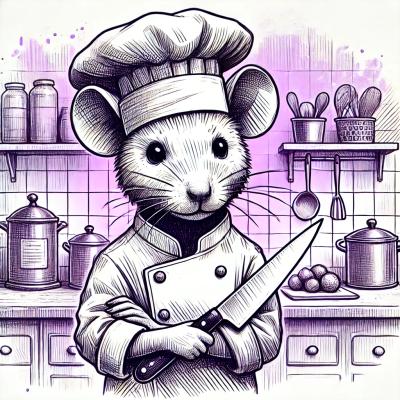
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
function-composer
Advanced tools
Compose functions with multiple type signatures.
Features:
Supported environments: node.js, Chrome, Firefox, Safari, Opera, IE9+.
Install via npm:
npm install function-composer
Example usage:
var compose = require('function-composer');
// compose a new function
var fn = compose({
'number': function (a) {
return 'a is a number';
},
'number, boolean': function (a, b) {
return 'a is a number, b is a boolean';
},
'number, number': function (a, b) {
return 'a is a number, b is a number';
}
});
// use the function
console.log(fn(2, true)); // outputs 'a is a number, b is a boolean'
console.log(fn(2)); // outputs 'a is a number'
// calling the function with a non-supported type signature will throw an error
try {
fn('hello world');
}
catch (err) {
console.log(err.toString()); // outputs: 'TypeError: Wrong function signature'
}
Type checking input arguments adds some overhead to a function. For very small
functions this overhead can be larger than the function execution itself is,
but for any non-trivial function the overhead is typically small to neglectable.
You need to keep in mind though that you probably would have to do the type
checking done by function-composer
anyway.
A function is constructed as:
compose(signatures: Object.<string, function>) : function
compose(name: string, signatures: Object.<string, function>) : function
compose.types: Object
A map with the object types as key and a type checking test as value.
Custom types can be added like:
function Person(...) {
...
}
compose.types['Person'] = function (x) {
return x instanceof Person;
};
compose.conversions: Array
An Array with built-in conversions. Empty by default. Can be used for example
to defined conversions from boolean
to number
. For example:
compose.conversions.push({
from: 'boolean',
to: 'number',
convert: function (x) {
return +x;
});
function-composer has the following built-in types:
null
boolean
number
string
function
Array
Date
RegExp
Object
*
(anytype)The functions generated with compose({...})
have:
toString()
function which returns well readable code, giving insight in
what the function exactly does.signatures
, which holds a map with the (normalized)
signatures as key and the original sub-functions as value.'*, boolean'
'string, ...'
'number?, array'
number | string, number'
'"linear" | "cubic"'
.'{name: string, age: number}'
'Object.<string, Person>'
'Array.<Person>'
To test the library, run:
npm test
To generate the minified version of the library, run:
npm run minify
2014-11-05, version 0.3.0
*
).FAQs
Compose functions with multiple type signatures
The npm package function-composer receives a total of 0 weekly downloads. As such, function-composer popularity was classified as not popular.
We found that function-composer demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.