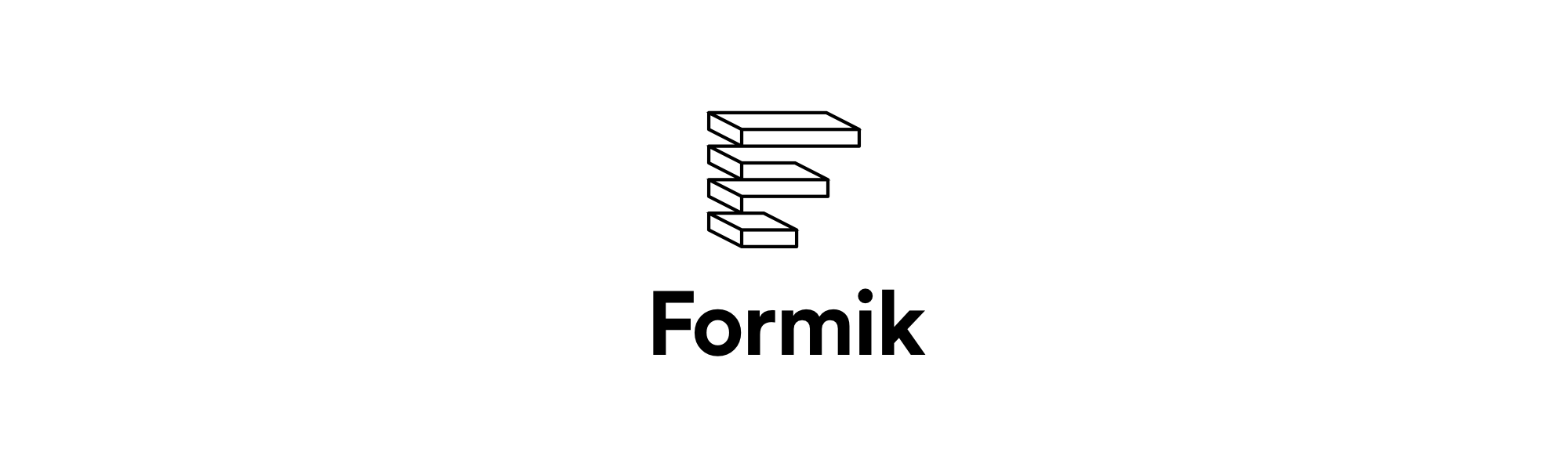
Forms in React, without tears.
Let's face it, forms are really really verbose in React. To make matters worse, most form helpers do wayyyyy too much magic and often have a significant performace cost. Formik is minimal a Higher Order Component that helps you with the 3 most annoying parts:
- Transforming props to a flat React state,
- Validation and error messages
- Transforming a flat React state back into a consumable payload for your API
Lastly, Formik helps you stay organized by colocating all of the above plus your submission handler in one place. This makes testing, refactoring, and reasoning about your forms a breeze.
Installation
Add Formik and Yup to your project. Formik uses Yup (Joi for the browser) for schema validation.
npm i formik yup --save
Table of Contents
Usage
Simple Example
Imagine you want to build a form that lets you edit user data. However, your user API has nested objects like so.
{
id: string,
email: string,
social: {
facebook: string,
twitter: string,
....
}
}
When we are done we want our form to accept just a user
prop and that's it.
import React from 'react';
import Dialog from 'MySuperDialog';
import EditUserForm from './EditUserForm';
const EditUserDialog = ({ user }) =>
<Dialog>
<EditUserForm user={user} />
</Dialog>;
Enter Formik.
