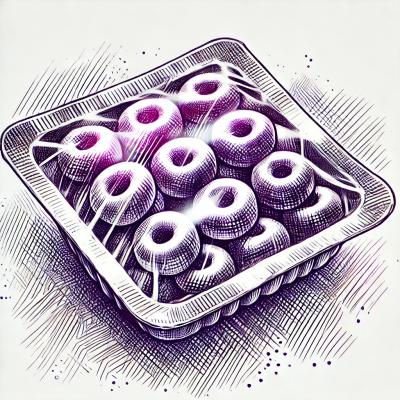
Security News
Understanding the Security Concerns of npm Shrinkwrap
Explore the security risks of using npm shrinkwrap, the potential for outdated dependencies, and best practices for mitigating these concerns in your projects.
fs-jetpack
Advanced tools
Package description
fs-jetpack is a file system utility library for Node.js that simplifies common file system operations. It provides a more intuitive and chainable API compared to the built-in 'fs' module, making it easier to perform tasks like reading, writing, copying, and deleting files and directories.
Reading Files
This feature allows you to read the contents of a file. The 'read' method takes the file path and encoding as arguments and returns the file content.
const jetpack = require('fs-jetpack');
const data = jetpack.read('path/to/file.txt', 'utf8');
console.log(data);
Writing Files
This feature allows you to write data to a file. The 'write' method takes the file path and the data to be written as arguments.
const jetpack = require('fs-jetpack');
jetpack.write('path/to/file.txt', 'Hello, world!');
Copying Files and Directories
This feature allows you to copy files or directories from one location to another. The 'copy' method takes the source path and the destination path as arguments.
const jetpack = require('fs-jetpack');
jetpack.copy('path/to/source', 'path/to/destination');
Deleting Files and Directories
This feature allows you to delete files or directories. The 'remove' method takes the path of the file or directory to be deleted as an argument.
const jetpack = require('fs-jetpack');
jetpack.remove('path/to/file_or_directory');
Inspecting Files and Directories
This feature allows you to get information about a file or directory. The 'inspect' method takes the path as an argument and returns an object with details like size, type, and modification time.
const jetpack = require('fs-jetpack');
const info = jetpack.inspect('path/to/file_or_directory');
console.log(info);
fs-extra is a popular file system utility library that extends the built-in 'fs' module with additional methods for common tasks like copying, moving, and removing files and directories. It is similar to fs-jetpack but offers a more extensive set of features and is widely used in the Node.js community.
node-fs is another file system utility library that provides additional methods for working with the file system. It offers functionalities like recursive directory creation and symbolic link management. While it is less feature-rich compared to fs-jetpack, it is still a useful alternative for basic file system operations.
graceful-fs is a drop-in replacement for the built-in 'fs' module that improves the handling of file system operations, especially under heavy load. It is designed to be more resilient and less prone to errors, making it a good choice for applications that require robust file system interactions.
Changelog
0.5.1 (2014-09-21)
cwd()
accepts many arguments as path parts.Readme
This is an attempt to make comprehensive, higher level API for node's fs library, which will be fun to use (see "Neat tricks fs-jetpack knows" as a starter).
npm install fs-jetpack
var jetpack = requite('fs-jetpack');
API has the same set of synchronous and asynchronous methods. All async methods are promise based (so no callbacks folks, promises instead).
Commonly used naming convention in node world is reversed in this library. Asynchronous methods are those with "Async" suffix, all methods without "Async" in the name are synchronous. Reason behind this is that it gives very nice look to blocking API, and promise based non-blocking code is verbose anyway, so one more word is not much of a difference. Also it just feels right to me. When you see "Async" word you are 100% sure this method is returning promise, and when you don't see it, you are 100% sure this method retuns immediately (and possibly blocks).
// Usage of blocking API
try {
jetpack.dir('foo');
} catch (err) {
// Something went wrong
}
// Usage of non-blocking API
jetpack.dirAsync('foo')
.then(function () {
// Done!
