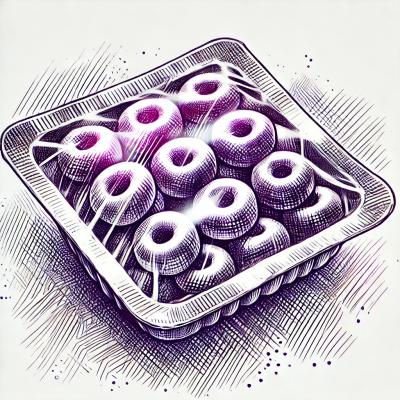
Security News
Understanding the Security Concerns of npm Shrinkwrap
Explore the security risks of using npm shrinkwrap, the potential for outdated dependencies, and best practices for mitigating these concerns in your projects.
fs-jetpack
Advanced tools
Package description
fs-jetpack is a file system utility library for Node.js that simplifies common file system operations. It provides a more intuitive and chainable API compared to the built-in 'fs' module, making it easier to perform tasks like reading, writing, copying, and deleting files and directories.
Reading Files
This feature allows you to read the contents of a file. The 'read' method takes the file path and encoding as arguments and returns the file content.
const jetpack = require('fs-jetpack');
const data = jetpack.read('path/to/file.txt', 'utf8');
console.log(data);
Writing Files
This feature allows you to write data to a file. The 'write' method takes the file path and the data to be written as arguments.
const jetpack = require('fs-jetpack');
jetpack.write('path/to/file.txt', 'Hello, world!');
Copying Files and Directories
This feature allows you to copy files or directories from one location to another. The 'copy' method takes the source path and the destination path as arguments.
const jetpack = require('fs-jetpack');
jetpack.copy('path/to/source', 'path/to/destination');
Deleting Files and Directories
This feature allows you to delete files or directories. The 'remove' method takes the path of the file or directory to be deleted as an argument.
const jetpack = require('fs-jetpack');
jetpack.remove('path/to/file_or_directory');
Inspecting Files and Directories
This feature allows you to get information about a file or directory. The 'inspect' method takes the path as an argument and returns an object with details like size, type, and modification time.
const jetpack = require('fs-jetpack');
const info = jetpack.inspect('path/to/file_or_directory');
console.log(info);
fs-extra is a popular file system utility library that extends the built-in 'fs' module with additional methods for common tasks like copying, moving, and removing files and directories. It is similar to fs-jetpack but offers a more extensive set of features and is widely used in the Node.js community.
node-fs is another file system utility library that provides additional methods for working with the file system. It offers functionalities like recursive directory creation and symbolic link management. While it is less feature-rich compared to fs-jetpack, it is still a useful alternative for basic file system operations.
graceful-fs is a drop-in replacement for the built-in 'fs' module that improves the handling of file system operations, especially under heavy load. It is designed to be more resilient and less prone to errors, making it a good choice for applications that require robust file system interactions.
Readme
#fs-jetpack
Attempt to make comprehensive, higher level API for node's fs library.
###Installation
npm install fs-jetpack
###Usage
var jetpack = requite('fs-jetpack');
#API
All asynchronous methods are promise based, and are using Q library for that purpose.
Commonly used naming convention in node world is reversed in this library. Asynchronous methods are those with "Async" suffix, all methods without "Async" in name are synchronous. Reason behind this is that it gives very nice look to blocking API, and promise based non-blocking code is verbose anyway, so one more word is not much of a difference. Also with this approach all methods without word "Async" are synchronous so you can very easily distinguish one from another.
Index
###cwd([path]) Returns Current Working Directory (CWD) path, or creates new jetpack object with different CWD.
parameters:
path
(optional) path to become new CWD. Could be absolute, or relative. If relative path given new CWD will be resolved basing on current CWD.
returns:
If path
not specified, returns CWD path of this jetpack object. For main instance of fs-jetpack it is always process.cwd()
.
If path
specified, returns new jetpack object (totally the same thing as main jetpack). The new object resolves paths according to its inner CWD, not the global one (process.cwd()
).
examples:
// let's assume that process.cwd() outputs...
console.log(process.cwd()); // '/one/two/three'
// jetpack.cwd() will always return the same value as process.cwd()
console.log(jetpack.cwd()); // '/one/two/three'
// now let's create new CWD context...
var jetParent = jetpack.cwd('..');
console.log(jetParent.cwd()); // '/one/two'
// ...and use this new context
jetParent.dir('four'); // we just created directory '/one/two/four'
// one CWD context can be used to create next CWD context
var jetParentParent = jetpackContext.cwd('..');
console.log(jetParentParent.cwd()); // '/one'
###copy(from, to, [options]) Copies given file or directory.
parameters:
from
path to location you want to copy.
to
destination path where copy should be placed.