import React from 'react';
import Formik from 'formik';
import Yup from 'yup';
const SimpleForm = ({ values, handleChange, handleSubmit, handleReset, errors, error, isSubmitting, }) =>
<form onSubmit={handleSubmit}>
<input
type="text"
name="email"
value={values.email}
onChange={handleChange}
placeholder="john@apple.com"
/>
{errors.email && <div>{errors.email}</div>}
<input
type="text"
name="facebook"
value={values.facebook}
onChange={handleChange}
placeholder="facebook username"
/>
{errors.facebook && <div>{errors.facebook}</div>}
<input
type="text"
name="twitter"
value={values.twitter}
onChange={handleChange}
placeholder="twitter username"
/>
{errors.twitter && <div>{errors.twitter}</div>}
{error && error.message && <div style={{color: 'red'}}>Top Level Error: {error.message}</div>}
<button onClick={handleReset}>Reset</button>
<button type="submit" disabled={isSubmitting}>Submit</button>
</form>;
export default Formik({
displayName: 'SimpleForm',
validationSchema: Yup.object().shape({
email: Yup.string().email().required(),
twitter: Yup.string(),
facebook: Yup.string(),
}),
mapPropsToValues: props => ({
email: props.user.email,
twitter: props.user.social,
facebook: props.user.facebook,
}),
mapValuesToPayload: values => ({
email: values.email,
social: {
twitter: values.twitter,
facebook: values.facebook
},
}),
handleSubmit: (payload, { props, setError, setSubmitting }) => {
CallMyApi(props.user.id, payload)
.then(
res => {
setSubmitting(false)
},
err => {
setSubmitting(false)
setError(err)
}
)
},
})(SimpleForm);
API
Formik(options)
Create A higher-order React component class that passes props and form handlers (the "FormikBag
") into your component derived from supplied options.
options
displayName: string
Set the display name of your component.
handleSubmit: (payload, FormikBag) => void
)
Your form submission handler. It is passed the result of mapValuesToPayload
(if specified), or the result of mapPropsToValues
, or all props that are not functions (in that order of precedence) and the "FormikBag
".
mapPropsToValues?: (props) => props
If this option is specified, then Formik will transfer its results into updatable form state and make these values available to the new component as props.values
. If mapPropsToValues
is not specified, then Formik will map all props that are not functions to the new component's props.values
. That is, if you omit it, Formik will only pass props
where typeof props[k] !== 'function'
, where k
is some key.
mapValuesToPayload?: (values) => payload
If this option is specified, then Formik will run this function just before calling handleSubmit
. Use it to transform the your form's values
back into a shape that's consumable for other parts of your application or API. If mapValuesToPayload
is not specified, then Formik will map all values
directly to payload
(which will be passed to handleSubmit
). While this transformation can be moved into handleSubmit
, consistently defining it in mapValuesToPayload
separates concerns and helps you stay organized.
validationSchema: Schema
A Yup schema. This is used for validation on each onChange event. Errors are mapped by key to the WrappedComponent
's props.errors
. Its keys should almost always match those of WrappedComponent's
props.values
.
Injected props and methods
The following props and methods will be injected into the WrappedComponent
(i.e. your form):
error?: any
A top-level error object, can be whatever you need.
errors: { [field]: string }
Form validation errors. Keys match the shape of the validationSchema
defined in Formik options. This should therefore also map to your values
object as well. Internally, Formik transforms raw Yup validation errors on your behalf.
handleBlur: (e: any) => void
onBlur
event handler. Useful for when you need to track whether an input has been touched
or not. This should be passed to <input onBlur={handleBlur} ... />
handleChange: (e: React.ChangeEvent<any>) => void
General input change event handler. This will update the form value according to an input's name
attribute. If name
is not present, handleChange
will look for an input's id
attribute. Note: "input" here means all HTML inputs.
handleChangeValue: (name: string, value: any) => void
Custom input change handler. Use this when you have custom inputs. name
should match the key of form value you wish to update.
handleReset: () => void
Reset handler. This should be passed to <button onClick={handleReset}>...</button>
handleSubmit: (e: React.FormEvent<HTMLFormEvent>) => void
Submit handler. This should be passed to <form onSubmit={handleSubmit}>...</form>
isSubmitting: boolean
Submitting state. Either true
or false
. Formik will set this to true
on your behalf before calling handleSubmit
to reduce boilerplate.