}, function (err) {
// Something went wrong
});
Methods:
also appendAsync(path, data, [options])
Appends given data to the end of file. If file (or any parent directory) doesn't exist, creates it (or them).
parameters:
path
the path to file.
data
data to append (could be String
or Buffer
).
options
(optional) Object
with possible fields:
mode
if the file doesn't exist yet, will be created with given mode. Value could be number (eg. 0700
) or string (eg. '700'
).returns:
Nothing.
also copyAsync(from, to, [options])
Copies given file or directory (with everything inside).
parameters:
from
path to location you want to copy.
to
path to destination location, where the copy should be placed.
options
(optional) additional options for customization. Is an Object
with possible fields:
overwrite
(default: false
) Whether to overwrite destination path if it exists. For directories, source directory is merged with destination directory, so files in destination which are not present in the source, will remain intact.only
(Array
of globs) will copy only items matching any of specified glob patterns (read more).allBut
(Array
of globs) will copy everything except items matching any of specified glob patterns (read more). If only
was also specified this field is ignored.returns:
Nothing.
examples:
// Copies a file (and replaces it if one already exists in "somewhere" direcotry)
jetpack.copy('file.txt', 'somwhere/file.txt', { overwrite: true });
// Copies only ".jpg" files from my_dir
jetpack.copy('my_dir', 'somewhere/my_dir', { only: ['*.jpg'] });
// Copies everything except "logs" directory inside my_dir
jetpack.copy('my_dir', 'somewhere/my_dir', { allBut: ['my_dir/logs'] });
Just an alias to vanilla fs.createReadStream.
Just an alias to vanilla fs.createWriteStream.
Returns Current Working Directory (CWD) for this instance of jetpack, or creates new jetpack object with given path as its internal CWD.
Note: fs-jetpack never changes value of process.cwd()
, the CWD we are talking about here is internal value inside every jetpack instance, and could be completely different than process.cwd()
.
parameters:
path
(optional) path to become new CWD. Could be absolute, or relative. If relative path given new CWD will be resolved basing on current CWD of this jetpack instance.
returns:
If path
not specified, returns CWD path of this jetpack object. For main instance of fs-jetpack it is always process.cwd()
.
If path
specified, returns new jetpack object (totally the same thing as main jetpack). The new object resolves paths according to its internal CWD, not the global one (process.cwd()
).
examples:
// Let's assume that process.cwd() outputs...
console.log(process.cwd()); // '/one/two/three'
// jetpack.cwd() will always return the same value as process.cwd()
console.log(jetpack.cwd()); // '/one/two/three'
// Now let's create new CWD context...
var jetParent = jetpack.cwd('..');
console.log(jetParent.cwd()); // '/one/two'
// ...and use this new context.
jetParent.dir('four'); // we just created directory '/one/two/four'
// One CWD context can be used to create next CWD context.
var jetParentParent = jetParent.cwd('..');
console.log(jetParentParent.cwd()); // '/one'
also dirAsync(path, [criteria])
Ensures that directory on given path meets given criteria. If any criterium is not met it will be after this call.
parameters:
path
path to directory to examine.
criteria
(optional) criteria to be met by the directory. Is an Object
with possible fields:
exists
(default: true
) whether directory should exist or not. If set to true
and path
contains many nested, nonexistent directories all of them will be created.empty
(default: false
) whether directory should be empty (no other files or directories inside). If set to true
and directory contains any files or subdirectories all of them will be deleted. If exists = false
this field is ignored.mode
ensures directory has specified mode. If not set and directory already exists, current mode will be preserved. Value could be number (eg. 0700
) or string (eg. '700'
).returns:
New CWD context with directory specified in path
as CWD.
Or undefined
if exists
was set to false
.
examples:
// Creates directory if doesn't exist
jetpack.dir('new_dir');
// Makes sure that directory does NOT exist
jetpack.dir('/my_stuff/some_dir', { exists: false });
// Makes sure directory mode is 0700 and that it's empty
jetpack.dir('empty_dir', { empty: true, mode: '700' });
// Because dir returns new CWD context pointing to just
// created directory you can create dir chains.
jetpack
.dir('main_dir') // creates 'main_dir'
.dir('sub_dir'); // creates 'main_dir/sub_dir'
also existsAsync(path)
Checks whether something exists on given path
. This method returns values more specyfic than true/false
to protect from errors like "I was expecting directory, but it was a file".
returns:
false
if path doesn't exist."dir"
if path is a directory."file"
if path is a file."other"
if none of the above.also fileAsync(path, [criteria])
Ensures that file meets given criteria. If any criterium is not met it will be after this call.
parameters:
path
path to file to examine.