options
(optional) additional options for customization. Is an object
with possible fields:
overwrite
(default: 'no'
) mode to use if file already exists in destination location. Is a string
with possible values:
'no'
don't allow to replace any file or directory in destination location.'yes'
replace every file already existing.only
(array
of masks) will copy only items matching any of specified masks. Mask is string
with .gitignore-like notation (see section "Matching paths .gitignore style").allBut
(array
of masks) will copy everything except items matching any of specified masks. If only
is specified this field is ignored.symlinks
(TODO, not implemented yet)returns:
Recently used CWD context.
examples:
// copy file and replace it if exists
jetpack.copy('/my_file.txt', '/somwhere/my_file.txt', { overwrite: 'yes' });
// copy only .txt files inside my_dir
jetpack.copy('/my_dir', '/somewhere/my_dir', { only: ['*.txt'] });
// copy everything except temp directory inside my_dir
jetpack.copy('/my_dir', '/somewhere/my_dir', { allBut: ['my_dir/temp'] });
###copyAsync(from, to, [options])
Asynchronous equivalent of copy()
method. The only difference is that it returns promise.
###dir(path, [criteria]) Ensures that directory meets given criteria. If any criterium is not met it will be after this call.
parameters:
path
path to directory to examine.
criteria
(optional) criteria to be met by the directory. Is an object
with possible fields:
exists
(default: true
) whether directory should exist or not. If set to true
and path
contains many nested, nonexistent directories all of them will be created.empty
(default: false
) whether directory should be empty (no other files or directories inside). If set to true
and directory contains any files or subdirectories all of them will be deleted. If exists = false
this field is ignored.mode
ensures directory has specified mode. If not set and directory already exists, current mode will be intact. Value could be number (eg. 0700
) or string (eg. '700'
).returns:
New CWD context with directory specified in path
as CWD.
If exists
field was set to false
returned CWD context points to parent directory of given path
.
examples:
// creates directory if not exists
jetpack.dir('new_dir');
// make sure that directory does NOT exist
var notExistsCwd = jetpack.dir('/my_stuff/some_dir', { exists: false });
// if exists == false, returned CWD context refers to parent of specified directory
console.log(notExistsCwd.cwd()) // '/my_stuff'
// creates directory with mode 0700 (if not exists)
// or make sure that it's empty and has mode 0700 (if exists)
jetpack.dir('empty_dir', { empty: true, mode: '700' });
// because dir returns new CWD context pointing to just
// created directory you can create dir chains
jetpack
.dir('main_dir') // creates 'main_dir'
.dir('sub_dir'); // creates 'main_dir/sub_dir'
###dirAsync(path, [criteria])
Asynchronous equivalent of dir()
method. The only difference is that it returns promise.
###exists(path)
Checks whether something exists on given path
. This method returns values more specyfic than true/false
to protect from errors like "I was expecting directory, but it was a file".
returns:
false
if path doesn't exist."dir"
if path is a directory."file"
if path is a file."other"
if path exists, but is of different "type".###existsAsync(path)
Asynchronous equivalent of exists()
method. The only difference is that it returns promise.
###file(path, [criteria]) Ensures that file meets given criteria. If any criterium is not met it will be after this call.
parameters:
path
path to file to examine.
criteria
(optional) criteria to be met by the directory. Is an object
with possible fields:
exists
(default: true
) whether file should exist or not.empty
(default: false
) whether file should be forced to be empty. If exists = false
this field is ignored.content
(string
, buffer
, object
ot array
) sets file content. If object
or array
given to this parameter the output will be JSON. If exists = false
, or empty = true
this field is ignored.mode
ensures file has specified mode. If not set and file already exists, current mode will be intact. Value could be number (eg. 0700
) or string (eg. '700'
).returns:
Recently used CWD context.
examples:
// creates file if not exists
jetpack.file('something.txt');
// ensure file does NOT exist (if exists will be deleted)
jetpack.file('not_something.txt', { exists: false });
// creates file with mode '777' and content 'Hello World!'
jetpack.file('hello.txt', { mode: '777', content: 'Hello World!' });
###fileAsync(path, [criteria])
Asynchronous equivalent of file()
method. The only difference is that it returns promise.
###list(path, [options]) Creates list of files inside given path (and more).
parameters:
path
path to file/directory to list.
options
(optional) additional options for customization. Is an object
with possible fields:
includeRoot
(default: false
) whether returned data should contain root directory (dir provided in path
), or only its children.subDirs
(default: false
) whether subdirectories should be also listed.symlinks
(TODO, not implemented yet)returns:
array
of objects
with most basic settings, and tree structure with more sophisticated settings.
examples:
jetpack.list('rootDir'); // will return array of objects
jetpack.list('rootDir', { includeRoot: true, subDirs: true });
// will return tree structure of this shape:
{
name: 'rootDir', // there is one root object if includeRoot was set to true
type: 'dir',
path: '/myStuff/rootDir', // absolute path to this location
size: 150, // (in bytes) in case of directory this number is combined size of all children
parent: null, // this directory actually has parent, but it was not scanned, so is not reachable
children: [ // contains Array of all dirs and files inside this directory
{
name: 'myFile.txt',
type: 'file',
path: '/myStuff/rootDir/myFile.txt',
parent: [Object], // reference to parent object
size: 150
}
]
}
###listAsync(path, [options])
Asynchronous equivalent of list()
method. The only difference is that it returns promise.
