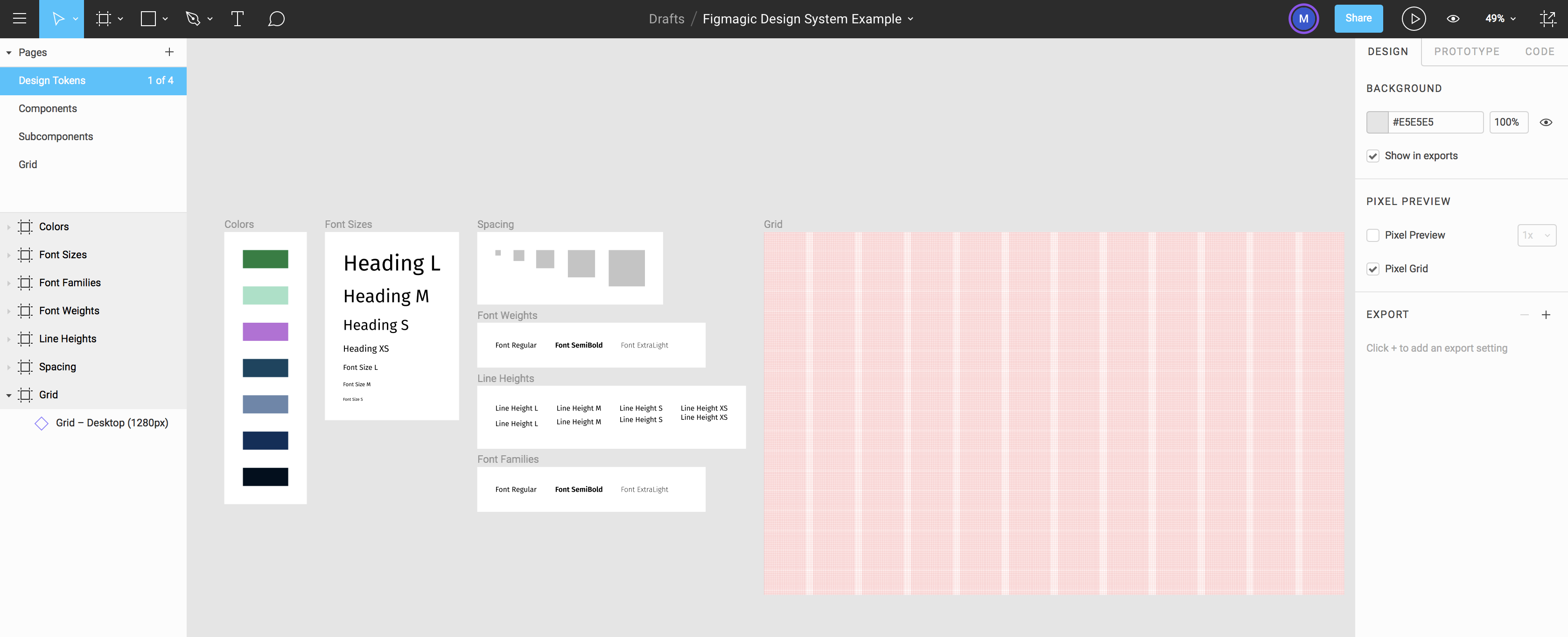 | ||
## Token formatting/conversion | ||
@@ -73,3 +90,3 @@ | ||
Uses any combination of rows, columns, and grid (but only one of each type). | ||
Uses any combination of rows, columns, and grid (but only one of each type). Grid sizes will use the native Javascript `Math.floor()` function to round down any decimal values. This may or may not cause issues, but is at least more hygienic than leaving potentially weird numbers for grid sizes. | ||
@@ -82,3 +99,3 @@ ### Font families | ||
Work in progress. | ||
**Work in progress**. | ||
@@ -103,7 +120,31 @@ ### Font sizes | ||
Text to come. | ||
Specifications for components are generated into the `specs` folder. A component will be anything that Figma catches as a component, regardless if they exist in your "Components" page or not. | ||
### Example of a Button component | ||
```js | ||
module.exports = { | ||
name: 'Button', | ||
gridWidth: 2, | ||
perfectlyFitsGrid: true, | ||
pxWidth: 200, | ||
pxHeight: 40, | ||
description: 'Regular button\nBold text\nLine height medium', | ||
subComponents: ['Box', 'Button Text'], | ||
id: '5:9' | ||
}; | ||
``` | ||
* **name**: The name of the component inside Figma | ||
* **gridWidth**: How wide the component is counted in your grid units. _Note:_ It will always use a higher value if not an exact fit (see below) | ||
* **perfectlyFitsGrid**: A boolean (true/false) to debug whether the component fits exactly in the grid or if it's bigger (see above) | ||
* **pxWidth**: How many pixels wide is the component in Figma | ||
* **pxHeight**: How many pixels high is the component in Figma | ||
* **description**: The description given in Figma. _Hint:_ Use this! | ||
* **subComponents**: What first-level subcomponents does the component include? | ||
* **id**: The Figma internal ID for the component | ||
## Structure | ||
* `bin` contains the project's JS files | ||
* `bin` contains the project's JS files; `bin/functions` contains most of the functions | ||
* `figma` will contain the extracted Figma JSON and various build-time JSON files | ||
@@ -118,5 +159,4 @@ * `tokens` will contain the token files (in .js format) | ||
* Still getting too many specs (for subcomponents)...? | ||
* Extract design specs for subcomponents as well | ||
* Map component values to tokens | ||
* Create error report if something gets botched during build-time | ||
* If Figma gets webhooks, create some way of automatically pulling updates |
Major refactor
Supply chain riskPackage has recently undergone a major refactor. It may be unstable or indicate significant internal changes. Use caution when updating to versions that include significant changes.
Found 1 instance in 1 package
461392
52
707
156
9
1