criteria
(optional) criteria to be met by the file. Is an Object
with possible fields:
exists
(default: true
) whether file should exist or not.empty
(default: false
) whether file should be empty. If exists = false
this field is ignored.content
(String
, Buffer
, Object
or Array
) sets file content. If Object
or Array
given to this parameter the output will be JSON. If exists = false
, or empty = true
this field is ignored.jsonIndent
(defaults to 0) if writing JSON data this tells how many spaces should one indentation have.mode
ensures file has specified mode. If not set and file already exists, current mode will be preserved. Value could be number (eg. 0700
) or string (eg. '700'
).returns:
Jetpack object you called this method on (self).
examples:
// Creates file if doesn't exist
jetpack.file('something.txt');
// Ensures file does NOT exist (if exists will be deleted)
jetpack.file('not_something.txt', { exists: false });
// Creates file with mode '777' and content 'Hello World!'
jetpack.file('hello.txt', { mode: '777', content: 'Hello World!' });
also inspectAsync(path, [options])
Inspects given path (replacement for fs.stat).
parameters:
path
path to inspect.
options
(optional). Possible values:
checksum
if specified will return checksum of inspected file. Possible values are strings 'md5'
or 'sha1'
. If given path is directory this field is ignored and omited.returns:
null
if given path doens't exist.
Otherwise Object
of structure:
{
name: "my_dir",
type: "file", // possible values: "file", "dir"
size: 123, // size in bytes, this is returned only for files
md5: '900150983cd24fb0d6963f7d28e17f72' // (if checksum option was specified)
}
also inspectTreeAsync(path, [options])
Calls inspect recursively on given path so it creates tree of all directories and sub-directories inside it.
parameters:
path
the path to inspect.
options
(optional). Possible values:
checksum
if specified will also calculate checksum of every item in the tree. Possible values are strings 'md5'
or 'sha1'
. Checksums for directories are calculated as checksum of all children' checksums plus their filenames (see example below).returns:
null
if given path doesn't exist.
Otherwise tree of inspect objects like:
{
name: 'my_dir',
type: 'dir',
size: 123, // this is combined size of all items in this directory
md5: '11c68d9ad988ff4d98768193ab66a646',
// checksum of this directory was calculated as:
// md5(child[0].name + child[0].md5 + child[1].name + child[1].md5)
children: [
{
name: 'empty',
type: 'dir',
size: 0, // the directory is empty
md5: null, // can't calculate checksum of empty directory
children: []
},{
name: 'file.txt',
type: 'file',
size: 123,
md5: '900150983cd24fb0d6963f7d28e17f72'
}
]
}
also listAsync(path, [useInspect])
Lists the contents of directory.
parameters:
path
path to directory you would like to list.
useInspect
(optional) the type of data this call should return. Possible values:
false
(default) returns just a list of filenames (the same as fs.readdir()
)true
performs inspect on every item in directory, and returns array of those objectsobject
if object has been passed to this parameter, it is treated as options
parameter for inspect method, and will alter returned inspect objectsreturns:
Array
of strings or objects depending on call properies. Or null
if given path doesn't exist.
also moveAsync(from, to)
Moves given path to new location.
parameters:
from
path to directory or file you want to move.
to
path where the thing should be moved.
returns:
Nothing.
Returns path resolved to internal CWD of this jetpack object.
parameters:
parts
strings to join and resolve as path (as many as you like).
returns:
Resolved path as string.
examples:
jetpack.cwd(); // if it returns '/one/two'
jetpack.path(); // this will return the same '/one/two'
jetpack.path('three'); // this will return '/one/two/three'
jetpack.path('..', 'four'); // this will return '/one/four'
also readAsync(path, [returnAs], [options])
Reads content of file. If file on given path doesn't exist returns null
instead of throwing ENOENT
error.
parameters:
path
path to file.
returnAs
(optional) how the content of file should be returned. Is a string with possible values:
'utf8'
(default) content will be returned as UTF-8 String.'buf'
content will be returned as Buffer.'json'
content will be returned as parsed JSON object.'jsonWithDates'
content will be returned as parsed JSON object, and date strings in ISO format will be automatically turned into Date objects.options
(optional) is an object with possible fields:
safe
if set to true
the file will be read in "safe mode".returns:
File content in specified format, or null
if file doesn't exist.
also removeAsync(path, [options])
Deletes given path, no matter what it is (file or directory).
parameters:
path
path to file or directory you want to remove.
options
(optional) additional conditions to removal process. Is an object with possible fields:
only
(Array
of globs) will delete only items matching any of specified glob patterns (read more on that).allBut
(Array
of globs) will delete everything except items matching any of specified glob patterns (read more on that). If only
was also specified this field is ignored.returns:
Nothing.