###path([parts...]) Returns path resolved to current CWD.
parameters:
parts
(optional) strings to join and resolve as path (as many as you like).
returns:
Resolved path as String.
examples:
jetpack.cwd(); // if it returns '/one/two'
jetpack.path(); // this will return the same '/one/two'
jetpack.path('three'); // this will return '/one/two/three'
jetpack.path('..', 'four'); // this will return '/one/four'
###read(path, [mode]) Reads content of file.
parameters:
path
path to file.
mode
(optional) how the content of file should be returned. Is a String with possible values:
'utf8'
(default) content will be returned as UTF-8 String.'buf'
content will be returned as Buffer.'json'
content will be returned as parsed JSON object.returns:
File content in specified format.
###readAsync(path, [mode])
Asynchronous equivalent of read()
method. The only difference is that it returns promise.
###remove(path, [options]) Deletes given path, no matter what it is (file or directory).
parameters:
path
path to file/directory to remove.
options
(optional) additional conditions to removal process. Is an object
with possible fields:
only
(array
of masks) will delete only items matching any of specified masks. Mask is string
with .gitignore-like notation (see section "Matching paths .gitignore style").allBut
(array
of masks) will delete everything except items matching any of specified masks. Mask is string
with .gitignore-like notation (see section "Matching paths .gitignore style"). If only
is specified this field is ignored.returns:
CWD context of directory parent to removed path.
examples:
// will delete 'notes.txt'
jetpack.remove('my_work/notes.txt');
// will delete directory 'important_stuff' and everything inside
jetpack.remove('my_work/important_stuff');
// will delete any .log file, and any folder or file named 'temp' inside 'my_app',
// but will leave all other files intact
jetpack.remove('my_app', { only: [ '*.log', 'temp' ] });
// will delete everything inside 'my_app' directory,
// but will leave directory 'my_app/user_data' intact
jetpack.remove('my_app', { allBut: [ 'my_app/user_data' ] });
###removeAsync(path, [options])
Asynchronous equivalent of remove()
method. The only difference is that it returns promise.
###write(path, content) Writes content to file.
parameters:
path
path to file.
content
data to be written. This could be string
, buffer
, object
or array
(if last two used, the data will be outputed into file as JSON).
returns:
Recently used CWD context.
###writeAsync(path, content)
Asynchronous equivalent of write()
method. The only difference is that it returns promise.
#Matching paths .gitignore style
For filtering options (only
and allBut
properties) this library uses notation familiar to you from .gitignore file (thanks to minimatch).
Few examples:
'work' // matches any item (file or dir) named "work", nevermind in which subdirectory it is
'*.txt' // matches any .txt file, nevermind in which subdirectory it is
'my_documents/*' // matches any file inside directory "my_documents"
'logs/2013-*.log' // matches any log file from 2013
'logs/2013-12-??.log' // matches any log file from december 2013
'my_documents/**/work' // matches any item named "work" inside "my_documents" and its subdirs
#Chaining jetpack commands
Because almost every jetpack method returns CWD context, you can chain commands together. What lets you operate on files in more declarative style, for example:
/*
We want to create file structure:
my_stuff
|- work
| |- hello.txt
|- photos
| |- me.jpg
*/
// Synchronous way
jetpack
.dir('my_stuff')
.dir('work')
.file('hello.txt', { content: 'Hello world!' })
.cwd('..') // go back to parent directory
.dir('photos')
.file('me.jpg', { content: new Buffer('should be image bytes') });
// Asynchronous way (unfortunately not that pretty)
jetpack
.dirAsync('my_stuff')
.then(function (context) {
return context.dirAsync('work');
})
.then(function (context) {
return context.fileAsync('hello.txt', { content: 'Hello world!' });
})
.then(function (context) {
return context.cwd('..').dirAsync('photos');
})
.then(function (context) {
return context.fileAsync('me.jpg', {
content: new Buffer('should be image bytes')
});
});
FAQs
Better file system API
The npm package fs-jetpack receives a total of 465,839 weekly downloads. As such, fs-jetpack popularity was classified as popular.
We found that fs-jetpack demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Explore the security risks of using npm shrinkwrap, the potential for outdated dependencies, and best practices for mitigating these concerns in your projects.
Security News
Node.js is taking steps towards removing Corepack from its distribution, aiming for changes in the next major release.
Security News
OpenSSF has released a guide to help package repositories adopt Trusted Publishers, which enhances security by using short-lived identity tokens for authentication, reducing the risks associated with long-lived secrets.