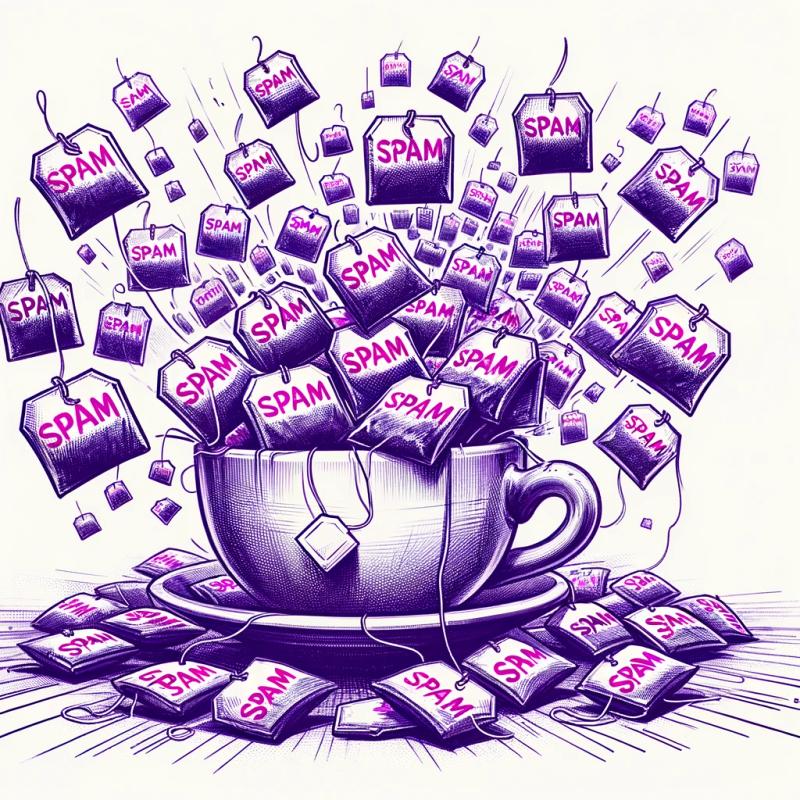
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
get-tsconfig
Advanced tools
Readme
Find and parse tsconfig.json
files.
tsconfig.json
extends
tsconfig.json
7 kB
Minified + Gzippednpm install get-tsconfig
For TypeScript related tooling to correctly parse tsconfig.json
file without depending on TypeScript.
Searches for a tsconfig.json
file and parses it. Returns null
if a config file cannot be found, or an object containing the path and parsed TSConfig object if found.
Returns:
type TsconfigResult = {
/**
* The path to the tsconfig.json file
*/
path: string
/**
* The resolved tsconfig.json file
*/
config: TsConfigJsonResolved
}
Type: string
Default: process.cwd()
Accepts a path to a file or directory to search up for a tsconfig.json
file.
Type: string
Default: tsconfig.json
The file name of the TypeScript config file.
Type: Map<string, any>
Default: new Map()
Optional cache for fs operations.
import { getTsconfig } from 'get-tsconfig'
// Searches for tsconfig.json starting in the current directory
console.log(getTsconfig())
// Find tsconfig.json from a TypeScript file path
console.log(getTsconfig('./path/to/index.ts'))
// Find tsconfig.json from a directory file path
console.log(getTsconfig('./path/to/directory'))
// Explicitly pass in tsconfig.json path
console.log(getTsconfig('./path/to/tsconfig.json'))
// Search for jsconfig.json - https://code.visualstudio.com/docs/languages/jsconfig
console.log(getTsconfig('.', 'jsconfig.json'))
The tsconfig.json
parser used internally by getTsconfig
. Returns the parsed tsconfig as TsConfigJsonResolved
.
Type: string
Required path to the tsconfig file.
Type: Map<string, any>
Default: new Map()
Optional cache for fs operations.
import { parseTsconfig } from 'get-tsconfig'
// Must pass in a path to an existing tsconfig.json file
console.log(parseTsconfig('./path/to/tsconfig.custom.json'))
Given a tsconfig.json
file, it returns a file-matcher function that determines whether it should apply to a file path.
type FileMatcher = (filePath: string) => TsconfigResult['config'] | undefined
Type: TsconfigResult
Pass in the return value from getTsconfig
, or a TsconfigResult
object.
Type: boolean
By default, it uses is-fs-case-sensitive
to detect whether the file-system is case-sensitive.
Pass in true
to make it case-sensitive.
For example, if it's called with a tsconfig.json
file that has include
/exclude
/files
defined, the file-matcher will return the config for files that match include
/files
, and return undefined
for files that don't match or match exclude
.
const tsconfig = getTsconfig()
const fileMatcher = tsconfig && createFileMatcher(tsconfig)
/*
* Returns tsconfig.json if it matches the file,
* undefined if not
*/
const configForFile = fileMatcher?.('/path/to/file.ts')
const distCode = compileTypescript({
code: sourceCode,
tsconfig: configForFile
})
Given a tsconfig with compilerOptions.paths
defined, it returns a matcher function.
The matcher function accepts an import specifier (the path to resolve), checks it against compilerOptions.paths
, and returns an array of possible paths to check:
function pathsMatcher(specifier: string): string[]
This function only returns possible paths and doesn't actually do any resolution. This helps increase compatibility wtih file/build systems which usually have their own resolvers.
import { getTsconfig, createPathsMatcher } from 'get-tsconfig'
const tsconfig = getTsconfig()
const pathsMatcher = createPathsMatcher(tsconfig)
const exampleResolver = (request: string) => {
if (pathsMatcher) {
const tryPaths = pathsMatcher(request)
// Check if paths in `tryPaths` exist
}
}
tsconfig.json
?This package is a re-implementation of TypeScript's tsconfig.json
parser.
However, if you already have TypeScript as a dependency, you can simply use it's API:
import {
sys as tsSys,
findConfigFile,
readConfigFile,
parseJsonConfigFileContent
} from 'typescript'
// Find tsconfig.json file
const tsconfigPath = findConfigFile(process.cwd(), tsSys.fileExists, 'tsconfig.json')
// Read tsconfig.json file
const tsconfigFile = readConfigFile(tsconfigPath, tsSys.readFile)
// Resolve extends
const parsedTsconfig = parseJsonConfigFileContent(
tsconfigFile.config,
tsSys,
path.dirname(tsconfigPath)
)
FAQs
Find and parse the tsconfig.json file from a directory path
The npm package get-tsconfig receives a total of 7,375,376 weekly downloads. As such, get-tsconfig popularity was classified as popular.
We found that get-tsconfig demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.