examples:
// Deletes file
jetpack.remove('my_work/notes.txt');
// Deletes directory "important_stuff" and everything inside
jetpack.remove('my_work/important_stuff');
// Will delete any ".log" file, and any folder or file named "temp" inside "my_app",
// but will leave all other files intact.
jetpack.remove('my_app', { only: [ '*.log', 'temp' ] });
// Will delete everything inside "my_app" directory,
// but will leave directory or file "my_app/user_data" intact.
jetpack.remove('my_app', { allBut: [ 'my_app/user_data' ] });
also renameAsync(path, newName)
Renames given file or directory.
parameters:
path
path to thing you want to change name.
newName
new name for this thing (not full path, just a name).
returns:
Nothing.
also writeAsync(path, data, [options])
Writes data to file.
parameters:
path
path to file.
content
data to be written. This could be String
, Buffer
, Object
or Array
(if last two used, the data will be outputed into file as JSON).
options
(optional) Object
with possible fields:
jsonIndent
(defaults to 0) if writing JSON data this tells how many spaces should one indentation have.safe
if set to true
the file will be written in "safe mode".returns:
Nothing.
So you can create many jetpack objects and work on directories in a little more object-oriented fashion.
var src = jetpack.cwd('path/to/source');
var dest = jetpack.cwd('path/to/destination');
src.copy('foo.txt', dest.path('bar.txt'));
You can create whole tree of directories and files in declarative style.
// Synchronous style
jetpack
.dir('foo')
.file('foo.txt', { content: 'Hello...' })
.file('bar.txt', { content: '...world!' })
.cwd('..')
.dir('bar')
.file('foo.txt', { content: 'Wazup?' });
// Asynchronous style (unfortunately not that pretty)
jetpack
.dirAsync('foo')
.then(function (dir) {
return dir.fileAsync('foo.txt', { content: 'Hello...' });
})
.then(function (dir) {
return dir.fileAsync('bar.txt', { content: '...world!' });
.then(function (dir) {
return dir.cwd('..').dirAsync('bar');
})
.then(function (dir) {
dir.fileAsync('foo.txt', { content: 'Wazup?' });
});
"ENOENT, no such file or directory" is the most annoying error when working with file system, and fs-jetpack does 2 things to save you the hassle:
null
is returned instead of throwing.Copy and remove have option for blacklisting and whitelisting things inside directory which will be affected by the operation. For instance:
// Let's assume we have folder structure:
// foo
// |- a.jpg
// |- b.pdf
// |- c.txt
// |- bar
// |- a.pdf
jetpack.copy('foo', 'foo1', { allBut: ['*.pdf'] });
// Will give us folder:
// foo1
// |- a.jpg
// |- c.txt
// |- bar
jetpack.copy('foo', 'foo2', { only: ['*.pdf'] });
// Will give us folder:
// foo2
// |- b.pdf
// |- bar
// |- a.pdf
It is not fully safe to just overwrite existing file with new content. If process will crash during the operation, you are basically srewed. The old file content is lost, because you overwritten it. And the new file is empty or written only partially. Fs-jetpack has built-in "safe mode", which helps you get rid of this issue. Under the hood it works as follows...
Let's assume there's already file.txt
with content Hello world!
on disk, and we want to update it to Hello universe!
.
jetpack.write('file.txt', { safe: true, content: 'Hello universe!' });
Above line will perform tasks as follows:
Hello universe!
to file.txt.__new__
(so we didn't owerwrite the original file).file.txt
(with Hello world!
) to file.txt.__bak__
, so it can serve as a backup.file.txt.__new__
to file.txt
, where we wanted it to be on the first place.file.txt.__bak__
, because it is no longer needed.For this to work, read operation have to be aware of the backup file.
jetpack.read('file.txt', { safe: true });
Above read will do:
file.txt
file.txt.__bak__
.The whole process is performed automatically for you by simply adding safe: true
to call options of write and read methods.
FAQs
Better file system API
The npm package fs-jetpack receives a total of 465,839 weekly downloads. As such, fs-jetpack popularity was classified as popular.
We found that fs-jetpack demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Explore the security risks of using npm shrinkwrap, the potential for outdated dependencies, and best practices for mitigating these concerns in your projects.
Security News
Node.js is taking steps towards removing Corepack from its distribution, aiming for changes in the next major release.
Security News
OpenSSF has released a guide to help package repositories adopt Trusted Publishers, which enhances security by using short-lived identity tokens for authentication, reducing the risks associated with long-lived secrets.