resetForm: (nextProps?: Props) => void
Imperatively reset the form. This will clear errors
and touched
, set isSubmitting
to false
and rerun mapPropsToValues
with the current WrappedComponent
's props
or what's passed as an argument. That latter is useful for calling resetForm
within componentWillReceiveProps
.
setError(err: any) => void
Set a top-level error
object. This can only be done manually. It is an escape hatch.
setErrors(fields: { [field]: string }) => void
Set errors
manually.
setSubmitting(boolean) => void
Set a isSubmitting
manually.
setTouched(fields: { [field]: string }) => void
Set touched
manually.
setValues(fields: { [field]: any }) => void
Set values
manually.
touched: { [field]: string }
Touched fields. Use this to keep track of which fields have been visited. Use handleBlur
to toggle on a given input. Keys work like errors
and values
.
values: { [field]: any }
Your form's values, the result of mapPropsToValues
(if specified) or all props that are not functions passed to your WrappedComponent
.
Recipes
Ways to call Formik
Formik is a Higher Order Component factory or "Monad" in functional programming lingo. In practice, you use it exactly like React Redux's connect
or Apollo's graphql
. There are basically three ways to call Formik on your component:
You can assign the HoC returned by Formik to a variable (i.e. withFormik
) for later use.
import React from 'react';
import Formik from 'formik';
const withFormik = Formik({...});
const MyForm = (props) => (
<form onSubmit={props.handleSubmit}>
<input type="text" name="thing" value={props.values.thing} onChange={props.handleChange} />
<input type="submit" value="Submit"/>
</form>
);
export default withFormik(MyForm);
You can also skip a step and immediately invoke (i.e. "curry") Formik instead of assigning it to a variable. This method has been popularized by React Redux. One downside is that when you read the file containing your form, its props seem to come out of nowhere.
import React from 'react';
import Formik from 'formik';
const MyForm = (props) => (
<form onSubmit={props.handleSubmit}>
<input type="text" name="thing" value={props.values.thing} onChange={props.handleChange} />
<input type="submit" value="Submit"/>
</form>
);
export default Formik({...})(MyForm);
Lastly, you can define your form component anonymously:
import React from 'react';
import Formik from 'formik';
export default Formik({...})((props) => (
<form onSubmit={props.handleSubmit}>
<input type="text" name="thing" value={props.values.thing} onChange={props.handleChange} />
<input type="submit" value="Submit"/>
</form>
));
I suggest using the first method, as it makes it clear what Formik is doing. It also let's you configure Formik above your component, so when you read your form's code, you know where those props are coming from. It also makes testing much easier.
Accessing React Component Lifecycle Functions
Sometimes you need to access React Component Lifecycle methods to manipulate your form. In this situation, you have some options:
- Lift that lifecycle method up into a React class component that's child is your Formik-wrapped form and pass whatever props it needs from there
- Convert your form into a React component class (instead of a stateless functional component) and wrap that class with Formik.
There isn't a hard rule whether one is better than the other. The decision comes down to whether you want to colocate this logic with your form or not. (Note: if you need refs
you'll need to convert your stateless functional form component into a React class anyway).
Example: Resetting a form when props change
import React from 'react';
import Formik from 'formik'
const withFormik = Formik({...});
class MyForm extends React.Component {
componentWillReceiveProps(nextProps) {
if (nextProps.thing !== this.props.thing) {
this.props.resetForm(nextProps)
}
}
render() {
return (
<form onSubmit={this.props.handleSubmit}>
<input
type="text"
name="thing"
value={this.props.values.thing}
onChange={this.props.handleChange}
/>
<input type="submit" value="Submit" />
</form>
);
}
}
export default withFormik(MyForm);
As for colocating a React lifecycle method with your form, imagine a situation where you want to use if you have a modal that's only job is to display a form based on the presence of props or not.
Authors
MIT License.